How to use conditional statement within child attribute of a Flutter Widget (Center Widget)
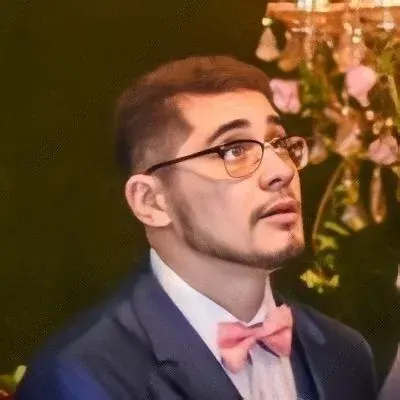
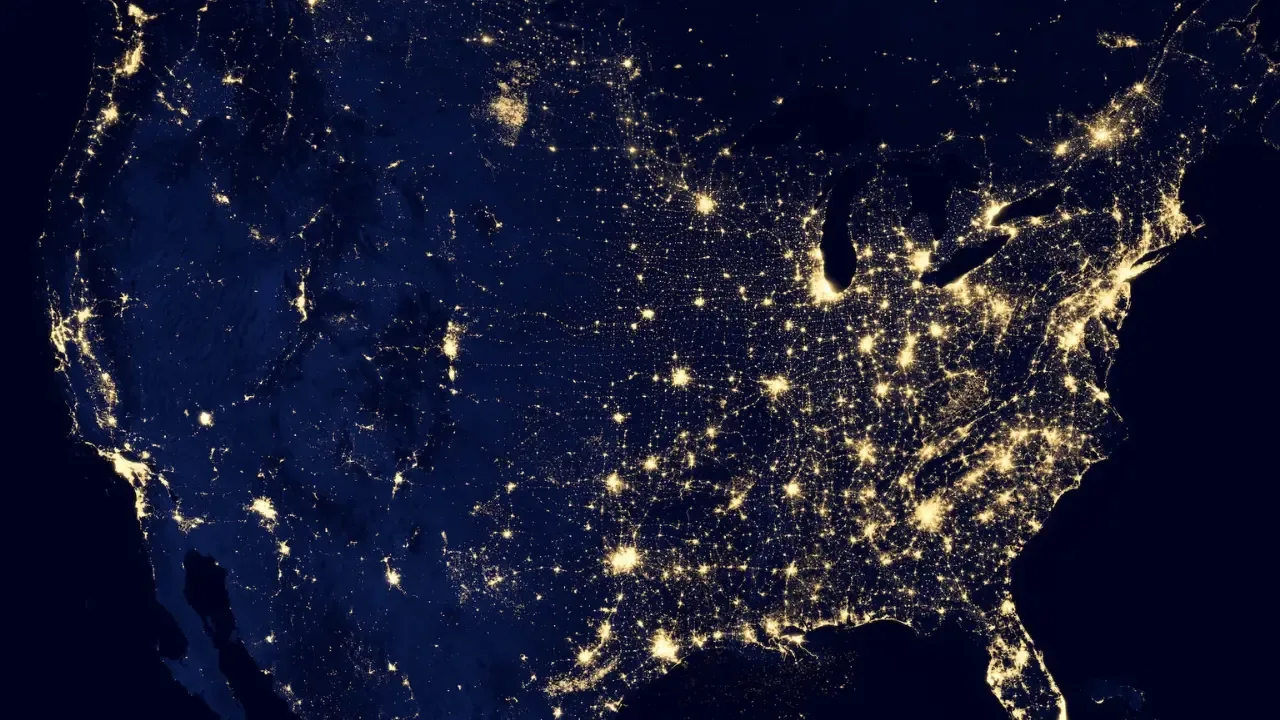
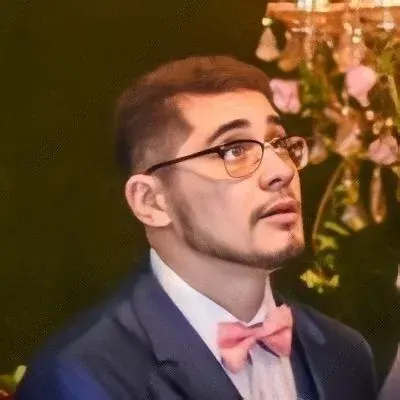
📝 Flutter Widget: How to Use Conditional Statements within the Child Attribute of a Center Widget
Are you trying to use a conditional statement within the child attribute of a Flutter Widget? Do you find yourself encountering a dead code warning or struggling to make it work properly? Don't worry, you're not alone! In this blog post, we'll dive into this common issue and provide you with easy solutions to overcome it. So let's get started! 🚀
🧐 Understanding the Problem
Let's begin by taking a closer look at the code snippet provided. In this case, the user wanted to conditionally render a widget within the child attribute of a Center
widget. Their initial attempt using the ternary operator (condition ? true : false
) was successful. However, when they tried using an if/else statement or a switch case statement, a dead code warning was triggered, preventing the code from running.
🔗 The Cause of Dead Code Warnings
The reason for this dead code warning is that Flutter's build method expects a single expression to be returned. In the case of using if/else or switch statements, the compiler is unable to determine if both branches (if and else) will be executed. As a result, it assumes that one of them will be unreachable, hence the warning.
💡 Easy Solutions
Fortunately, there are a few easy solutions to overcome this issue and use conditional statements within the child attribute of a Flutter Widget. Let's explore them one by one:
1️⃣ Solution 1: Using the Ternary Operator
As mentioned earlier, the ternary operator is a valid way to achieve conditional rendering within the child attribute of a Flutter Widget. Here's an example:
Center(
child: condition ? Container() : Container()
)
2️⃣ Solution 2: Using a Builder Widget
Another approach is to use a Builder
widget, which provides a separate build context for conditional rendering. It allows you to write if/else or switch statements without triggering any dead code warnings. Here's how you can implement it:
Center(
child: Builder(
builder: (BuildContext context) {
if (condition) {
return Container();
} else {
return Container();
}
},
),
)
3️⃣ Solution 3: Using a Function
You can also extract your conditional logic into a separate function and call it within the child attribute. This ensures that Flutter's build method receives a single expression. Here's an example:
Widget renderContainer() {
if (condition) {
return Container();
} else {
return Container();
}
}
Center(
child: renderContainer(),
)
🔔 Engage with Us!
We hope these solutions helped you overcome any challenges you were facing when using conditional statements within the child attribute of a Center
widget. If you have any additional questions or need further assistance, feel free to leave a comment below. We're here to help! 😊
💥 Share the Knowledge!
Found this blog post helpful? Share it with your fellow Flutter enthusiasts and help them tackle this common issue too. Together, we can make the Flutter community even stronger! 🙌