How to get build and version number of Flutter app
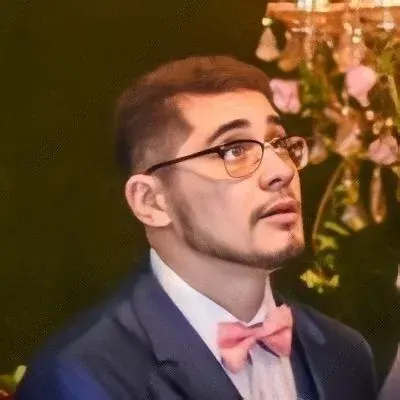
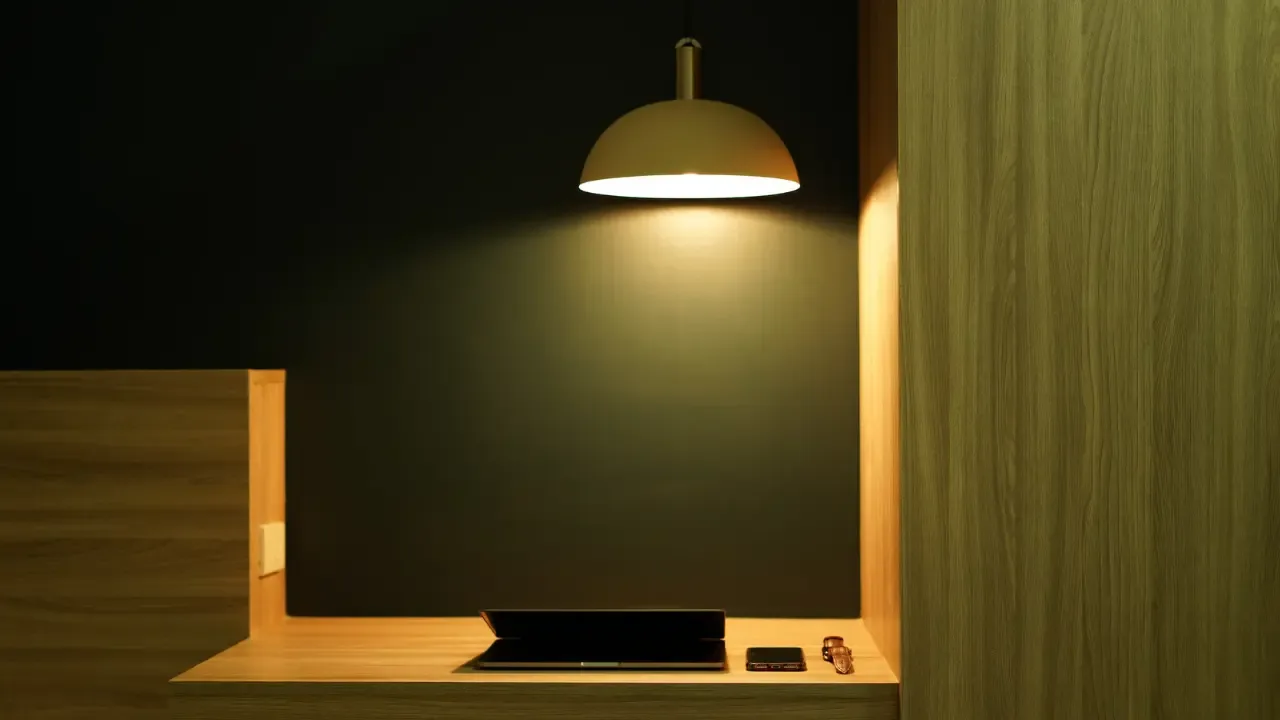
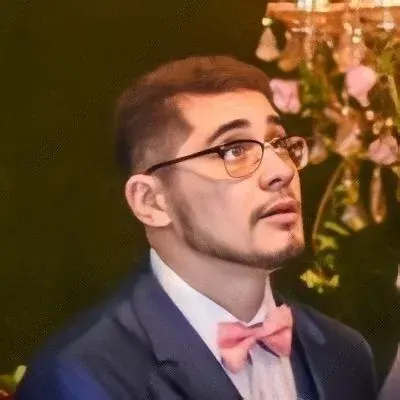
How to Get Build and Version Number of a Flutter App
š± Are you developing an awesome Flutter app? š Do you want to display the build and version number to your users? š·ļø No worries, we got you covered! In this guide, we'll walk you through the steps to get the build and version number of your Flutter app.
The Challenge
ā ļø Imagine you're developing an app in beta mode and want to show your users the version they are currently on. For example, "v1.0b10 - iOS". You've already tried using the code Text("Build: V1.0b10 - " + (Platform.isIOS ? "iOS" : "Android"))
, but you still need to fetch the actual build version and number dynamically.
The Solution
ā
To solve this challenge, we'll use the package called package_info
. This package provides access to various information about the application, including the version number and build number.
š” Here are the easy steps to get the build and version number of your Flutter app:
Add the
package_info
dependency to yourpubspec.yaml
file:
dependencies:
flutter:
sdk: flutter
package_info: ^2.0.3
Run
flutter pub get
to fetch the dependency.Import the
package_info
package in your Dart file:
import 'package:package_info/package_info.dart';
Get the build and version numbers using the
PackageInfo
class:
PackageInfo packageInfo = await PackageInfo.fromPlatform();
String version = packageInfo.version;
String buildNumber = packageInfo.buildNumber;
Use the obtained
version
andbuildNumber
variables wherever you want to display the information in your app:
Text("Build: ${version}b${buildNumber} - ${Platform.isIOS ? "iOS" : "Android"}")
// Example output: "Build: v1.0b10 - iOS"
That's it! š You will now be able to display the actual build and version number dynamically in your Flutter app.
Call-to-Action
š£ Now that you know how to get the build and version number of your Flutter app, go ahead and implement it in your project! š Show your users that you care about providing them the latest updates and improvements. š
ā Do you have more questions or encountered any issues along the way? Let us know in the comments section below! Our amazing community is always here to help you out. š¤
š Don't forget to share this blog post with your fellow Flutter developers! Sharing is caring. ā¤ļø
Happy coding! š»āØ