How to run code after some delay in Flutter?
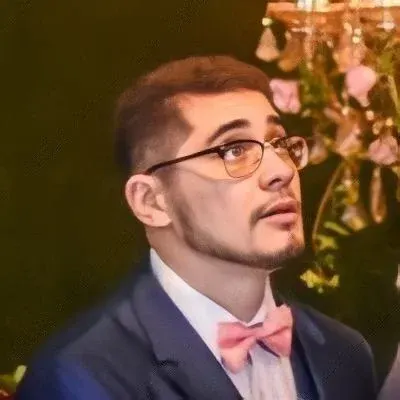
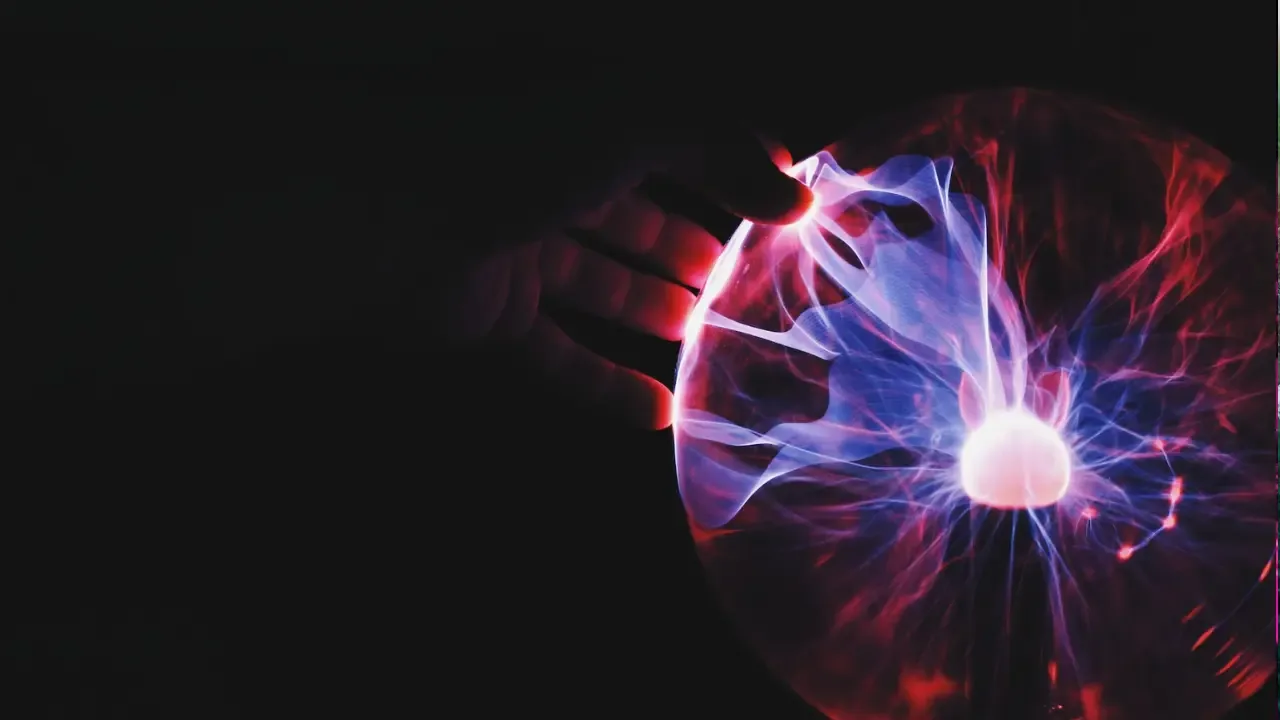
Running code after a delay in Flutter made easy! 😎
So, you want to execute a function after a certain delay in your Flutter app? No worries, we've got you covered! 🚀
The Problem
Let's understand the problem first. You have a widget and you want to perform some action, like changing the style property, after a certain duration.
The Solution
Flutter provides a simple and idiomatic way to achieve this using the Future
class and Future.delayed
method. Here's how you can do it:
import 'package:flutter/material.dart';
class MyDelayedWidget extends StatefulWidget {
@override
_MyDelayedWidgetState createState() => _MyDelayedWidgetState();
}
class _MyDelayedWidgetState extends State<MyDelayedWidget> {
@override
void initState() {
super.initState();
_startDelayedTask();
}
Future<void> _startDelayedTask() async {
await Future.delayed(Duration(seconds: 2)); // Change the duration as per your requirement
setState(() {
// Perform your action here, like changing the style property
});
}
@override
Widget build(BuildContext context) {
return FlutterLogo();
}
}
In the above example, we create a stateful widget MyDelayedWidget
that extends StatefulWidget
. In the state class _MyDelayedWidgetState
, we override the initState
method, which gets called when the widget is first created.
Inside the initState
method, we call a private method _startDelayedTask
. This method is marked async
to allow us to use the await
keyword. We use Future.delayed
to introduce the desired delay of 2 seconds (you can change it as per your requirement). Once the delay is over, we call setState
to update the widget and perform the desired action, like changing the style property.
In the build
method, we simply return a FlutterLogo
widget, which will be updated after the given delay.
Let's see it in action! 🎬
class MyApp extends StatelessWidget {
@override
Widget build(BuildContext context) {
return MaterialApp(
home: Scaffold(
appBar: AppBar(
title: Text('Delayed Flutter'),
),
body: Center(
child: MyDelayedWidget(),
),
),
);
}
}
In the build
method of your main app widget, you can simply add an instance of MyDelayedWidget
inside a Center
widget. The MyDelayedWidget
will do its magic and update the style property after the specified delay.
A little more customization 🎨
What if you want to execute different actions after different delays? For example, changing the style property after 2 seconds, and changing another property after 5 seconds?
You can achieve this by creating multiple methods _startDelayedTask
and calling them at appropriate places, as per your requirement.
Conclusion
Now you have the power to run code after a delay in your Flutter app. It's as easy as using Future.delayed
and setState
. Go ahead, give it a try, and level up your app's user experience! 🚀
If you have any questions or suggestions, feel free to leave a comment below. Happy coding! 💻
Keep Fluttering! 🦋
Take Your Tech Career to the Next Level
Our application tracking tool helps you manage your job search effectively. Stay organized, track your progress, and land your dream tech job faster.
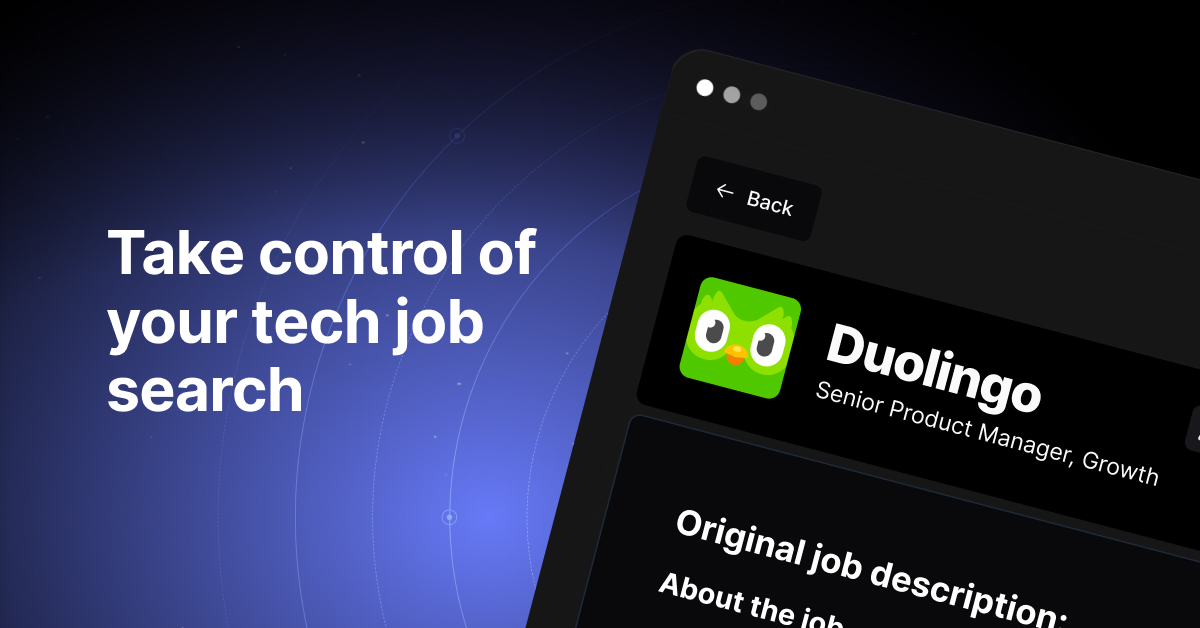