How to underline text in flutter
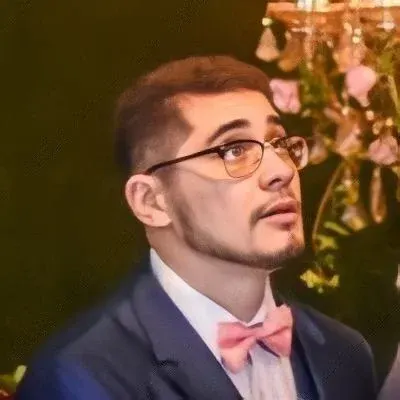

How to Underline Text in Flutter: A Complete Guide 🎯
So you want to underline text in Flutter, specifically inside the Text
widget? You're in the right place! Whether you're a Flutter newbie or a seasoned developer, it's always good to have a clear understanding of how to achieve this simple yet crucial task. Let's dive in and explore the different methods to underline text in Flutter.
The Underline Text Dilemma ❓
You're probably wondering why the TextStyle
's fontStyle
property doesn't seem to have an underline option. Well, you're not alone! Flutter's TextStyle
doesn't provide a direct way to underline text. However, fear not! There are a couple of workarounds that you can adopt to achieve the desired effect. Let's explore them one by one.
Workaround #1: Using the Container
Widget 📦
One way to underline text in Flutter is by utilizing the Container
widget. Here's how you can implement it:
Container(
decoration: BoxDecoration(
border: Border(
bottom: BorderSide(
color: Colors.black, // Specify your desired underline color
width: 1.0, // Specify the underline thickness
),
),
),
child: Text(
"Your Underlined Text",
style: TextStyle(
fontSize: 16.0, // Specify the desired font size
color: Colors.black, // Specify the desired text color
// Any other desired text style properties
),
),
)
By using the Container
widget and its decoration
property, we create a border with a specified color and thickness at the bottom of the widget. This visually simulates underlining the text.
Workaround #2: Using the RichText
Widget 🌈
Another approach to underline text in Flutter is by leveraging the RichText
widget. Here's an example:
RichText(
text: TextSpan(
text: "Your Underlined Text",
style: TextStyle(
fontSize: 16.0, // Specify the desired font size
color: Colors.black, // Specify the desired text color
// Any other desired text style properties
),
decorations: [
TextDecoration.underline,
].map((decoration) {
return TextDecorationStyle.solid;
}).toList(),
),
)
Here, we use the RichText
widget along with TextSpan
to apply text decorations. By setting the decorations
property to [TextDecoration.underline]
, we achieve the underlined effect. Adjust the font size, color, and any other desired text style properties to suit your needs.
Choose Your Underlining Method ✏️
Now that you've seen both workarounds, it's up to you to choose the best approach for your Flutter project. Both methods provide the desired underlining effect, but depending on your specific needs and the context in which you're applying the underline, one may be more suitable than the other.
Get Underlining! 📝
Now that you know how to underline text in Flutter, it's time to implement it in your own projects! Play around with different font styles, colors, and underline thicknesses to achieve the perfect visual effect.
If you have any questions or other Flutter-related topics you'd like us to cover, let us know in the comments below. Happy coding! 💻🚀
🌟 Download the Underline Flutter Example Project 🌟
To help you further, we've prepared a sample Flutter project showcasing the different methods for underlining text. Download it now and get a head start on implementing underlined text in your own applications.
Take Your Tech Career to the Next Level
Our application tracking tool helps you manage your job search effectively. Stay organized, track your progress, and land your dream tech job faster.
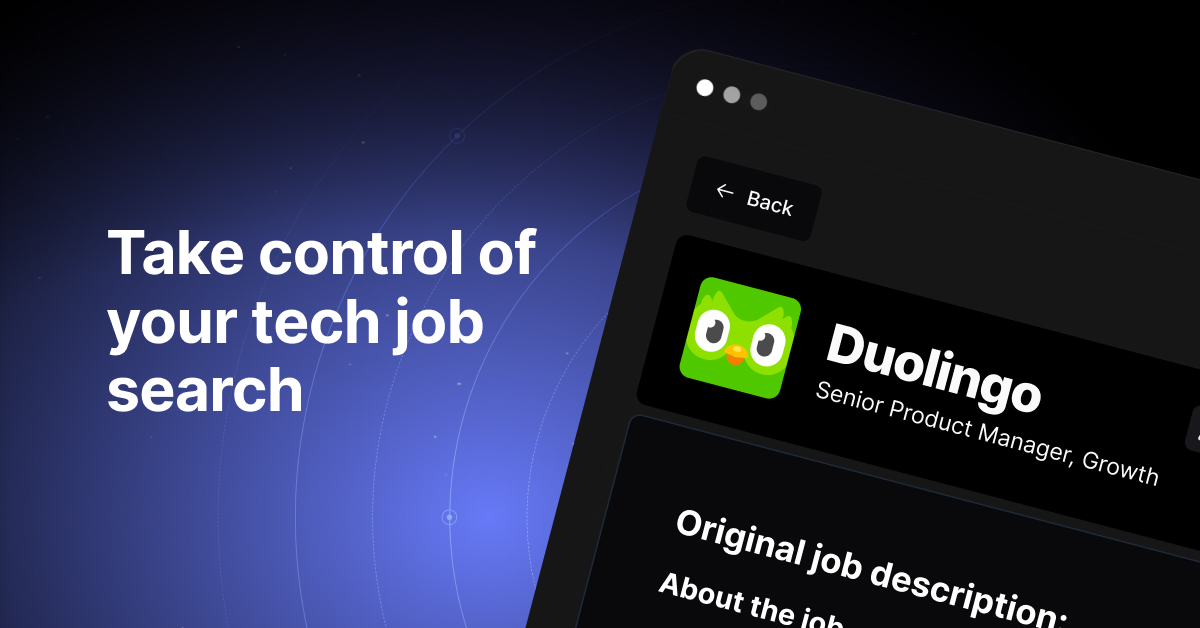