How to deactivate or override the Android "BACK" button, in Flutter?
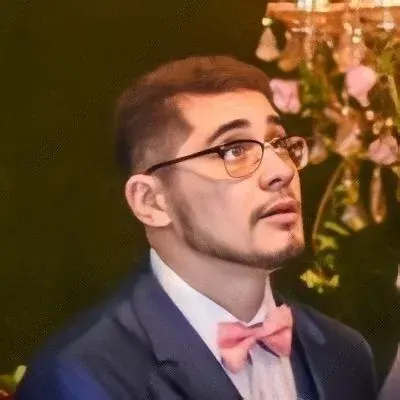
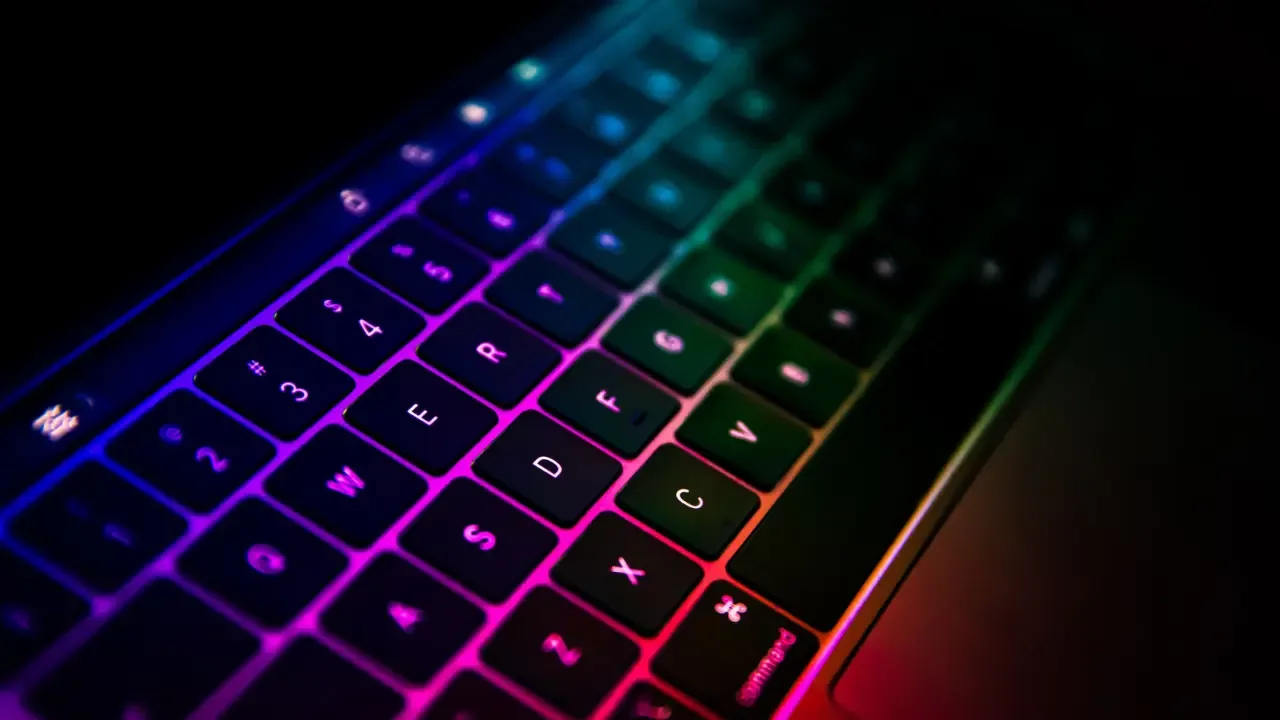
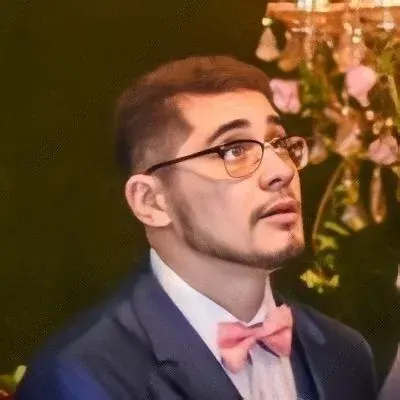
How to Deactivate or Override the Android "BACK" Button in Flutter?
Are you facing issues with the Android "Back" button in your Flutter app? Do you want to disable or override the functionality of the back button on certain screens? We understand your dilemma, and we're here to help!
The Challenge
In Flutter, the Android "Back" button is a system-level button that allows users to navigate back to the previous screen. However, in certain cases, you may want to disable or override this functionality to create a more controlled user experience.
The Solution
To address this problem, we can leverage the WillPopScope
widget in Flutter. This widget allows us to handle the back button press and define actions accordingly. Here's how you can implement it:
Wrap your widget that needs back button customization with the
WillPopScope
widget.
class WakeUpApp extends StatelessWidget {
@override
Widget build(BuildContext context) {
return MaterialApp(
title: "Time To Wake Up?",
home: WillPopScope(
onWillPop: () async {
// Handle your custom behavior here
return false; // Return true to allow back navigation or false to prevent it
},
child: WakeUpHome(),
),
routes: <String, WidgetBuilder>{
'/pageOne': (BuildContext context) => pageOne(),
'/pageTwo': (BuildContext context) => pageTwo(),
},
);
}
}
Inside the
onWillPop
method, you can define your custom behavior when the back button is pressed. For example, if you want to disable the back button completely, you can returnfalse
. If you want to allow back navigation, you can returntrue
. To disable the back button based on a condition (e.g., keeping the finger pressed for 5 seconds), you can use timers, flags, or state management techniques.
onWillPop: () async {
// Conditionally disable the back button
bool isParent = // Check if user is a parent
if (isParent) {
await Future.delayed(Duration(seconds: 5)); // Wait for 5 seconds
return false; // Disable the back button after 5 seconds
}
return true; // Allow back navigation for other users
},
And that's it! You have successfully deactivated or overridden the Android "Back" button in your Flutter app for specific screens.
Conclusion
Effectively managing the functionality of the Android "Back" button in Flutter can enhance the user experience of your app. By utilizing the WillPopScope
widget and customizing the onWillPop
function, you can easily deactivate or override the back button behavior based on your app's specific requirements.
Remember to keep the user's needs in mind when implementing these changes. Providing clear instructions or visual cues can prevent frustration and ensure a seamless user experience.
Now it's your turn! Try implementing the suggested solution in your Flutter app and let us know how it works for you.
👉 Have you encountered any other unique challenges while working with Flutter? Share your experiences, questions, or suggestions in the comments below. Let's learn and grow together! 🚀