How to create a hyperlink in Flutter widget?
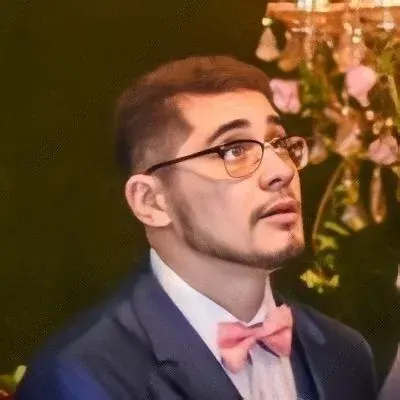
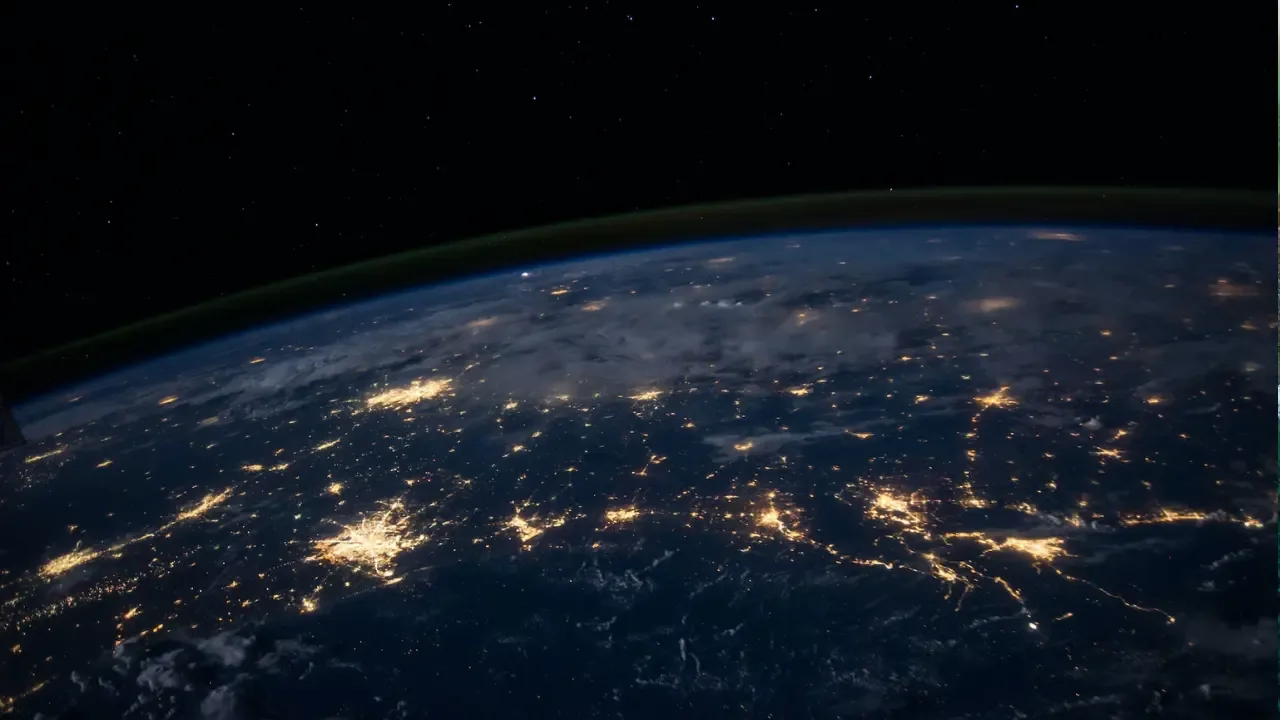
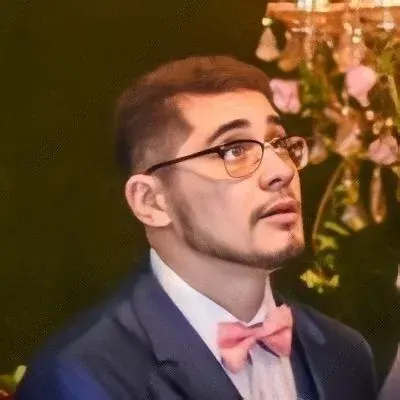
How to Create a Hyperlink in Flutter Widget? 🌐
Are you looking to add a hyperlink to your Flutter app? 📱💡 Creating a clickable link within a text can be a powerful way to share information and engage users. In this blog post, we'll explore how to easily create hyperlinks within Flutter widgets, such as Text
, and provide simple solutions to common challenges. So, let's dive in!
The Problem 😫
The challenge lies in embedding a hyperlink within a Text
widget or similar text views. The example provided in the question shows an attempt to create a hyperlink using HTML-like syntax:
The last book bought is <a href='#'>this</a>
However, Flutter does not support rendering HTML tags directly in its widgets. So, how can we achieve this desired hyperlink effect? Let's find out! 🕵️♂️
Solution 1: Using RichText
📝
Flutter provides us with the RichText
widget, which offers more advanced text formatting options than the basic Text
widget. It allows us to create custom-styled text spans, including hyperlinks. Here's how you can use RichText
to create a hyperlink:
RichText(
text: TextSpan(
text: 'The last book bought is ',
children: [
TextSpan(
text: 'this',
style: TextStyle(
color: Colors.blue,
decoration: TextDecoration.underline,
),
recognizer: TapGestureRecognizer()
..onTap = () {
// Code to execute when the link is tapped
},
),
],
),
)
In the above example, we define a TextSpan
as a child of RichText
. The TextSpan
is responsible for rendering the hyperlink text ('this') with the desired style (TextStyle
). We use the TapGestureRecognizer
from the flutter/gestures.dart
package to handle taps on the hyperlink, allowing us to execute custom code when the link is tapped.
Solution 2: Using the Link
package 📦
If you prefer a more convenient solution, you can use the Link
package, which simplifies the process of creating hyperlinks in Flutter. To use the Link
package, follow these steps:
Add the
link
package to your pubspec.yaml file:dependencies: link: ^1.1.1
Import the package in your Dart file:
import 'package:link/link.dart';
Wrap your hyperlink text with the
Link
widget:Link( child: Text('The last book bought is this'), url: '#', onError: (LinkError? error) { // Handle any errors that occur }, );
The Link
widget takes a child
argument, which can be any widget, and a url
argument, which specifies the URL to navigate when the link is tapped. The onError
callback allows you to handle any errors that occur during navigation.
Time to Create Awesome Hyperlinks! 🚀💪
With these solutions at your fingertips, you're now equipped to create hyperlinks in your Flutter app with ease! Whether you choose to use the RichText
widget or the Link
package, your users will appreciate the ability to interact with your app's content in a more dynamic way.
Have any other Flutter-related questions or cool tips to share? Leave a comment below and let's start a conversation! 😄🎉
Happy coding! 💙🚀