How do you change the value inside of a textfield flutter?
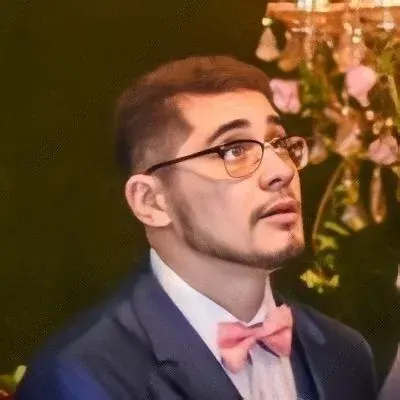
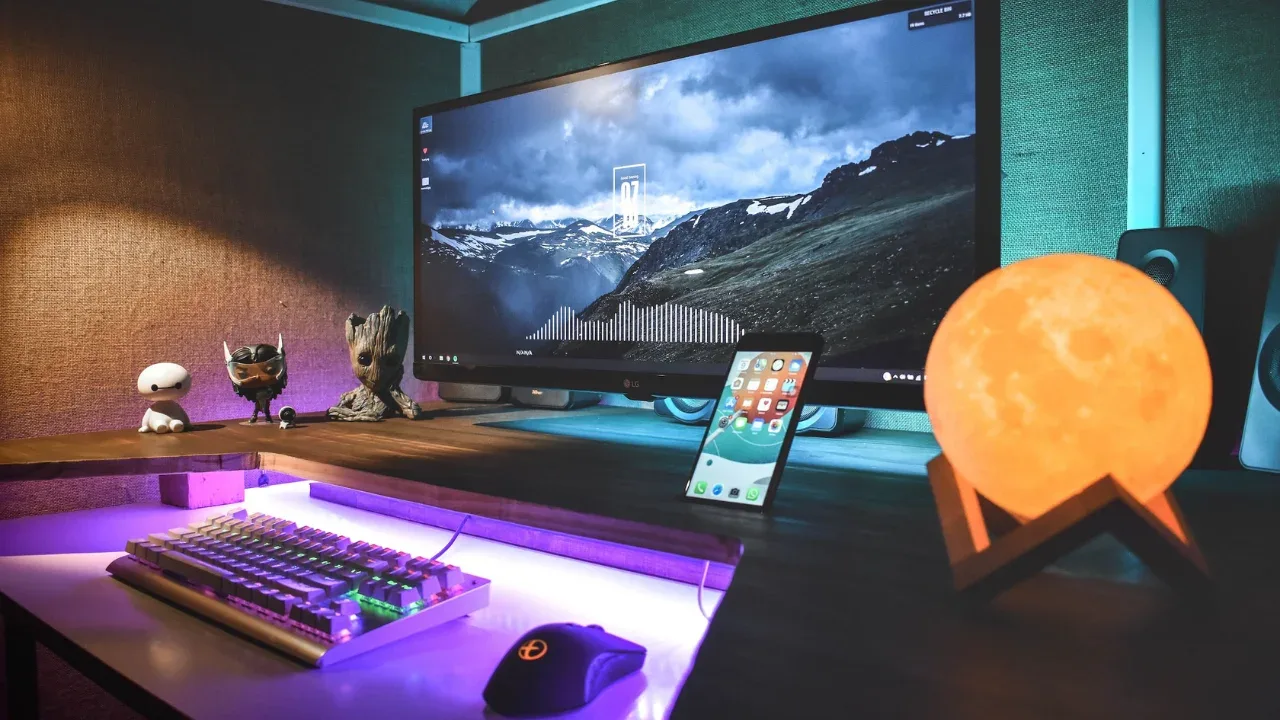
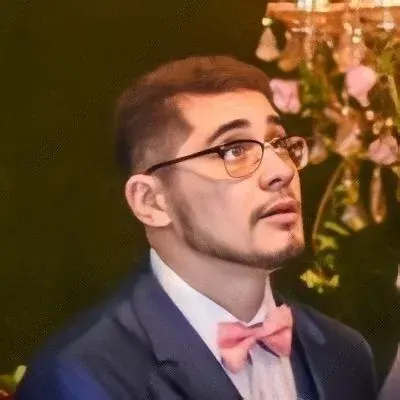
Title: 🤔 How to Change the Value Inside of a Textfield in Flutter
Introduction: Hey there flutteristas! Have you ever struggled with changing the text inside of a Textfield or TextFormField in Flutter? 🤔 Don't worry, we've got your back! In this blog post, we'll explore common issues, provide you with easy solutions, and show you how to become a master of updating text values. 💪 So let's dive right in!
Problem: 😫 Can't Change the Text Inside of a Textfield or TextFormField
The Scenario: Imagine this, you have a TextEditingController and you want to change the text inside a Textfield or TextFormField when a user clicks a button. You've tinkered around, but for some reason, the text remains stubbornly unaltered. It's like playing tug-of-war with your keyboard! 😩
Solution: ✔️ Updating the Value Inside the Textfield
Fear not, dear developer! Changing the text inside a Textfield in Flutter is as easy as pie 🥧. Sit back, relax, and let us guide you through two simple methods to tackle this challenge:
Method 1: Using a TextEditingController
Declare your TextEditingController. This controller will be your loyal sidekick in updating the text value. It's like having your very own personal assistant! 😎
TextEditingController _controller = TextEditingController();
Assign the controller to your Textfield or TextFormField. This forms an inseparable bond between the two, enabling you to manipulate the text inside. 🤝
TextFormField( controller: _controller, )
Update the text value within your button's onPressed method. Here's where the magic happens! Use the
text
property of your TextEditingController to change the text value. ✨ElevatedButton( onPressed: () { _controller.text = "New Text"; }, child: Text("Change Text"), )
Now, whenever the button is pressed, the text inside the Textfield will magically transform into "New Text". 🪄
Method 2: Using a GlobalKey
The GlobalKey method is an alternative approach to changing the text value inside a Textfield. 🗝️
Declare a GlobalKey for your Textfield or TextFormField. This unique key grants you access to the internals of the widget, allowing you to manipulate its properties directly. 🚪
GlobalKey<FormFieldState> _formKey = GlobalKey<FormFieldState>();
Assign the key to your Textfield or TextFormField. This creates a strong bond between the key and the widget, unleashing your power to control its destiny! 💥
TextFormField( key: _formKey, )
Update the text value within your button's onPressed method. This time, you'll utilize the
currentState
property of the GlobalKey to access the widget's state and change its text value. 🏋️♂️ElevatedButton( onPressed: () { var currentState = _formKey.currentState; if (currentState != null && currentState.validate()) { currentState.save(); // add your logic to update the text value here } }, child: Text("Change Text"), )
With this method, the text value inside the Textfield can be modified while also validating the field's input. How cool is that? 😎
Call-to-Action: 📢 Join the Flutter Textfield Masters!
Congratulations, you've conquered the challenge of changing the value inside a Textfield in Flutter! But don't stop here, master! There's still much more to explore on your Flutter journey. Join our community of Flutter Textfield Masters and unlock a whole new world of app development possibilities. 🚀
Share your own tips, tricks, and creative solutions to inspire others on their coding adventure. Let's build amazing Flutter apps together! 💙
So, what are you waiting for? Hit that "Share" button and let your fellow developers know about this handy guide. Together, we can conquer all Textfield-related hurdles, one Flutter at a time! 💪🚀