How to add clear button to TextField Widget
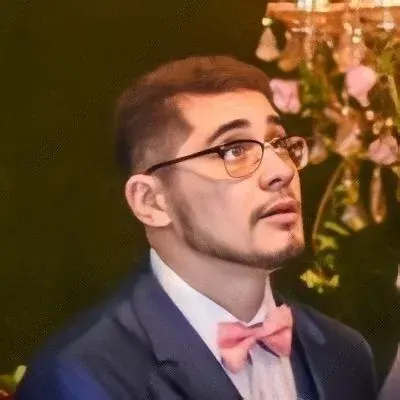
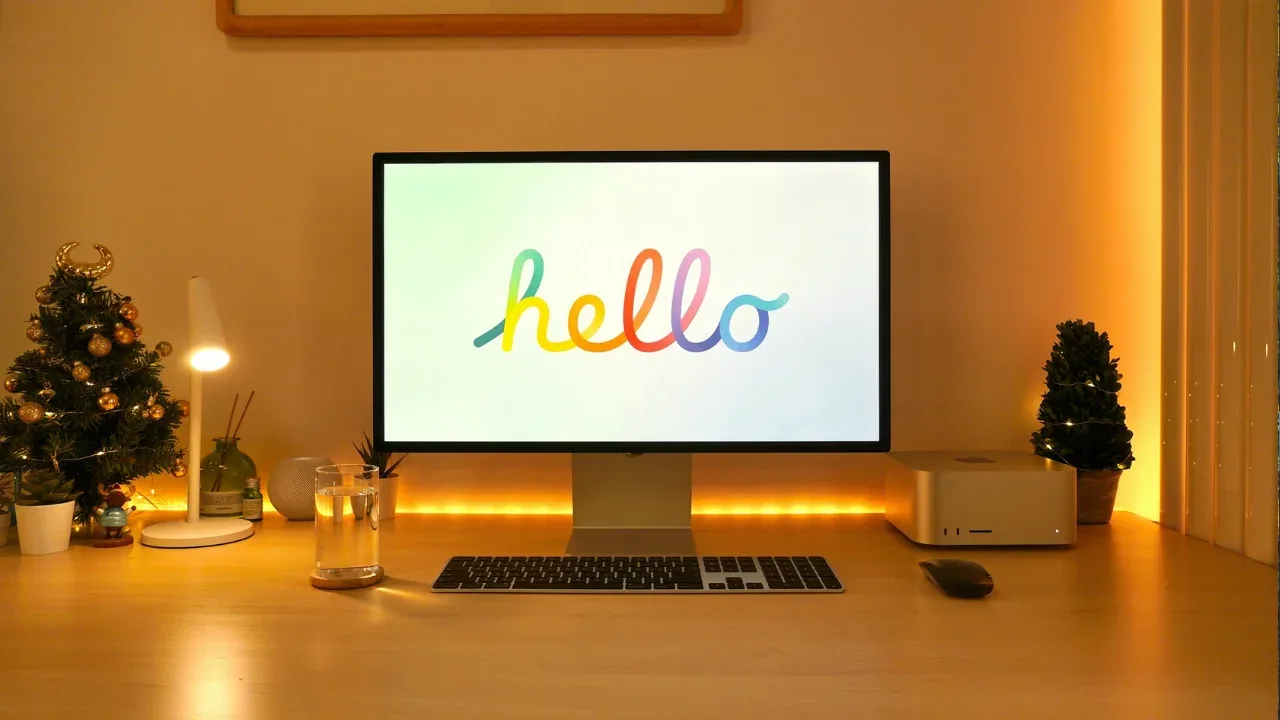
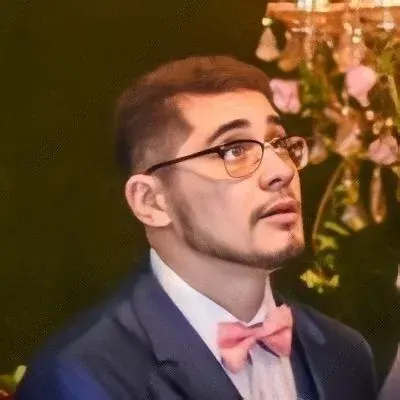
Adding a Clear Button to the TextField Widget: A Step-by-Step Guide
Are you looking for a way to add a clear button to the TextField
widget in your app? Perhaps you've come across the Material Design guidelines that showcase a clear button for text inputs. Well, you're in luck! In this guide, we'll walk you through the process of adding a clear button to your TextField
widget. Let's get started!
The Issue and Common Problems
Before we dive into the solution, let's address the issue at hand. Many developers struggle with finding the right way to add a clear button to the TextField
. While there are multiple approaches available, some may be more complex than others and might not provide the desired functionality. The main problem is finding a reliable and efficient solution that follows best practices.
The Solution: Setting a Clear IconButton
The good news is that there is a simple and effective solution to adding a clear button to the TextField
widget. One common way to achieve this is by setting a clear IconButton
in the InputDecoration
's suffixIcon
.
Here's an example of how you can implement this solution:
TextField(
decoration: InputDecoration(
labelText: 'Enter your text',
suffixIcon: IconButton(
icon: Icon(Icons.clear),
onPressed: () {
// Logic to clear the text in the TextField
},
),
),
)
In the example above, we create a TextField
widget with an InputDecoration
. Inside the InputDecoration
, we set the suffixIcon
property to an IconButton
widget. The IconButton
displays the clear icon from the Material Design icons library. Additionally, we attach an onPressed
callback to the IconButton
to handle the clearing of the text when it is pressed. You can modify the logic inside the onPressed
callback to fit your specific requirements.
Additional Customizations
Although the solution above provides a basic implementation of the clear button, you can further customize it to align with your app's design and behavior. Here are a few examples of additional customizations:
Changing the Clear Button's Appearance
To change the clear button's appearance, you can modify the properties of the IconButton
. For example, you can set a different icon color, size, or add animation effects. Explore the various options available in the Material Design icon library to find a suitable icon that fits your design.
Fine-tuning Clear Button Behavior
By leveraging the onChanged
callback of the TextField
, you can customize the behavior of the clear button even further. For instance, you can conditionally hide or show the clear button based on the text input's state. You can also implement complex validation or formatting logic triggered by the onChanged
event.
Get Creative and Share Your Experience!
Now that you know how to add a clear button to the TextField
widget, why not take it a step further? Experiment with different icon styles, animations, or even create a more advanced clear button functionality. Share your experience and the creative solutions you come up with in the comments below!
Conclusion
Adding a clear button to the TextField
widget can greatly enhance the user experience of your app. By following the steps and tips outlined in this guide, you should now be able to easily incorporate this feature into your project. Remember to always consider your app's design guidelines and user expectations when implementing custom features. Have fun coding, and don't forget to clear those text inputs like a pro! 💪🔥
What do you think about adding a clear button to the TextField
widget? Did you find this guide helpful? Share your thoughts and experiences in the comments below!