How to present an empty view in flutter?
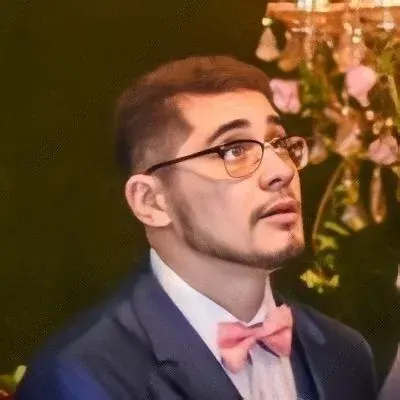
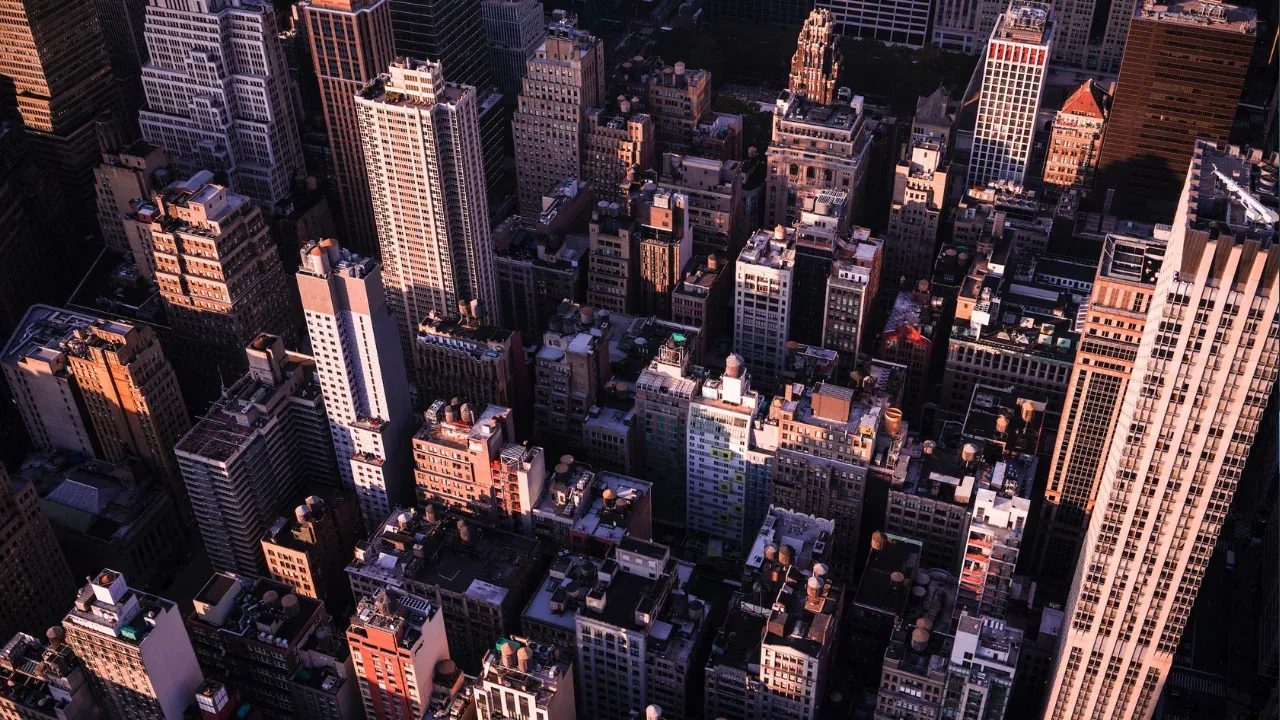
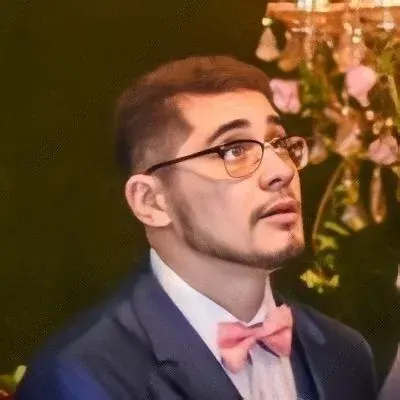
📝 Title: How to Present an Empty View in Flutter without Returning Null 😄📱💡
Hey there, Flutter enthusiasts! 👋 Are you struggling to present an empty view in your Flutter app without returning null? Don't worry, you're not alone! 😅 In this blog post, we'll dive into the common issue of representing an empty view in Flutter and provide easy solutions to help you overcome this challenge. Let's get started! 🚀
The Dilemma 😕
One of the common practices in Flutter is building UI widgets using the build
method. However, a challenge arises when you want to display an empty view while ensuring that Widget.build
doesn't return null. The question is, how do you tackle this situation? 🤔
The Problem Explained 🤓
The Flutter framework doesn't allow returning null from the build
method as it expects a valid widget to be rendered. Consequently, directly returning null to indicate an empty view simply won't work. 😫
Easy Solutions 💡
No worries, my fellow Flutter enthusiasts! We have a few easy solutions to help you present an empty view without resorting to returning null from the build
method. Let's check them out: 🌟
Solution 1: Wrap it in a Container 📦
Simply wrap your desired empty view in a Container
widget with zero dimensions. This effectively presents an empty view while still providing a valid widget to Flutter. Let's take a look at an example:
Widget build(BuildContext context) {
if (someCondition) {
return // your normal widget here
} else {
return Container(); // empty view
}
}
Solution 2: Utilize an Empty Container Widget 🗑️
Wouldn't it be great to have a reusable widget specifically designed for empty views? Well, good news - you can create your own! Here's a useful example of an EmptyContainer
widget:
class EmptyContainer extends StatelessWidget {
final Widget child;
EmptyContainer({required this.child});
@override
Widget build(BuildContext context) {
return someCondition ? child : Container();
}
}
You can then use it like this:
Widget build(BuildContext context) {
return EmptyContainer(
child: // your normal widget here,
);
}
Solution 3: Leverage the Visibility Widget 👀
Another approach is to make use of the Visibility
widget. By setting the child
property to null, you can achieve the desired empty view. Check it out below:
Widget build(BuildContext context) {
return Visibility(
visible: !someCondition, // show empty view if the condition is true
child: // your normal widget here,
);
}
You Did It! 🎉
Congratulations! You've learned three easy ways to present an empty view in Flutter without returning null. Now you can confidently represent empty states in your Flutter app with style. You're awesome! 💪😎
Conclusion: Keep Fluttering On! 🚀
Next time you face the challenge of presenting an empty view in Flutter without returning null, fear not! Remember these handy solutions: wrap it in a container, utilize an empty container widget, or leverage the visibility widget. With these techniques, you'll wow your users with sleek empty views. Embrace the Flutter way and keep on fluttering! 💙
Now it's your turn! Share your thoughts, experiences, or any other tips you have to tackle empty views in Flutter. We'd love to hear from you! 😊💬
Happy coding and happy Fluttering, everyone! ✨💻🔥
Remember to follow us for more exciting Flutter tips and tricks! 📚💡 And if you found this blog post useful, share the knowledge with your fellow Flutter enthusiasts! Let's spread the Flutter love! 🚀❤️