Force Flutter navigator to reload state when popping
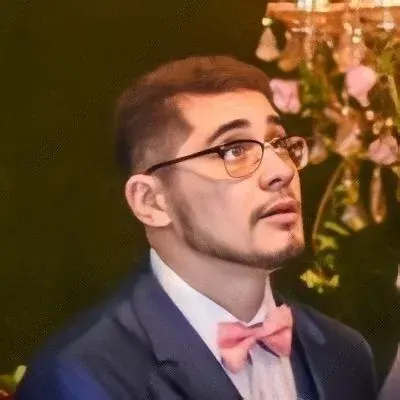
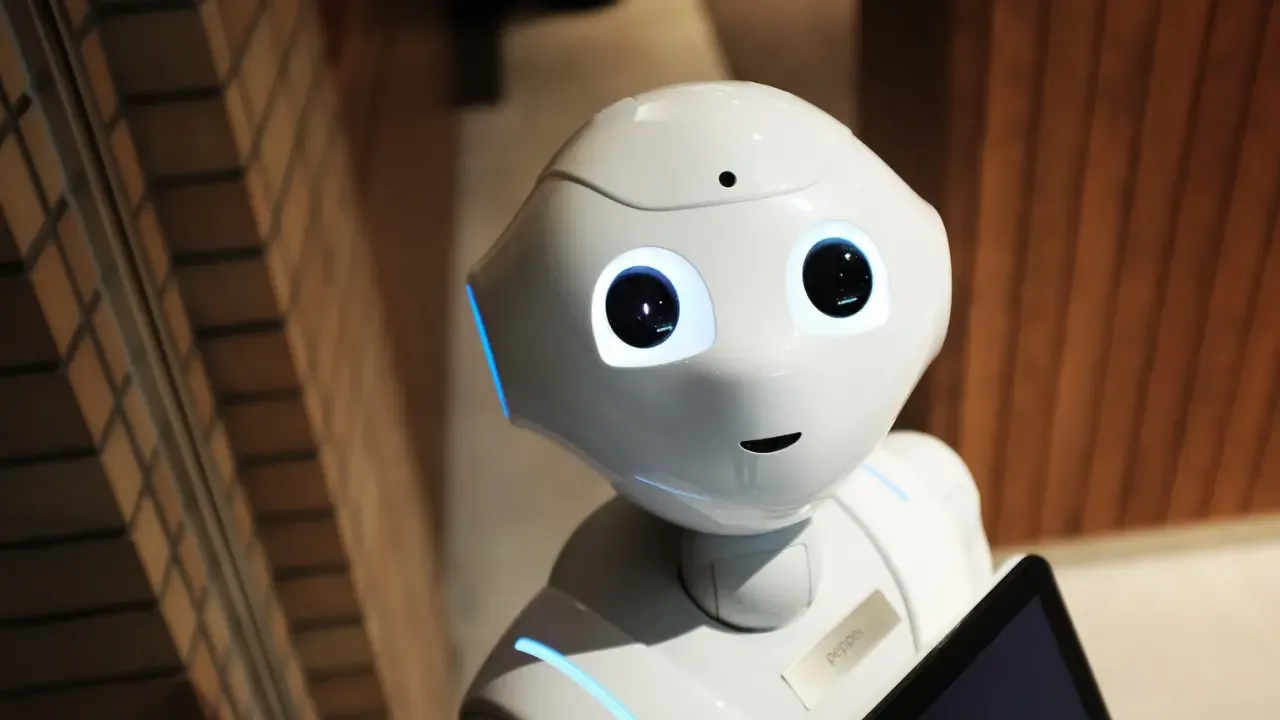
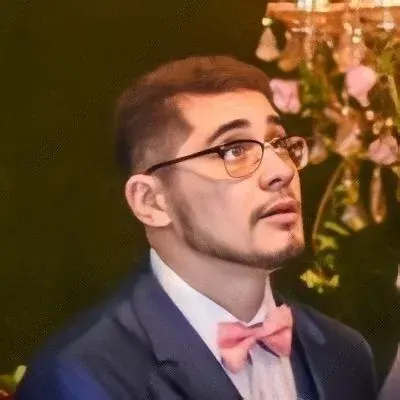
📝 How to Force Flutter Navigator to Reload State When Popping
Hey there, Flutter enthusiasts! 👋 Have you ever encountered an issue where you want to force the reload of a State when popping from a second widget to the first one? Don't worry, you're not alone! Many Flutter developers face this challenge, but we've got you covered with some easy solutions. Let's dive right in! 🚀
🔍 The Problem
Imagine you have a StatefulWidget with a button that navigates you to another StatefulWidget using Navigator.push()
. On the second widget, you make some changes to the global state, such as user preferences. However, when you go back to the first widget using Navigator.pop()
, it remains in the old state. This is not what you want, as you desire the first widget to be forcefully reloaded upon popping.
💡 The Ugly Solution
One possible solution, though far from ideal, is as follows:
Use
pop
to remove the second widget (current one).Use
pop
again to remove the first widget (previous one).Finally, push the first widget again, which should force a redraw.
However, this approach feels messy and is not recommended as it involves extra navigation steps.
✨ The Elegant Solutions
Let's explore two elegant solutions to force the reload of the first widget:
1️⃣ Using then
with push
The push
method can return a Future
that completes when the pushed route is popped. By utilizing then
, we can execute code after popping the route. Here's how you can do it:
Navigator.push(context, MaterialPageRoute(builder: (context) => SecondWidget()))
.then((value) {
setState(() {});
});
In the above example, we wrap the push
method with then
and call setState
to trigger a rebuild of the first widget when the second widget is popped.
2️⃣ Using didPop
with addPostFrameCallback
Another elegant solution involves using WidgetsBindingObserver
and didPop
callback in combination with addPostFrameCallback
. Let's take a look at the code:
class FirstWidget extends StatefulWidget {
@override
_FirstWidgetState createState() => _FirstWidgetState();
}
class _FirstWidgetState extends State<FirstWidget> with WidgetsBindingObserver {
@override
void initState() {
super.initState();
WidgetsBinding.instance!.addObserver(this);
}
@override
void dispose() {
WidgetsBinding.instance!.removeObserver(this);
super.dispose();
}
@override
void didPop() {
setState(() {});
super.didPop();
}
@override
Widget build(BuildContext context) {
// Widget build code here
}
}
In the above example, we include with WidgetsBindingObserver
in the state class and override didPop
to call setState
and trigger a rebuild when the second widget is popped. Additionally, make sure to add the observer in initState
and remove it in dispose
to avoid memory leaks.
📣 Wrapping Up
And there you have it! Two elegant solutions to force the reload of the first widget when popping from the second widget in Flutter Navigator. Say goodbye to the old state and welcome the fresher version of your widget! 🔄
Now it's your turn! Have you faced this issue before? Which solution worked best for you? Let us know in the comments below and keep the Flutter community engaged and thriving! 🌟
Until next time, happy coding! 😄✨