How to add image in Flutter
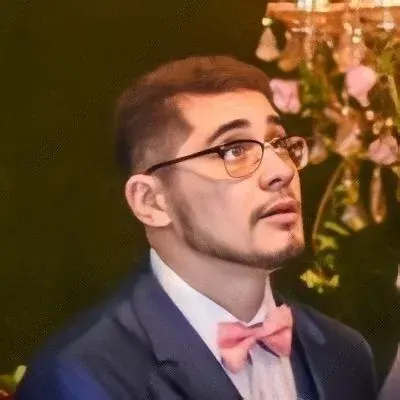
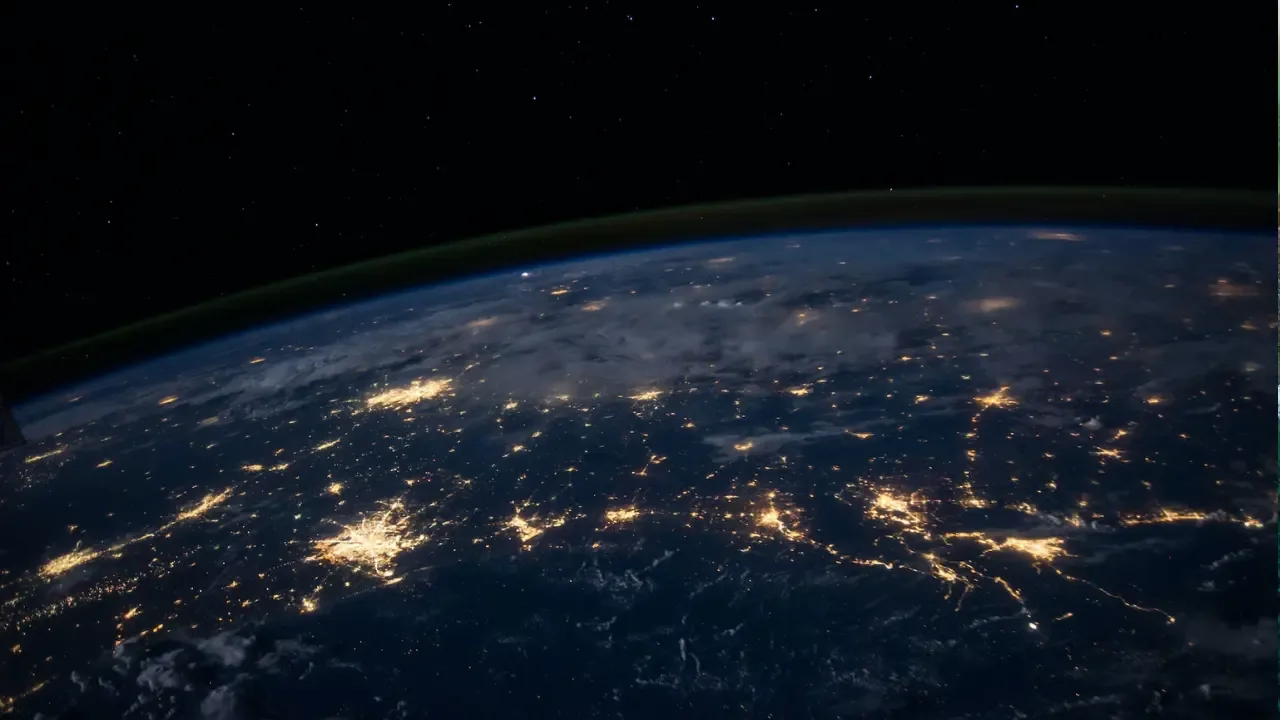
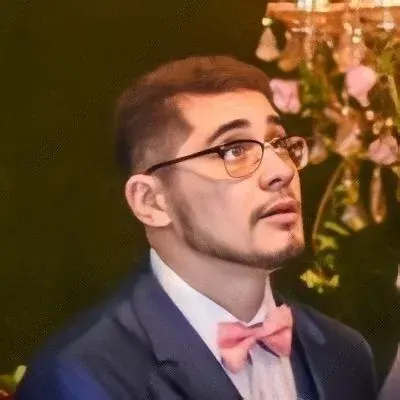
How to Add an Image in Flutter: A Simple Guide
š Hey there! Are you developing a Flutter app for the first time and facing issues with adding an image? Don't worry, we've got you covered! In this guide, we'll address common questions and provide easy solutions to help you add an image to your Flutter app. Let's dive in! š
1. Creating an Images Folder
The first question you might have is where to create the images folder. In Flutter, it's recommended to create an assets folder at the root of your project. This is typically where you'll store all your app's assets, including images.
2. Adding the Assets Tag in pubspec.yaml
To ensure that Flutter recognizes and includes your images in your app, you need to add the assets tag in the pubspec.yaml
file. Open your pubspec.yaml
file and add the following code:
flutter:
assets:
- assets/images/
Make sure to replace assets/images/
with the path to your actual images folder.
3. Organizing the Assets Folder
Now, let's talk about the assets folder. While it's not mandatory to have a specific folder for your images inside the assets folder, it's considered a good practice for better organization. You can create an images folder inside your assets folder and store all your images there.
4. Adding Images in Your Code
Once you've set up the assets folder and added the assets tag in your pubspec.yaml
file, you can now easily add images in your Flutter code.
In your main.dart
file, locate the part where you want to add the image. To add an image, use the Image.asset
widget and provide the path to your image file:
Image.asset(
'assets/images/lake.jpg',
width: 600.0,
height: 240.0,
fit: BoxFit.cover,
),
Make sure to replace assets/images/lake.jpg
with the path to your actual image file.
Common Issue: Gradle Build Failure
If you encounter a gradle build failure with an error log similar to the one below:
ERROR: Exiting with exit code 1
Finished with error: Gradle build failed: 1
First, ensure that the path to your asset in the pubspec.yaml
file is correct. Next, try running the build command with additional options like --stacktrace
, --info
, or --debug
for more detailed logs. You can also try restarting your IDE or performing a clean rebuild using the IDE's or Flutter's built-in options.
Clean Rebuild in Flutter
Regarding your question about tools for a clean rebuild in Flutter, you can perform a clean rebuild using either the IDE's built-in option or Flutter's command-line tool. In Flutter, you can use the following command:
flutter clean
This command will delete the build directory, ensuring a fresh start for your rebuild.
Feel Free to Dive Deeper
That's it! You now know how to add an image in Flutter. š If you want to further explore Flutter's layout capabilities, we recommend checking out the tutorial you mentioned: https://flutter.io/tutorials/layout/
If you have any more questions or need additional guidance, feel free to leave a comment below. We love engaging with our readers! Let's build amazing Flutter apps together! š