How do you detect the host platform from Dart code?
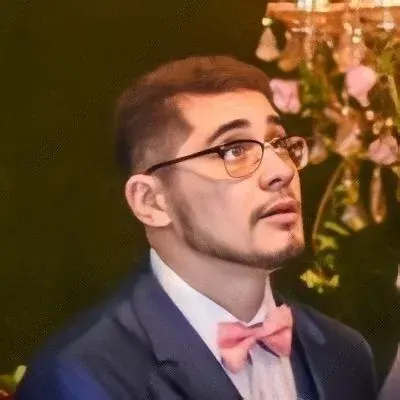
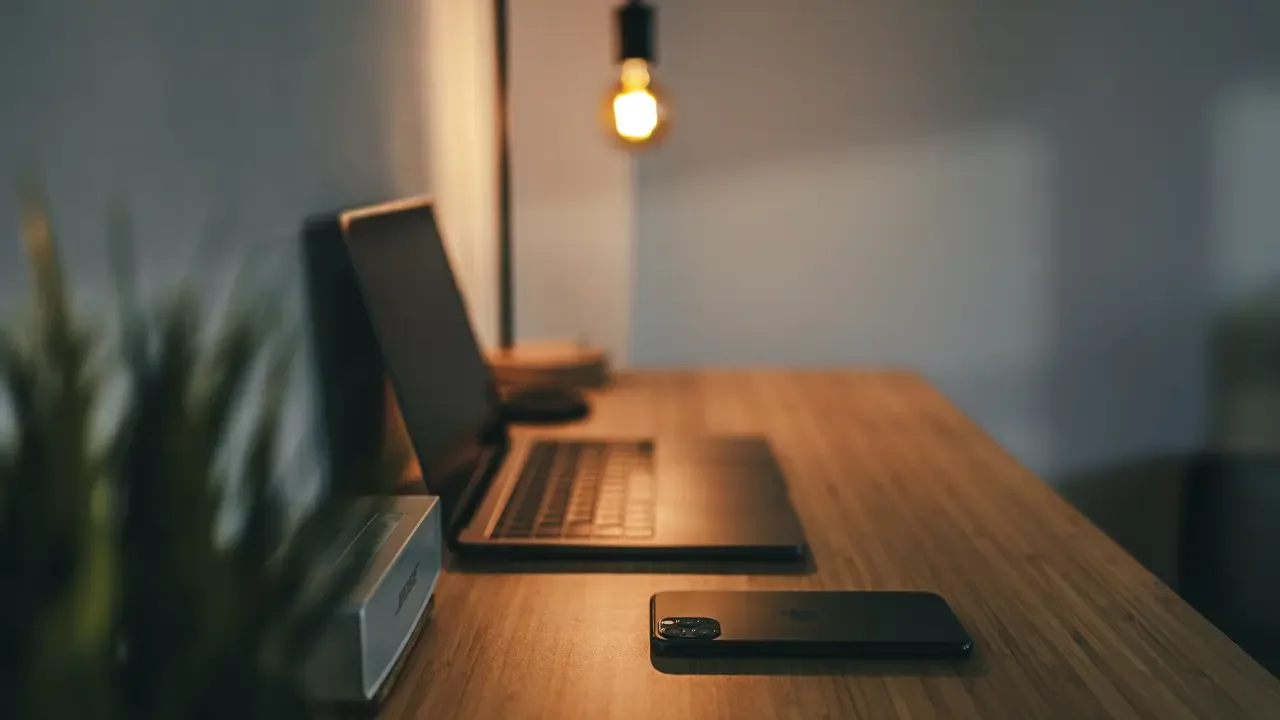
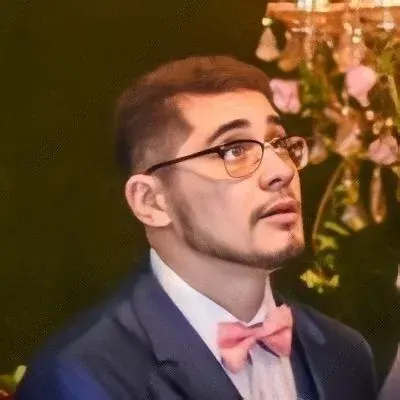
How to Detect the Host Platform from Dart Code? 📱💻
Are you building a mobile app with Dart and want to customize the user interface for different platforms? Look no further! In this guide, we will show you how to detect the host platform in your Dart code, allowing you to provide platform-specific UI tweaks. Let's dive right in! 💪🏼
The Dilemma: UI Customization for Different Platforms 😕
Imagine you have developed a mobile app that should have slightly different UI layouts and behaviors on iOS and Android. For example, you might want to use different fonts, icons, or colors tailored to each platform's design guidelines. But how do you determine which platform the app is currently running on? 🤔
The Quest for the Solution 🕵️♂️
You scoured the Dart documentation, hoping to find a built-in method or library that could easily provide the information you needed. Unfortunately, your search came up empty-handed. 😢
💡 The Solution: Flutter Platform Detection Plugin
While Dart doesn't have a direct way to detect the host platform, you can leverage the Flutter Platform Detection plugin to solve this problem effortlessly. This plugin provides a simple API to identify the platform, making it a go-to solution for many Flutter developers.
To begin, let's add the Flutter Platform Detection plugin to your pubspec.yaml
file:
dependencies:
flutter_platform_detection: ^2.1.4
Next, run flutter pub get
to fetch the plugin's latest version and get it ready for use.
Implementing Platform Detection in Your Dart Code 🚀
With the Flutter Platform Detection plugin installed, you can now utilize its API to detect the host platform in your Dart code.
Here's an example of how you could use the plugin:
import 'package:flutter_platform_detection/flutter_platform_detection.dart';
void main() {
final platform = getPlatform();
if (platform.isIOS) {
// Customize UI for iOS
print('Running on iOS');
} else if (platform.isAndroid) {
// Customize UI for Android
print('Running on Android');
} else {
// Handle other platforms (if necessary)
print('Running on an unknown platform');
}
}
By calling getPlatform()
from Flutter Platform Detection and using its properties such as isIOS
and isAndroid
, you can easily customize your UI based on the host platform.
Engage and Share Your Experiences! 📢
Now that you know how to detect the host platform from your Dart code, it's time to put this knowledge into action! Customize your app's UI for iOS and Android to deliver an optimal and platform-specific user experience. 😍
Share your experiences with platform detection in the comments below. Have you encountered any challenges or found alternative solutions? Let's spark a conversation and help fellow developers overcome platform-related hurdles together!
Remember, the Flutter Platform Detection plugin is just one tool in your arsenal. Explore other libraries and techniques to enhance your app's cross-platform compatibility and delightful user experience. Happy coding! 💻✨
Stay tuned for more tech guides, tips, and tricks on our blog. Don't miss out - subscribe to our newsletter for the latest updates straight to your inbox! 💌
Note: The Flutter Platform Detection plugin mentioned in this guide is accurate as of the writing date. Ensure you check for any updates or changes in the plugin's documentation for future compatibility.