How to make button width match parent?
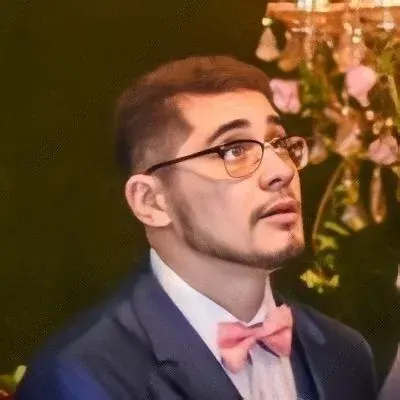
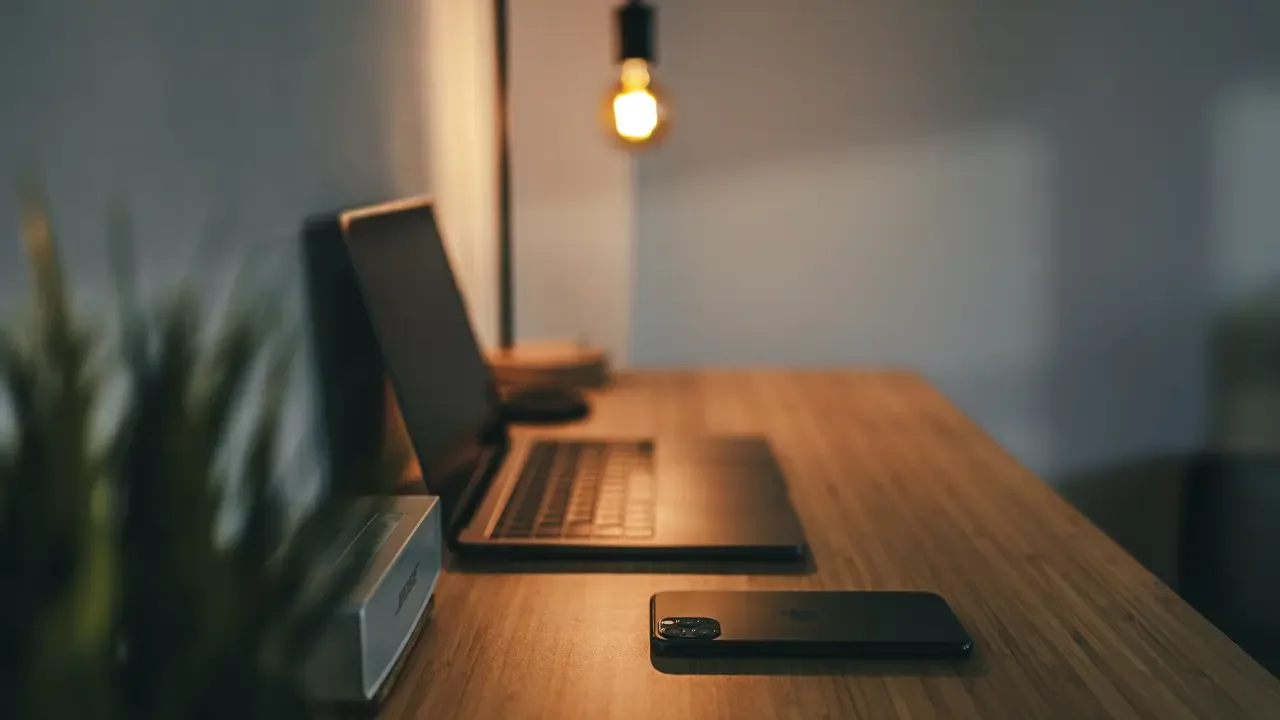
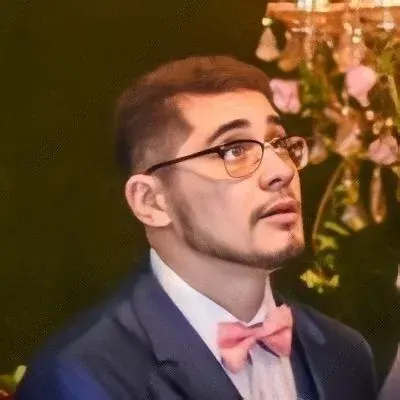
How to Make Button Width Match Parent: A Complete Guide
Have you ever struggled with aligning a button to match its parent widget's width? Don't worry, you're not alone! Many developers find it challenging to achieve this seemingly simple task. In this guide, we'll explore common issues, easy solutions, and empower you with the knowledge you need to conquer this problem. 🚀
The Problem
Let's take a closer look at a specific scenario:
new Container(
width: 200.0,
padding: const EdgeInsets.only(top: 16.0),
child: new RaisedButton(
child: new Text(
"Submit",
style: new TextStyle(
color: Colors.white,
)
),
colorBrightness: Brightness.dark,
onPressed: () {
_loginAttempt(context);
},
color: Colors.blue,
),
),
In the code above, you can see a Container
widget containing a RaisedButton
. The developer wants the button's width to match that of the parent Container
. However, the button does not automatically adjust its width.
The Solution
To solve this problem, you can use the Expanded
widget. Although the OP mentioned being unsure about it, don't worry, we'll guide you through it! 💪
new Container(
width: 200.0,
padding: const EdgeInsets.only(top: 16.0),
child: new Row(
children: <Widget>[
new Expanded(
child: new RaisedButton(
child: new Text(
"Submit",
style: new TextStyle(
color: Colors.white,
),
),
colorBrightness: Brightness.dark,
onPressed: () {
_loginAttempt(context);
},
color: Colors.blue,
),
),
],
),
),
In this updated code, we wrapped the RaisedButton
with a Row
widget. Inside the Row
, we added an Expanded
widget around the button. This allows the button to take up all available space within the Row
, effectively matching its parent's width.
Additional Tips
Here are a few extra tips to enhance your understanding and avoid common pitfalls:
Nested Layouts: If you encounter this issue within nested layouts, ensure that you apply the
Expanded
widget at the appropriate level. It should be placed within the same container (or its parent) that you want to match in width.Column Layout: If you're dealing with a vertical layout using
Column
, apply theExpanded
widget similarly. Wrap the desired widget withExpanded
and ensure it's placed within the same container or parent.
Conclusion and Your Turn!
You've learned a simple yet powerful solution to make a button's width match its parent. Now it's your turn to implement this technique in your own projects! Share your success stories, ask questions, and let us know how this guide helped you in the comments below. Together, we can conquer any layout challenges! 🎉👩💻👨💻
So, what are you waiting for? Go ahead and level up your button alignment game! 💪✨
[^1]: Example code sourced from: stackoverflow.com