How can I change the background color of Elevated Button in Flutter from function?
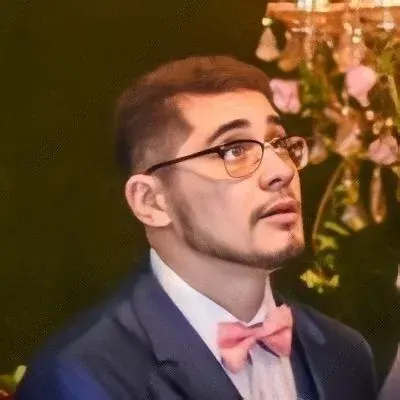
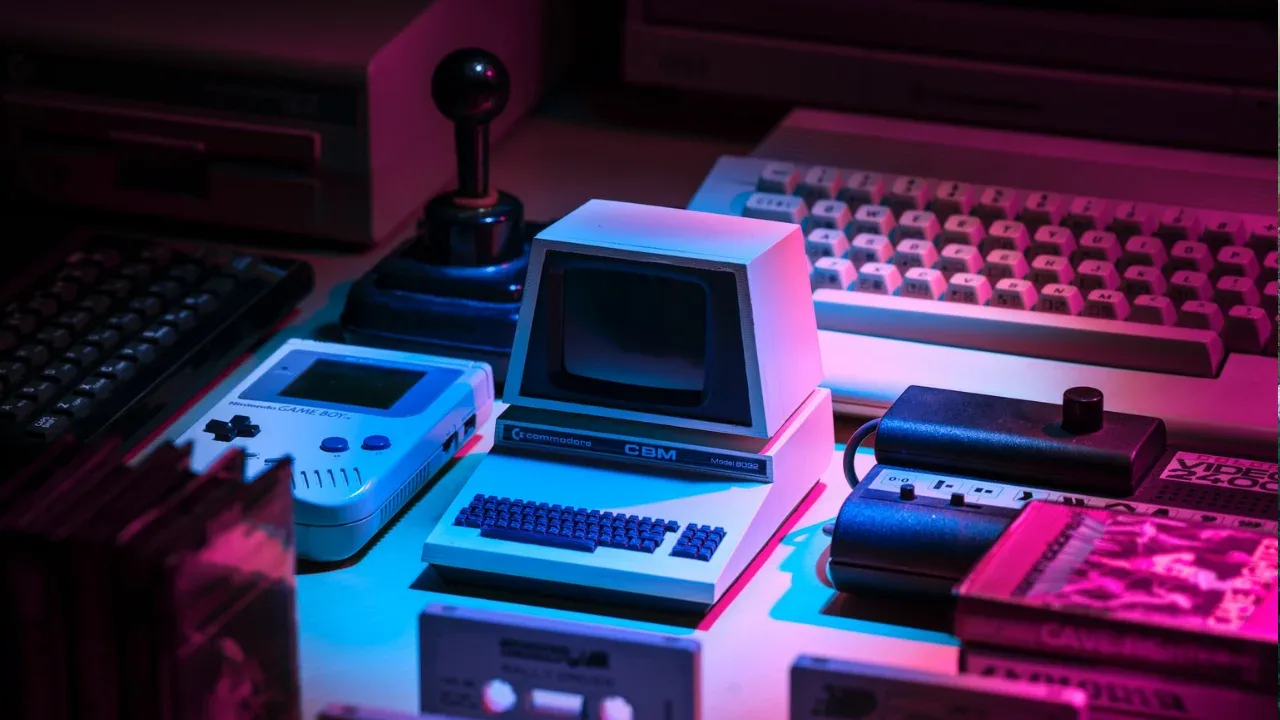
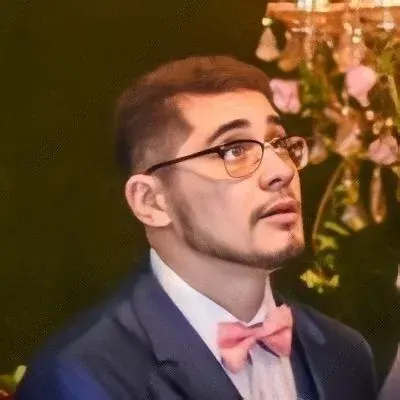
🎵 Change the background color of an Elevated Button in Flutter 🌈
Are you new to Flutter and looking to create a simple Xylophone application? You've come to the right place! In this guide, we'll show you how to change the background color of Elevated Buttons in Flutter and address the common issue you may encounter along the way.
The Error
Here's the error you might be facing when trying to call the playSound
function:
type '_MaterialStatePropertyAll' is not a subtype of type 'MaterialStateProperty<Color?>?'
The Code
First, let's take a look at the code for the playSound
function and the buildPlayButton
method, where the error is occurring:
void playSound(int soundNumber) {
final player = AudioCache();
player.play('note$soundNumber.wav');
}
Expanded buildPlayButton({MaterialStateProperty color, int soundNumber}) {
return Expanded(
child: ElevatedButton(
onPressed: () {
playSound(soundNumber);
},
style: ButtonStyle(
backgroundColor: color,
),
),
);
}
The Solution
To solve this error, you need to provide a valid Color
object instead of MaterialStateProperty
in the buildPlayButton
method. Here's how you can modify the code:
Expanded buildPlayButton({Color color, int soundNumber}) {
return Expanded(
child: ElevatedButton(
onPressed: () {
playSound(soundNumber);
},
style: ButtonStyle(
backgroundColor: MaterialStateProperty.all(color),
),
),
);
}
In the modified code, we replaced MaterialStateProperty
with Color
as a parameter for the buildPlayButton
method. Additionally, we wrapped the color
parameter with MaterialStateProperty.all
to create a valid MaterialStateProperty<Color?>
for the backgroundColor
property.
After making these changes, you can call the buildPlayButton
method with the desired color like this:
Widget build(BuildContext context) {
return Column(
crossAxisAlignment: CrossAxisAlignment.stretch,
children: [
buildPlayButton(color: Colors.red, soundNumber: 1),
buildPlayButton(color: Colors.orangeAccent, soundNumber: 2),
buildPlayButton(color: Colors.yellow, soundNumber: 3),
buildPlayButton(color: Colors.indigo, soundNumber: 4),
buildPlayButton(color: Colors.blue, soundNumber: 5),
buildPlayButton(color: Colors.lightGreenAccent, soundNumber: 6),
buildPlayButton(color: Colors.green, soundNumber: 7),
],
);
}
Take It to the Next Level! ✨
Now that you've successfully changed the background color of Elevated Buttons in Flutter, why not explore further? Experiment with different colors and styles to personalize your Xylophone application even more.
Share your creations with us and let your imagination run wild! 🎉
If you have any other questions or need additional assistance, feel free to leave a comment below. Happy coding! 💻