How to change the appBar back button color
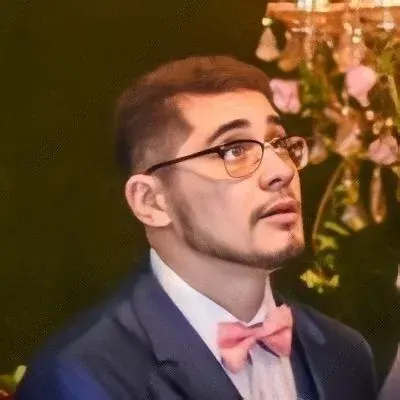
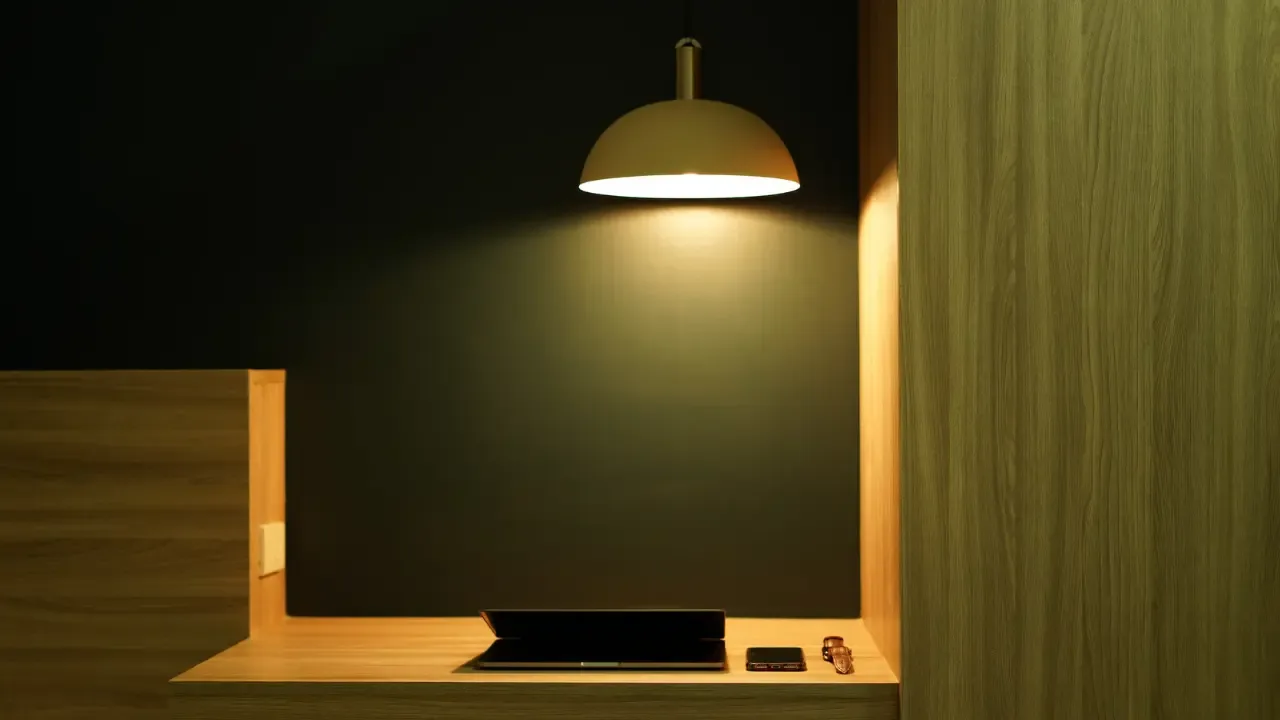
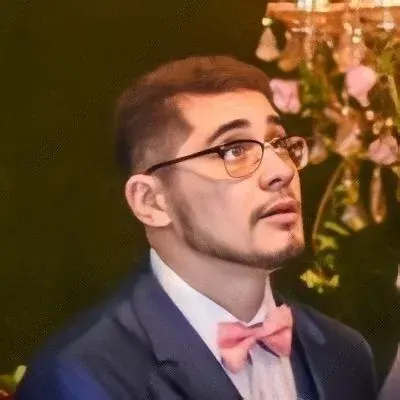
How to Change the AppBar Back Button Color
š Are you struggling to change the color of the automatic back button on your AppBar? We've got you covered! In this blog post, we'll address this common issue and provide you with easy solutions. Let's dive in! š
Understanding the Problem
š It seems that you want to customize the color of the automatic back button in your AppBar. However, you are unable to figure out how to make it work. Let's break it down to understand the problem better.
š In the provided code snippet, we can see that the AppBar is already customized with a white background color and an image as the title. However, the back button inherits the system default color and doesn't match the desired style.
š” Changing the color of the back button is not as straightforward as changing the AppBar's background color. It requires a bit of additional effort. But don't worry, we've got some simple solutions for you to try! šŖ
Solution 1: Using the automaticallyImplyLeading
Property
š ļø The automaticallyImplyLeading
property determines whether the AppBar should automatically show the default back button. By setting it to false, we can customize the back button ourselves. Let's see how it works.
First, modify your code like this:
appBar: AppBar(
backgroundColor: Colors.white,
title: Image.asset(
'images/.jpg',
fit: BoxFit.fill,
),
centerTitle: true,
automaticallyImplyLeading: false, // Add this line
),
š Now, the back button should be hidden because we disabled the default implementation.
Next, to add a custom back button with a different color, we can use the leading
property. Here's an example:
appBar: AppBar(
backgroundColor: Colors.white,
title: Image.asset(
'images/.jpg',
fit: BoxFit.fill,
),
centerTitle: true,
automaticallyImplyLeading: false,
leading: IconButton(
icon: Icon(
Icons.arrow_back,
color: Colors.red, // Customize the color here
),
onPressed: () {
// Implement your back button behavior here
},
),
),
š” Feel free to choose any color you desire for your back button by specifying it in the color
parameter.
Solution 2: Theming with AppBarTheme
š ļø If you prefer a more global approach to customizing your AppBar, you can utilize the AppBarTheme
and appBarTheme
properties available in the Theme
component to ensure consistent styling across your application.
Add this code snippet to your MaterialApp
or Theme
widget:
theme: ThemeData(
appBarTheme: AppBarTheme(
backgroundColor: Colors.white,
iconTheme: IconThemeData(
color: Colors.blue, // Set your desired color here
),
),
),
š By setting the iconTheme
property within appBarTheme
, you can define the color of the back button globally for all your AppBar instances.
Wrapping Up
š Congratulations! š You have successfully learned how to change the color of the AppBar back button. Whether you prefer a local or global approach, we've covered both solutions for you.
š” Remember, the automaticallyImplyLeading
property and the leading
property provide you with a localized way to customize the back button. On the other hand, theming with AppBarTheme
allows you to set a global style.
We hope this guide has helped you overcome your issue and achieve the desired customization. Now it's your turn to implement these solutions!
š¬ Drop a comment below and let us know which solution worked best for you. If you have any further questions or suggestions, we'd love to hear from you! Happy coding! ā¤ļøļø