Navigator operation requested with a context that does not include a Navigator
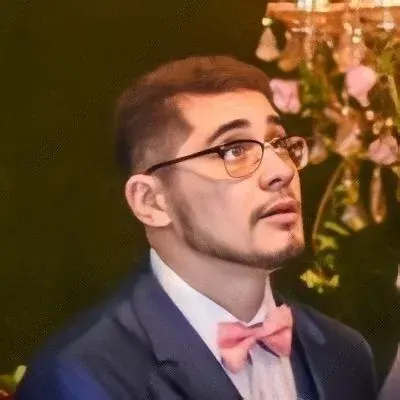
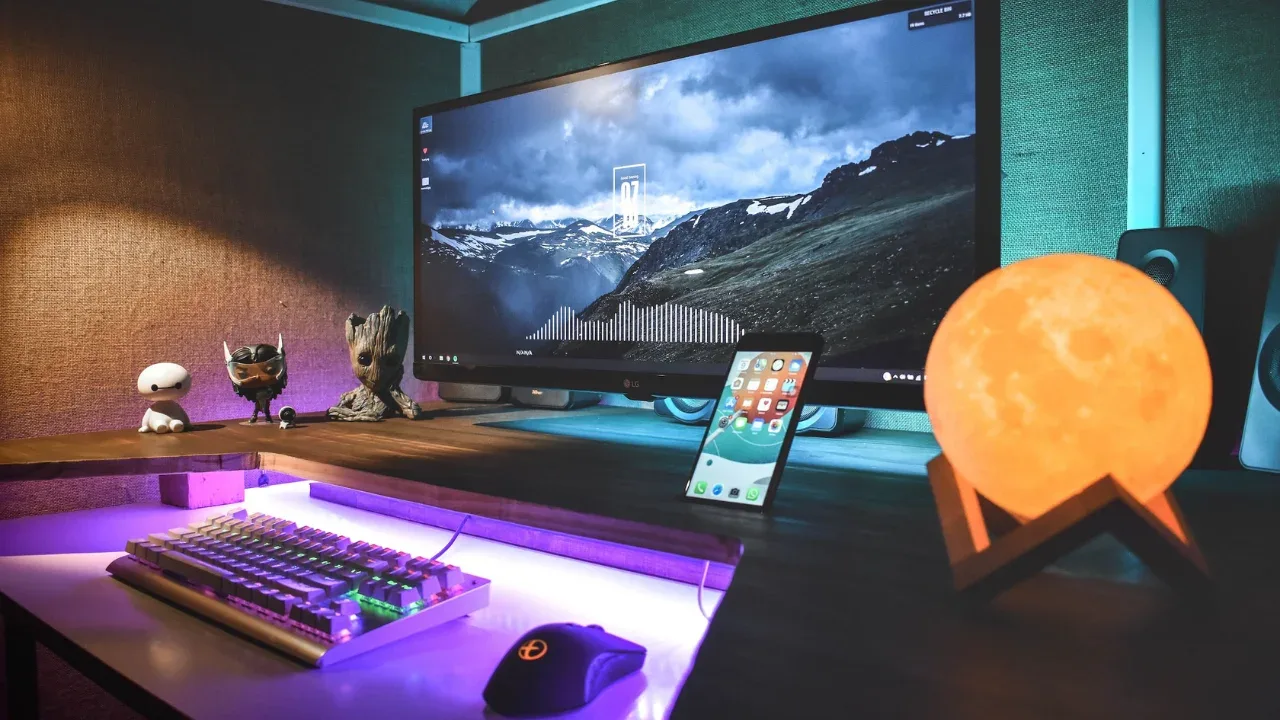
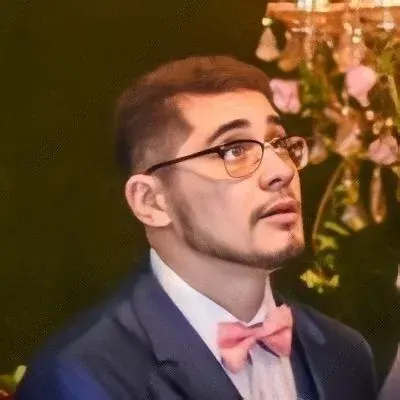
š Title: "Troubleshooting "Navigator operation requested with a context that does not include a Navigator" Error"
š Hey there, techies! It's time to dive into another common error that Flutter developers encounter - the dreaded "Navigator operation requested with a context that does not include a Navigator" error. š«š
Let's take a closer look at the code provided by the reader:
onTap: () { Navigator.of(context).pushNamed('/settings'); },
The error is thrown here because the context
used to call the Navigator.of
method does not include a Navigator
. But don't worry, I've got some solutions that will help you navigate through this issue with ease. š
Solution 1: Check the App's Routes Configuration š§
The reader mentioned that they have set up a route in their app, as shown below:
routes: <String, WidgetBuilder>{
'/settings': (BuildContext context) => new SettingsPage(),
},
To resolve the error, ensure that you have added the routes properly in the MaterialApp
or CupertinoApp
widget. These routes are used by the Navigator
to locate the destination screen.
Solution 2: Ensure a Valid Navigation Context š§©
The context
used in the Navigator.of(context)
call should be associated with the current widget tree, where the Navigator
is properly configured.
In the provided code, the onTap
callback is defined within a build method of a stateless widget. Ensure that this widget is wrapped within a Navigator
or is part of a widget hierarchy that includes a Navigator
.
Here's an example of how you can wrap your widget tree with a Navigator
:
class MyApp extends StatelessWidget {
@override
Widget build(BuildContext context) {
return MaterialApp(
// Add your routes here if necessary
home: Navigator(
onGenerateRoute: (RouteSettings settings) {
// Handle routes here
},
child: YourWidget(),
),
);
}
}
Solution 3: Verify the Widget Inheritance šļø
In the provided code, the SettingsPage
class extends Navigator
, which is not the recommended way to create a page. Instead, SettingsPage
should extend a widget like StatefulWidget
or StatelessWidget
.
Ensure that you import the necessary packages and correct the inheritance of the SettingsPage
class.
class SettingsPage extends StatelessWidget {
//...
}
š That's it! We've covered some common solutions to help you fix the "Navigator operation requested with a context that does not include a Navigator" error. Just follow these steps, and you'll be navigating your Flutter app like a pro in no time! š
If you still have any doubts or want to share your experience with this error, leave a comment below! Let's help each other out in our coding journeys. š
Keep coding and Flutter on! š