How to hide soft input keyboard on flutter after clicking outside TextField/anywhere on screen?
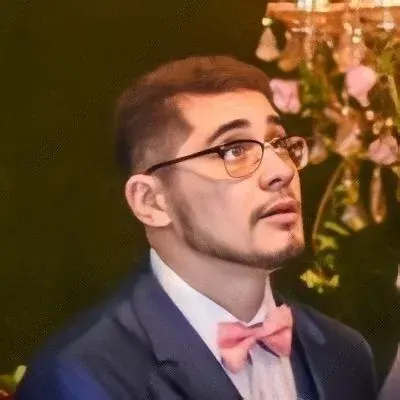
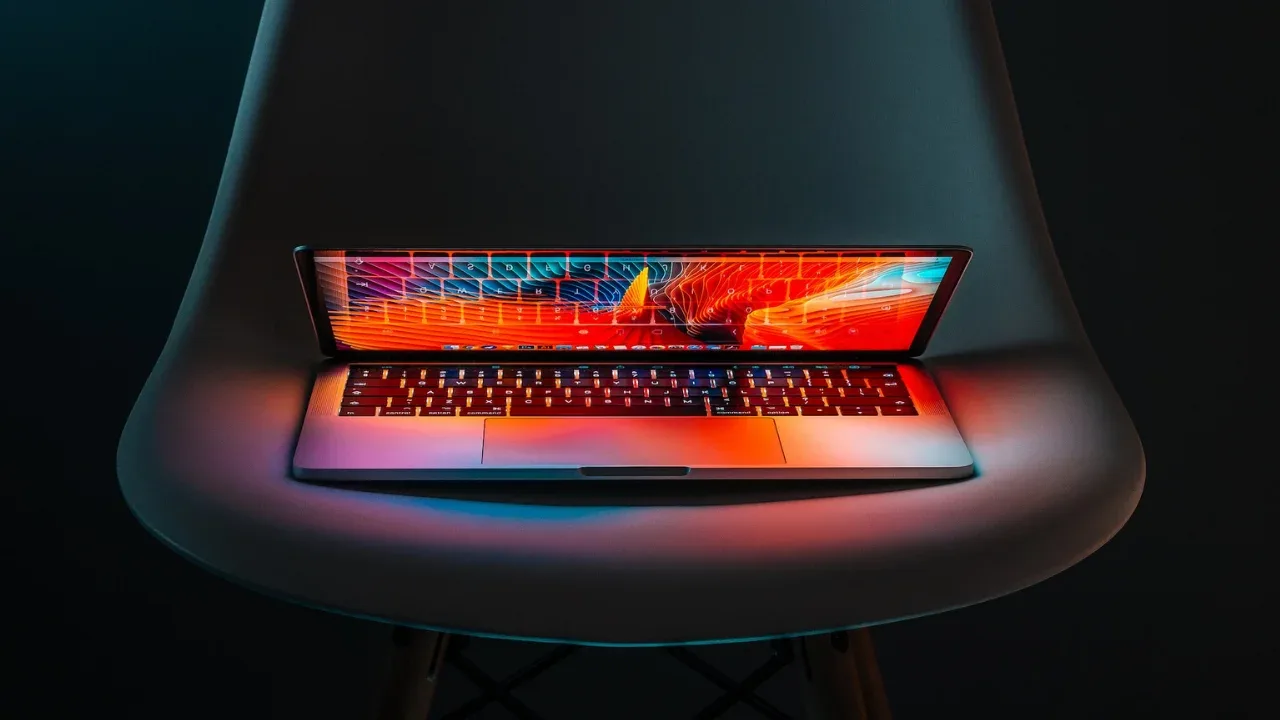
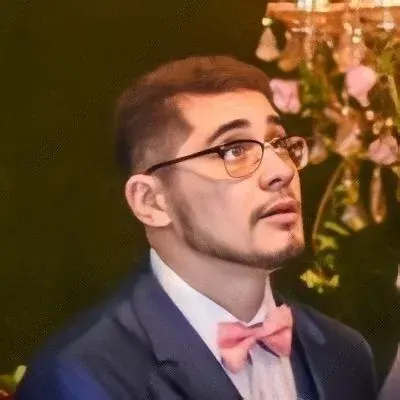
How to Hide Soft Input Keyboard on Flutter After Clicking Outside TextField/Anywhere on Screen 😎💻📱
Are you looking to enhance your Flutter app's user experience by allowing users to hide the soft input keyboard when they click outside of a TextField or anywhere on the screen? You've come to the right place! In this blog post, we'll address this common issue and provide you with easy solutions to implement this feature in your app. 🚀🔥
The Problem 🤔
By default, when users have the soft input keyboard open on their device while interacting with a TextField in your Flutter app, they need to tap the back button or tap on a button specifically designed to hide the keyboard. This can sometimes be inconvenient and disrupt the user's flow. So, how can we allow the user to hide the keyboard simply by tapping outside the TextField or anywhere on the screen? Let's find out! 🧐
Solution 1: Using GestureDetector 🖐️👆
One way to achieve this behavior is by utilizing the GestureDetector widget provided by Flutter. The GestureDetector widget listens to touch events on the screen and allows us to handle those events accordingly. Here's an example of how you can use it to hide the soft keyboard:
// Wrap your entire app or relevant portion of your widget tree with a GestureDetector
GestureDetector(
behavior: HitTestBehavior.translucent,
onTap: () {
// To hide the soft keyboard, remove the focus from the current TextField
FocusManager.instance.primaryFocus?.unfocus();
},
child: YourAppWidget(), // Replace this with your app's main widget
)
In the code snippet above, we wrap our app's widget tree with a GestureDetector. The behavior property is set to "translucent" to ensure that the GestureDetector detects taps on transparent areas of the screen. Inside the onTap callback, we simply remove focus from the current TextField by calling unfocus()
on the primaryFocus. This action will hide the soft keyboard. ✨🙌
Solution 2: Using an Overlay with Stack 🖥️🎛️
Another alternative to tackle this issue is by using an Overlay and Stack widgets combination. This solution is particularly useful when you want to have more control over the visibility of your app's components and handle more complex UI scenarios. Here's an example:
class YourAppState extends State<YourApp> {
OverlayEntry? overlayEntry;
@override
Widget build(BuildContext context) {
return GestureDetector(
behavior: HitTestBehavior.translucent,
onTap: () {
overlayEntry?.remove(); // Remove the overlay when tapped outside the TextField
},
child: Stack(
children: [
YourAppWidget(), // Replace this with your app's main widget
if (overlayEntry != null) OverlayWidget(), // Replace with your overlay widget
],
),
);
}
// Show the overlay when the TextField gains focus
void showOverlay() {
overlayEntry = OverlayEntry(builder: (context) => OverlayWidget());
Overlay.of(context)?.insert(overlayEntry!);
}
}
In this approach, we add a GestureDetector to our widget tree as before, but this time we use a Stack to stack our app's main widget and an optional OverlayWidget. The OverlayWidget can be shown when the TextField gains focus, and we remove it by calling remove()
on the OverlayEntry when the user taps outside the TextField. This way, we have more control over UI elements and can add additional interactive components if needed. 🔝💡
Conclusion and Next Steps 🎉🚀
Congrats! You've learned two easy solutions to hide the soft input keyboard on Flutter after clicking outside of a TextField or anywhere on the screen. You can choose the solution that best fits your app's requirements and implementation strategy. Now it's time to give it a try and enhance your app's user experience! 😊💪
Remember, creating a seamless and user-friendly app is crucial for engaging users and keeping them coming back. By implementing this feature, you can provide a smoother user experience and increase user satisfaction. So go ahead, apply what you've learned, and level up your Flutter app! And if you have any questions or suggestions, feel free to leave a comment below. We'd love to hear from you! 👇❓📝
Happy coding! 🎉💻✨