How do I disable a Button in Flutter?
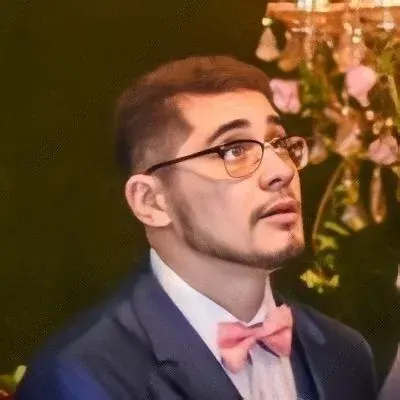
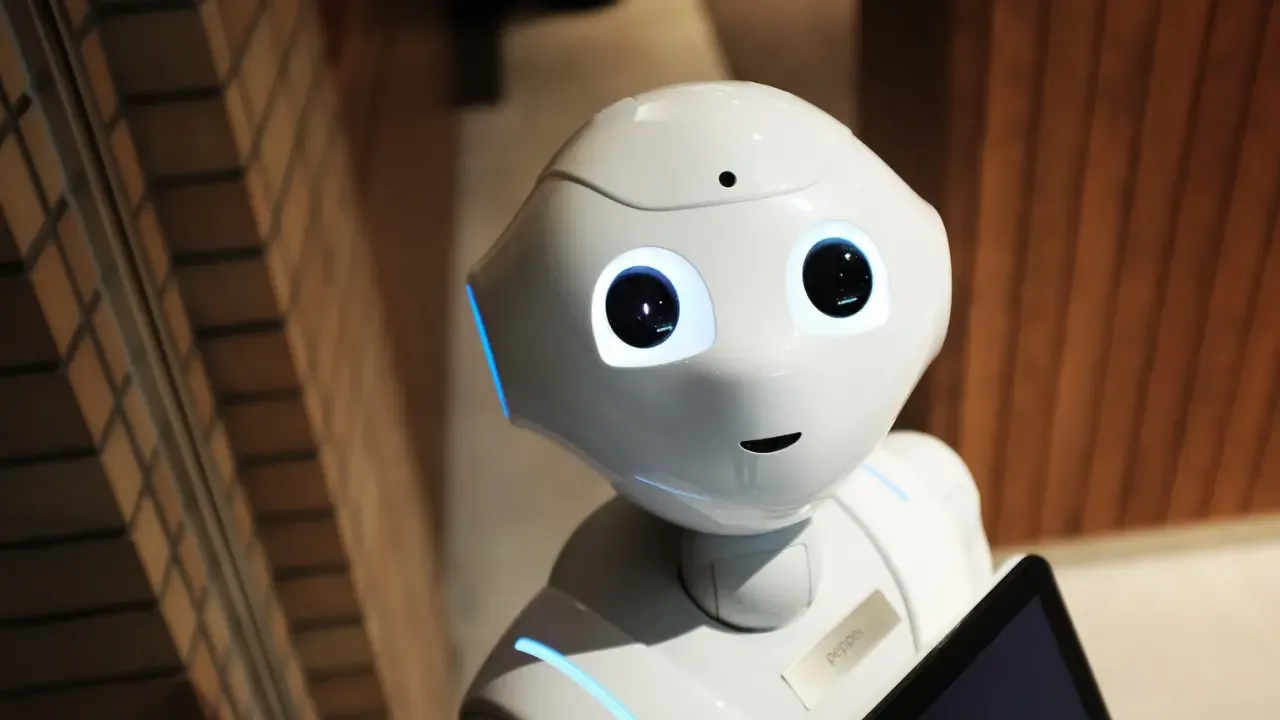
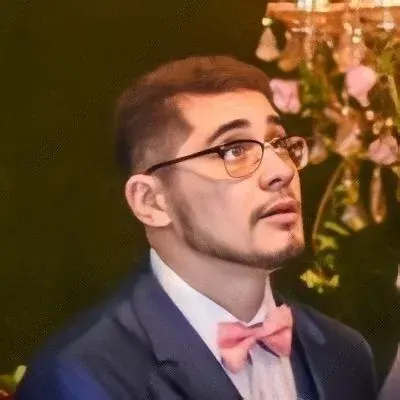
How to Disable a Button in Flutter: A Complete Guide
Are you a Flutter beginner who's struggling with setting the enabled state of a button? Don't worry, you're not alone! This common issue can be a bit tricky to figure out at first, but once you understand how it works, you'll be able to disable and enable buttons like a pro. 🚀
Understanding the basics
According to the Flutter documentation, to disable a button you need to set the onPressed
property to null
. Conversely, to enable it, you assign a callback function to onPressed
. This approach works well if the button's state remains the same throughout its lifecycle. But what if you need a button to dynamically change its enabled state? That's where a custom Stateful widget comes into play. 😉
Creating a custom Stateful widget
To achieve dynamic button enabling and disabling, you'll need to create a custom Stateful widget that allows you to update the button's enabled state or onPressed
callback.
Let's dive into a step-by-step example to better understand this process:
Start by creating a new Flutter project using your preferred IDE or the command line:
flutter create my_button_app
Next, open the
lib/main.dart
file and replace its content with the following code:
import 'package:flutter/material.dart';
class MyButton extends StatefulWidget {
@override
_MyButtonState createState() => _MyButtonState();
}
class _MyButtonState extends State<MyButton> {
bool _isEnabled = true;
void _toggleButtonState() {
setState(() {
_isEnabled = !_isEnabled;
});
}
@override
Widget build(BuildContext context) {
return MaterialApp(
home: Scaffold(
appBar: AppBar(
title: Text("Disable Button Example"),
),
body: Center(
child: Column(
mainAxisAlignment: MainAxisAlignment.center,
children: [
ElevatedButton(
onPressed: _isEnabled ? _toggleButtonState : null,
child: Text(_isEnabled ? "Enabled" : "Disabled"),
),
],
),
),
),
);
}
}
void main() {
runApp(MyButton());
}
Save the file and run the app using the following command:
flutter run
Congratulations! You've just created a basic Flutter app with a button that can be enabled and disabled.
Let's take a closer look at the code:
The
MyButton
class represents our custom Stateful widget.Within the
_MyButtonState
class, we have a boolean variable_isEnabled
to track the button's state.The
_toggleButtonState
function is responsible for toggling the button's state whenever it's pressed. It uses thesetState
method to notify Flutter that the UI needs to be updated.In the
build
method, we define a simple app structure with a centered column that contains our button.The
onPressed
property of theElevatedButton
widget is set to_isEnabled ? _toggleButtonState : null
, which means the button will be enabled if_isEnabled
is true and disabled if it's false.The button label dynamically changes between "Enabled" and "Disabled" based on the
_isEnabled
variable.
Take it to the next level
Now that you have a basic understanding of how to disable a button in Flutter, the possibilities are endless! You can customize the button's appearance, add additional logic based on different conditions, or even disable multiple buttons simultaneously.
Feel free to experiment with different Flutter widgets and explore the Flutter documentation for more advanced features. Happy coding! 🎉
Have you encountered any challenges while disabling a button in Flutter? How did you overcome them? Share your experience in the comments section below and let's help each other out! 😄