How should I check if the input is an email address in Flutter?
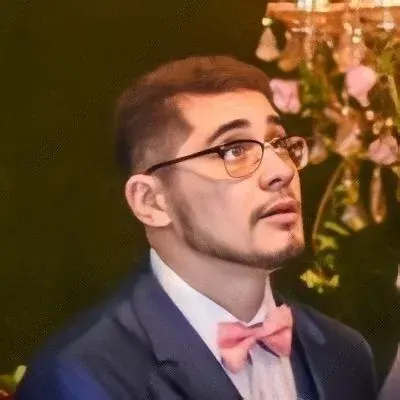
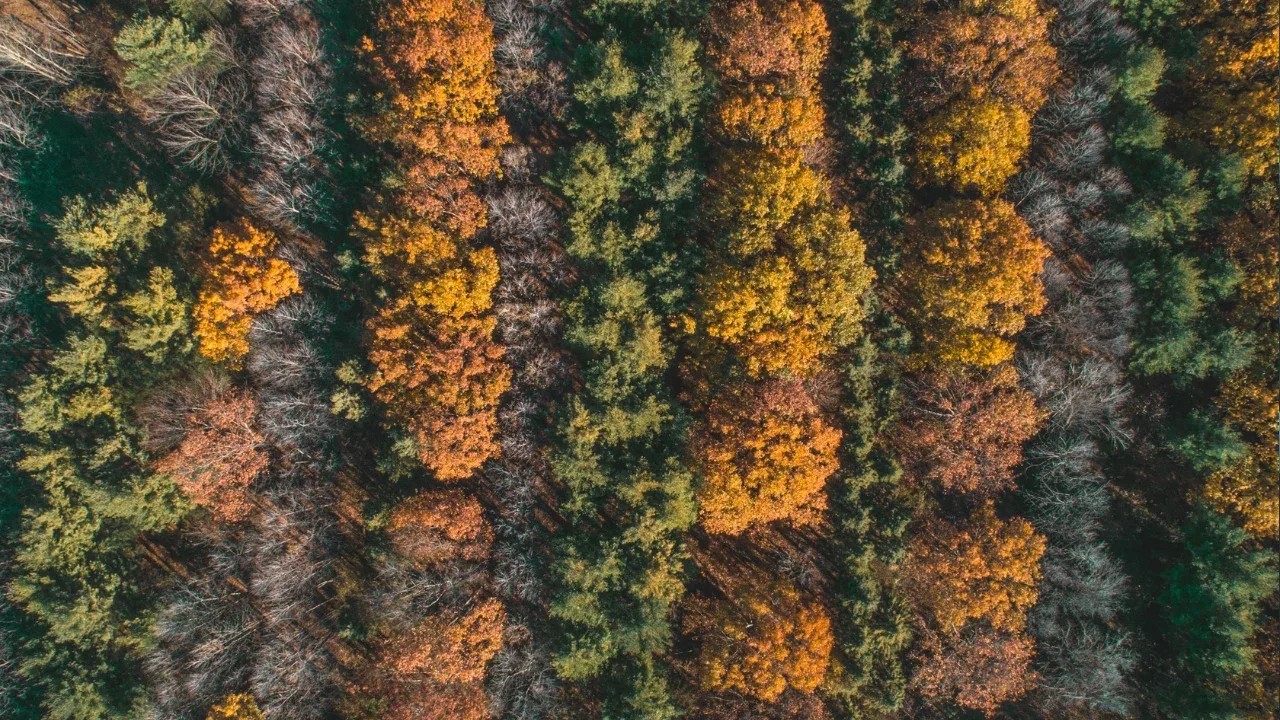
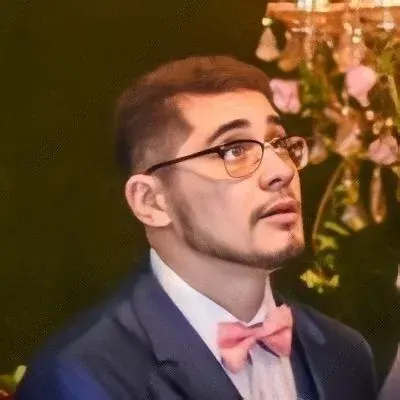
📝 Hey there tech enthusiasts! Are you wondering how to check if the input is an email address in Flutter? 📧 Look no further, because we've got you covered! In this blog post, we will address common issues and provide easy solutions for identifying email addresses in your Flutter applications. So, let's dive right in! 💪
✋ Before we get started, it's important to note that we need to use regular expressions (regex) to efficiently validate email addresses. In this case, we'll be using JavaScript (Perl 5) regular expressions, which are also supported in Dart. According to the RegExp documentation, you can find the ECMA specification for regex objects. Let's move on and explore the methods we can use in Dart to tackle this challenge! 🕵️♂️
1️⃣ Using RegExp Match Method:
The easiest and most straightforward way to check if the input is an email address is by using the RegExp match
method. Here's an example of how you can implement it in your Flutter code:
String email = "test@example.com";
RegExp regex = RegExp(r'^[\w-]+(?:\.[\w-]+)*@(?:[\w-]+\.)+[a-zA-Z]{2,7}$');
bool isEmail = regex.hasMatch(email);
if (isEmail) {
print("Valid email address!");
} else {
print("Invalid email address!");
}
In the example above, we define a regular expression pattern using the RegExp
constructor. The pattern checks for the correct email format and ensures that the top-level domain (TLD) is between 2 and 7 characters long. The hasMatch
method returns a bool
indicating whether the input matches the pattern. You can easily customize the pattern to suit your specific needs.
2️⃣ Using EmailValidator Package:
Another great option for email address validation is using the email_validator
package. To get started, add the following dependency to your pubspec.yaml
file:
dependencies:
email_validator: ^2.0.1
After running flutter pub get
, you can use the package in your code like this:
import 'package:email_validator/email_validator.dart';
String email = "test@example.com";
bool isEmail = EmailValidator.validate(email);
if (isEmail) {
print("Valid email address!");
} else {
print("Invalid email address!");
}
The EmailValidator
class provides a simple and convenient way to validate email addresses. It also ensures that the local part and the domain part of the email address are valid.
3️⃣ User-friendly Input Validation: When checking for email addresses in a Flutter application, it's important to provide real-time feedback to the user. You can use Flutter's TextFormField widget to achieve this. Here's an example:
TextEditingController emailController = TextEditingController();
TextFormField(
controller: emailController,
keyboardType: TextInputType.emailAddress,
decoration: InputDecoration(
labelText: "Email Address",
errorText: !EmailValidator.validate(emailController.text)
? "Invalid email address"
: null,
),
);
By utilizing the EmailValidator
class in conjunction with a TextFormField widget, you can display an error message when the user enters an invalid email address. This enhances the user experience by giving them immediate feedback.
🚀 And there you have it! You now have multiple options for checking if the input is an email address in Flutter. Whether you prefer regular expressions or using a dedicated package, the choice is yours! Why not give it a try and level up your Flutter game today? 😉✨
📢 We would love to hear your thoughts and experiences! Have you faced any challenges while validating email addresses in Flutter? Share them with us in the comments below. And don't forget to hit that share button to spread the Flutter love! 💙🚀