How to save to local storage using Flutter?
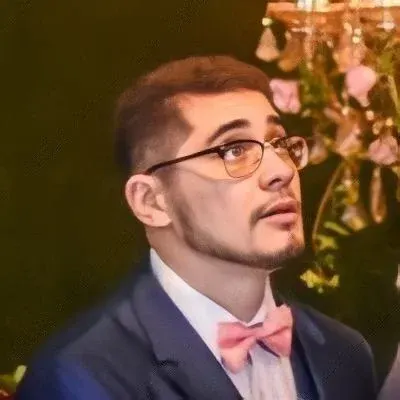
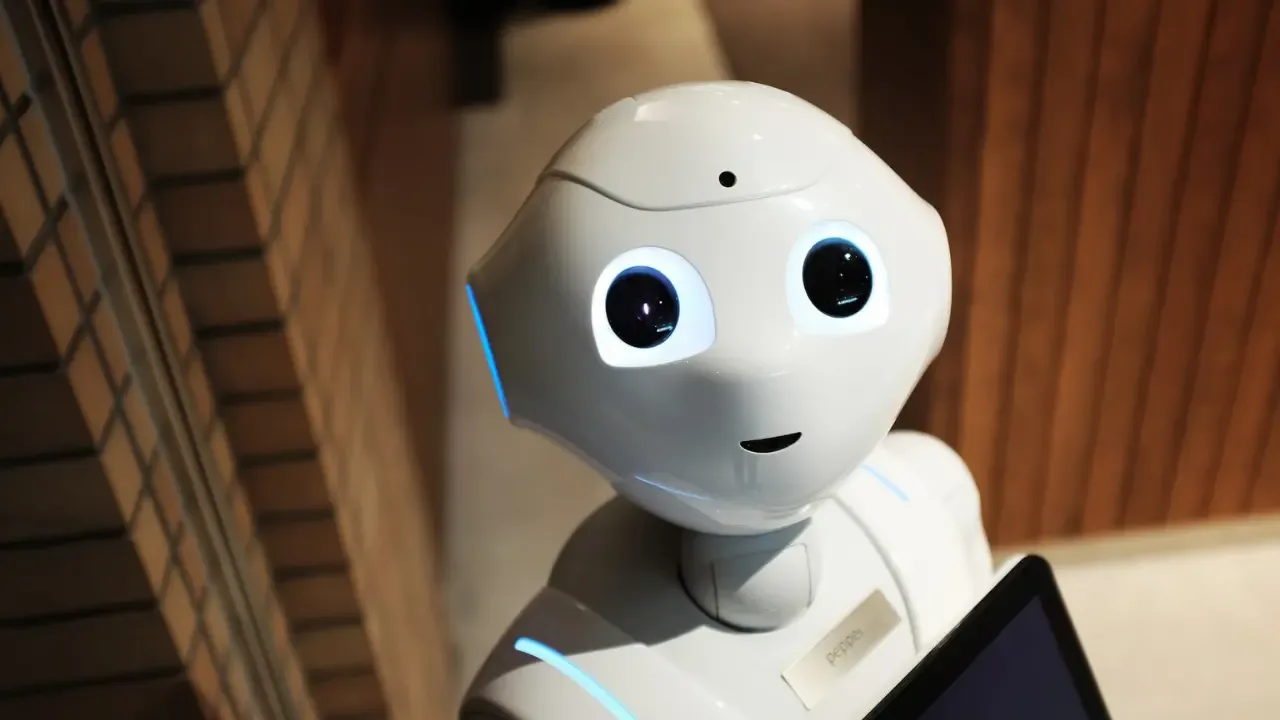
Saving Data to Local Storage in Flutter: A Beginner's Guide ππΎπ±
So, you're building a Flutter app and you want to save data to the local storage? π€ Don't worry, we got you covered! In this guide, we'll explore how to save data to local storage using Flutter, without the need for device-specific code or external dependencies. Let's dive right in! πͺπ
Understanding the Options
In Android, you have various options like SharedPreferences
, SQLite databases, or writing files to the device. But what about Flutter? π€·ββοΈ
When it comes to Flutter, you can save data locally using the following approaches:
Shared Preferences: This method is similar to Android's
SharedPreferences
and allows you to store key-value pairs persistently.Local Database (SQLite): Flutter provides a built-in package called
sqflite
that enables you to interact with a local SQLite database. This is useful for storing more complex data structures.File System: If you simply need to read and write files, Flutter also provides APIs to interact with the file system.
Saving to Local Storage Using Shared Preferences
Saving data to local storage using the shared preferences package is straightforward and doesn't require much code. Here's how you can do it:
Add the
shared_preferences
package to yourpubspec.yaml
file:
dependencies:
shared_preferences: ^2.0.13
Import the package in your Dart file:
import 'package:shared_preferences/shared_preferences.dart';
Saving data to shared preferences:
Future<void> saveDataToPrefs(String key, String value) async {
final prefs = await SharedPreferences.getInstance();
await prefs.setString(key, value);
print('Data saved successfully!');
}
Retrieving data from shared preferences:
Future<String?> getDataFromPrefs(String key) async {
final prefs = await SharedPreferences.getInstance();
return prefs.getString(key);
}
Remember to replace String
with the appropriate data type based on your requirements.
Saving to Local Database (SQLite)
If you're dealing with more complex data structures or want the querying capabilities of a database, you can use the sqflite
package for interacting with a local SQLite database in Flutter.
Add the
sqflite
package to yourpubspec.yaml
file:
dependencies:
sqflite: ^2.0.0+4
Import the package in your Dart file:
import 'package:sqflite/sqflite.dart';
import 'package:path/path.dart';
Creating a table and saving data:
Future<void> saveDataToDb(String name) async {
final database = openDatabase(
join(await getDatabasesPath(), 'my_database.db'),
onCreate: (db, version) {
return db.execute(
'CREATE TABLE my_table(id INTEGER PRIMARY KEY, name TEXT)',
);
},
version: 1,
);
final db = await database;
await db.transaction((txn) async {
await txn.rawInsert(
'INSERT INTO my_table(name) VALUES(?)',
[name],
);
print('Data saved successfully!');
});
}
Retrieving data from the database:
Future<List<Map<String, dynamic>>> getDataFromDb() async {
final database = openDatabase(
join(await getDatabasesPath(), 'my_database.db'),
);
final db = await database;
final List<Map<String, dynamic>> maps = await db.query('my_table');
return maps;
}
Make sure to customize the table name and column names according to your needs.
Saving to File System
If all you need is basic file read and write operations, Flutter also supports file system interactions. Here's an example:
Writing to a file:
Future<void> writeToFile(String text) async {
final file = await File('path_to_file.txt').create();
await file.writeAsString(text);
print('Data written to file!');
}
Reading from a file:
Future<String> readFromFile() async {
final file = File('path_to_file.txt');
if (await file.exists()) {
final contents = await file.readAsString();
return contents;
} else {
return 'File not found!';
}
}
Remember to replace 'path_to_file.txt'
with the actual file path or name.
Conclusion
Saving data to local storage in Flutter doesn't have to be complicated! Whether you prefer shared preferences, local databases, or simple file system interactions, Flutter offers numerous options to suit your needs. π¦
Now that you know these techniques, go ahead and build amazing Flutter apps with the ability to persist data across sessions!
Do you have any other questions or tricks for saving data locally in Flutter? Let us know in the comments below! ππ
Happy Fluttering! ππ₯
Take Your Tech Career to the Next Level
Our application tracking tool helps you manage your job search effectively. Stay organized, track your progress, and land your dream tech job faster.
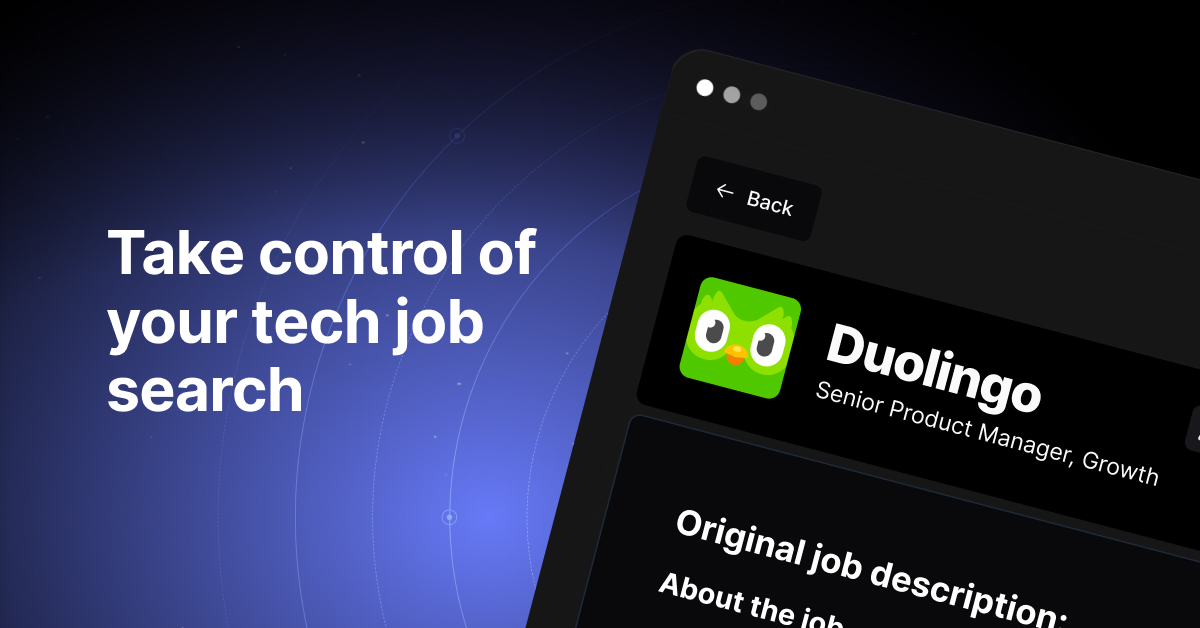