How can I dismiss the on screen keyboard?
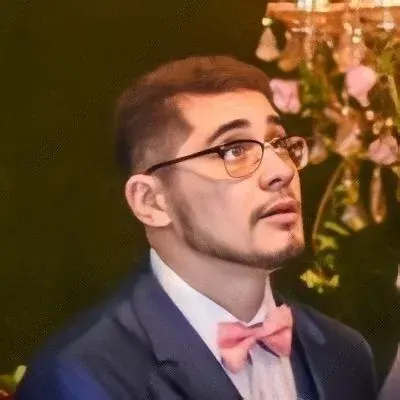
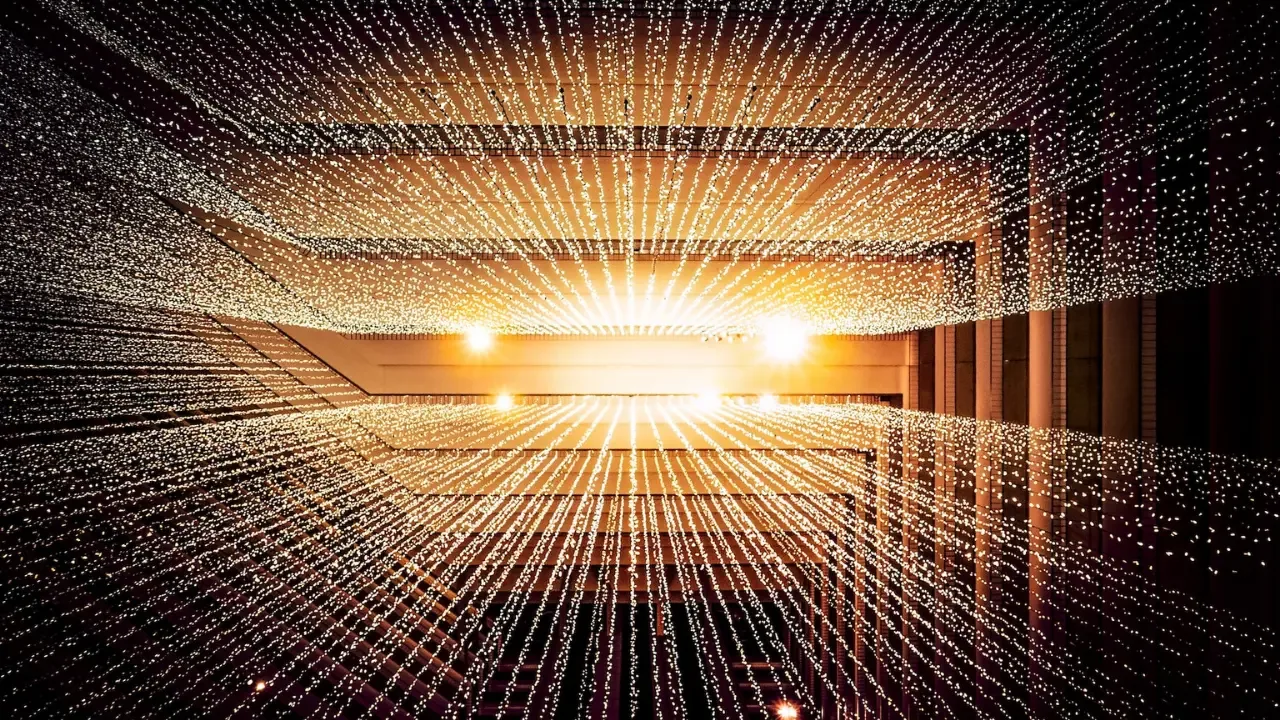
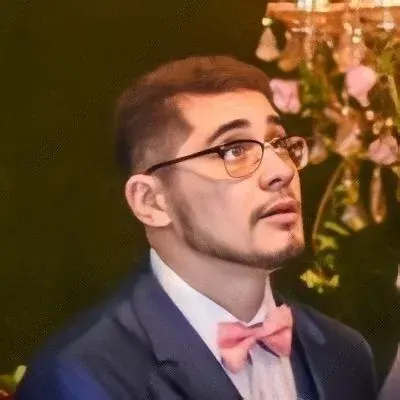
📝📲💻 Blog Post: How to Dismiss the On-Screen Keyboard in Flutter
Hey there, Flutter fans! Today, we're going to tackle a common issue many of you might have encountered while developing your Flutter apps - dismissing the on-screen keyboard. 😮
Picture this: you have a cool app that collects user input using a TextFormField widget, and when the user is done inputting their text, they tap a FloatingActionButton to indicate they're finished. But, uh-oh! The on-screen keyboard just stays there, obscuring the view and preventing a smooth user experience. 😫
Don't worry, though! We've got you covered with some easy-peasy solutions. Let's dive into the code and see how we can make the keyboard go away automatically. 🚀
import 'package:flutter/material.dart';
class MyHomePage extends StatefulWidget {
MyHomePageState createState() => new MyHomePageState();
}
class MyHomePageState extends State<MyHomePage> {
TextEditingController _controller = new TextEditingController();
@override
Widget build(BuildContext context) {
return new Scaffold(
appBar: new AppBar(),
floatingActionButton: new FloatingActionButton(
child: new Icon(Icons.send),
onPressed: () {
setState(() {
// send message
// dismiss on screen keyboard here
_controller.clear();
});
},
),
body: new Container(
alignment: FractionalOffset.center,
padding: new EdgeInsets.all(20.0),
child: new TextFormField(
controller: _controller,
decoration: new InputDecoration(labelText: 'Example Text'),
),
),
);
}
}
class MyApp extends StatelessWidget {
@override
Widget build(BuildContext context) {
return new MaterialApp(
home: new MyHomePage(),
);
}
}
void main() {
runApp(new MyApp());
}
🔎 So, how can we dismiss that pesky on-screen keyboard? Let's check out two viable solutions in Flutter:
Using the
FocusScope
class andunfocus
method:
import 'package:flutter/services.dart;
// Inside your function
FocusScope.of(context).unfocus();
With this approach, you call the unfocus()
method after the user taps the FloatingActionButton. This method will remove focus from any focused input fields and also dismiss the keyboard!
Utilize the
TextInputAction
and theonFieldSubmitted
property:
TextFormField(
controller: _controller,
decoration: new InputDecoration(labelText: 'Example Text'),
textInputAction: TextInputAction.send,
onFieldSubmitted: (_) {
// send message
// dismiss on screen keyboard here
_controller.clear();
},
),
By setting the textInputAction
property to TextInputAction.send
, you can trigger the keyboard's "send" button. Combine this with the onFieldSubmitted
property, where you can handle the text submission and dismiss the keyboard simultaneously.
💡 Remember to wrap your TextFormField with a Form
widget if you haven't already, as it allows for validation and easy access to the on-screen keyboard.
🎉 And there you have it, Flutter folks! Two simple ways to dismiss the on-screen keyboard in your Flutter app. Give them a try and let us know how it goes! If you have any other Flutter-related questions or tips, feel free to drop a comment below. We love hearing from our Flutter community! 😊
✨ Keep calm and Flutter on! ✨