How to show/hide widgets programmatically in Flutter
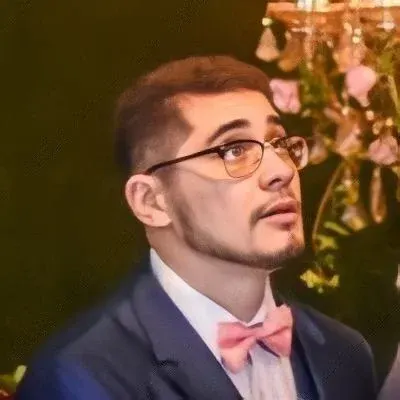
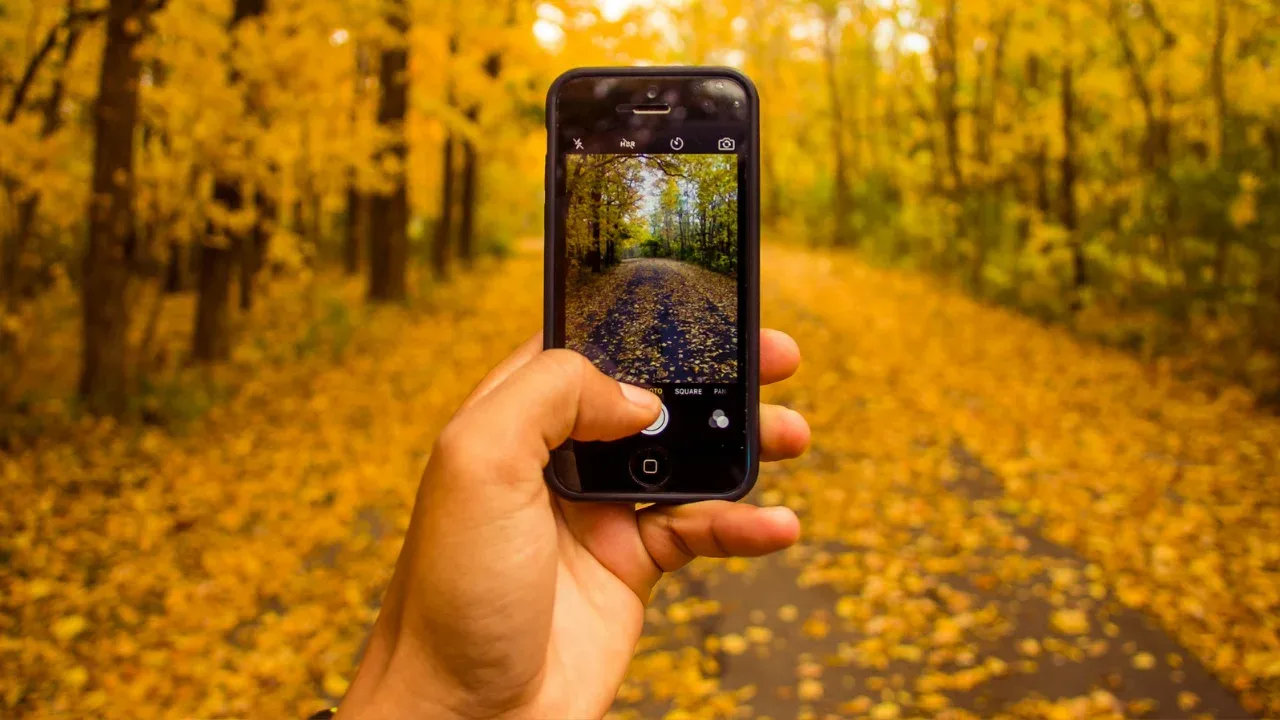
How to Show/Hide Widgets Programmatically in Flutter 😎✨
Have you ever wondered how to dynamically show or hide widgets in your Flutter application? In Android, we have the setVisibility()
method to modify the visibility of a View
object easily. But what about Flutter? 🤔
The Equivalent Visibility Options in Flutter 📱
Just like in Android, Flutter provides us with three options for setting the visibility of widgets:
Visible 🌟: Renders the widget visible inside the layout. It's the equivalent of
View.VISIBLE
in Android.Invisible 🕶️: Hides the widget, but leaves a gap where it would be if it were visible. This option is similar to
View.INVISIBLE
in Android.Gone 🚫: Hides the widget completely and removes it from the layout. It's as if the widget's
height
andwidth
were0dp
. You can relate this toView.GONE
in Android.
The Solution and Example Code 💡📝
To show or hide widgets programmatically in Flutter, we can make use of the Visibility
widget, which is perfect for this task. The Visibility
widget allows us to toggle the visibility of its child widget based on a bool
value.
Let's say we have a simple scenario where we want to toggle the visibility of a Container
widget based on a button tap. Here's how we can achieve that:
bool _isVisible = true;
// ...
Visibility(
visible: _isVisible,
child: Container(
// Your container properties and child widgets
),
),
// Button to toggle visibility
ElevatedButton(
onPressed: () {
setState(() {
_isVisible = !_isVisible;
});
},
child: Text(_isVisible ? 'Hide Container' : 'Show Container'),
)
In the code snippet above, we have a _isVisible
boolean variable that determines the visibility of the Container
widget. We wrap the Container
widget with Visibility
and set the visible
property to _isVisible
. Depending on the value of _isVisible
, the Container
widget will be shown or hidden.
The button below the Visibility
widget allows us to toggle the visibility by changing the value of _isVisible
on button press.
Conclusion and Your Next Step 🚀📣
Now that you know how to show/hide widgets programmatically in Flutter, you can start adding dynamic behavior to your apps with ease. Experiment with different scenarios and adapt this solution to suit your specific needs.
Remember, using the Visibility
widget and a boolean variable gives you a powerful way to control the visibility of widgets in Flutter.
So go ahead, give it a try! Share your thoughts and experiences with us in the comments below. Let's level up our Flutter game together! 💪💙
🌟🚀 Happy coding, Flutteristas! 🚀🌟
Take Your Tech Career to the Next Level
Our application tracking tool helps you manage your job search effectively. Stay organized, track your progress, and land your dream tech job faster.
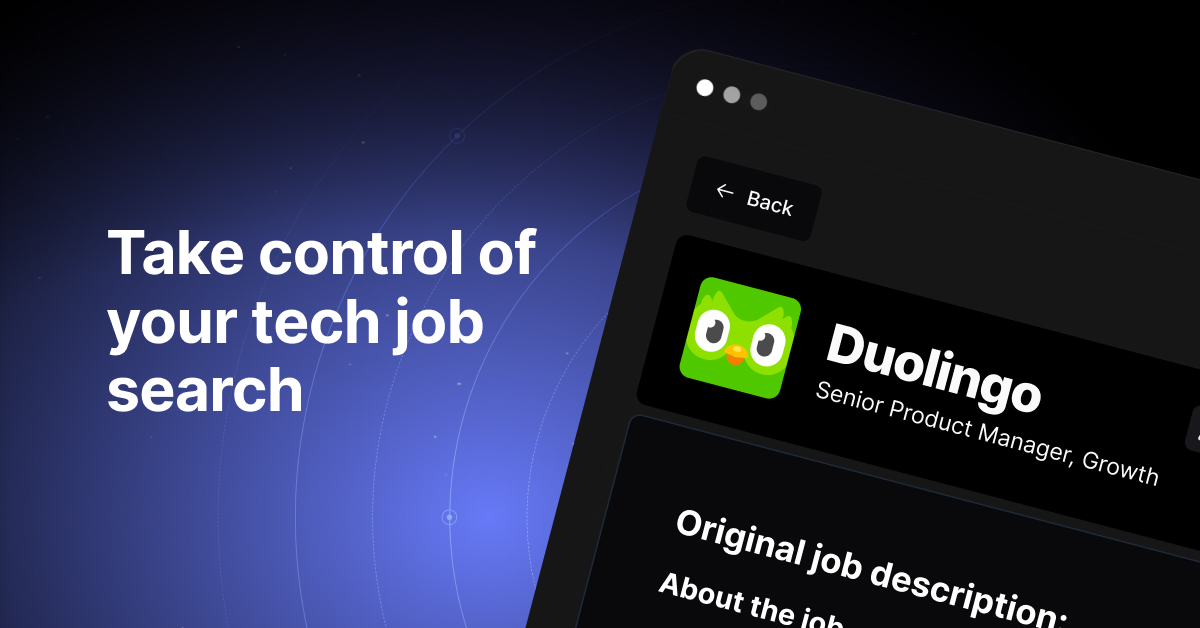