Flutter: Scrolling to a widget in ListView
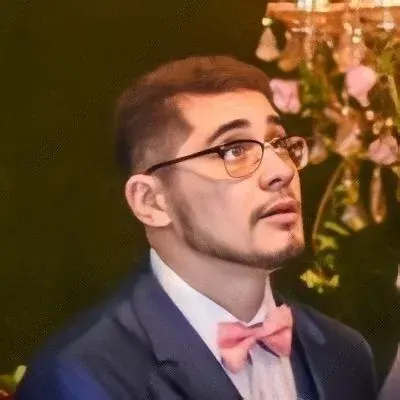
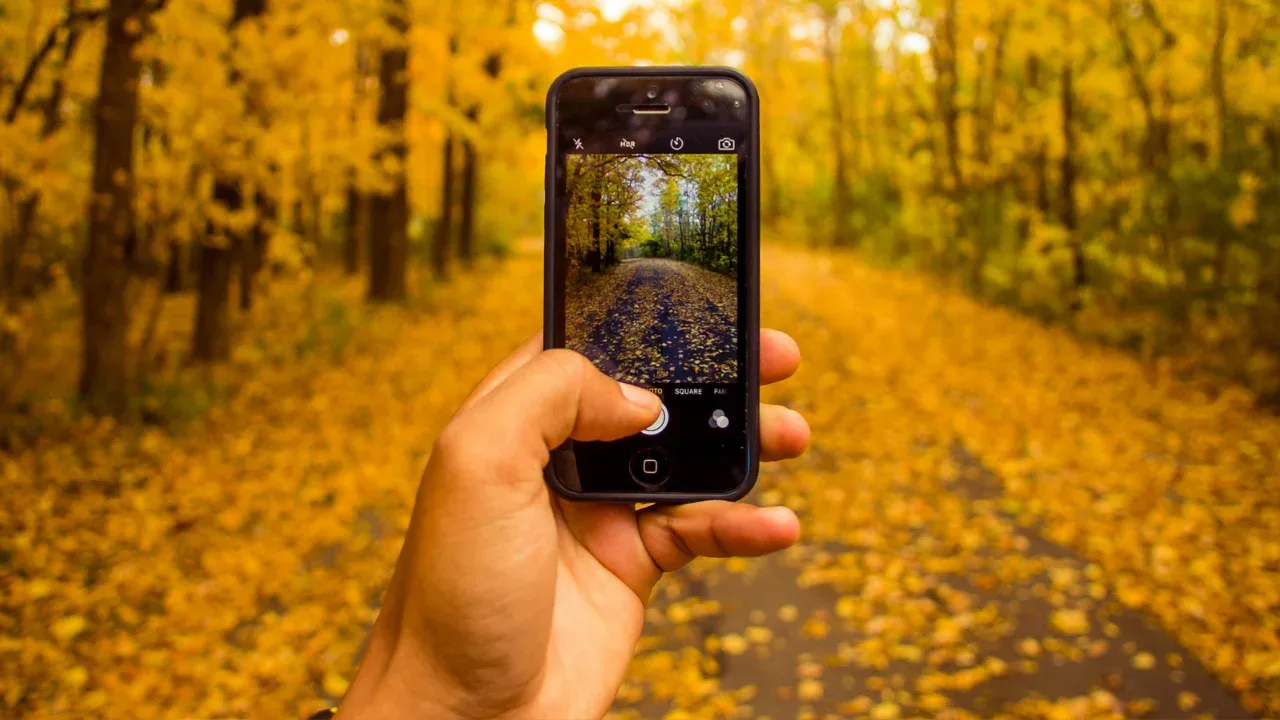
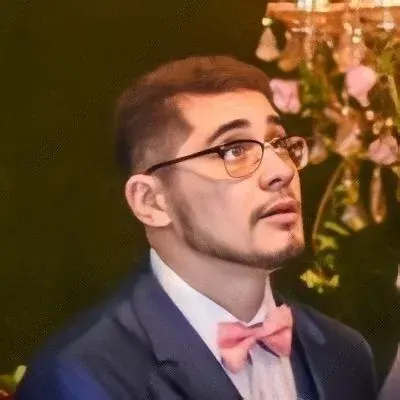
📝 Flutter: Scrolling to a Widget in ListView 📜
Have you ever wondered how to scroll automatically to a specific widget in a ListView? 🤔 Imagine you have a ListView with multiple containers, and you want to scroll to a particular container when a specific button is pressed. In this blog post, we'll explore common issues and provide easy solutions to help you achieve this scroll functionality effortlessly. So buckle up and let's dive right in! 🚀
The Problem 🧐
Many Flutter developers struggle to implement scrolling to a widget in a ListView when triggered by a button press. The challenge lies in identifying the target widget and scrolling to its position within the ListView. But worry not, we've got you covered with some simple and effective solutions! 💪
Solution 1: ScrollController to the Rescue 🏋️♀️
One way to achieve scrolling to a widget in a ListView is by using a ScrollController. The ScrollController class provides methods to control the scrolling behavior of a ListView. Here's how you can implement it:
// Create a ScrollController instance
final ScrollController _scrollController = ScrollController();
// Wrap your ListView with a ListView.builder
ListView.builder(
controller: _scrollController,
itemCount: yourContainerList.length,
itemBuilder: (BuildContext context, int index) {
return yourContainerList[index];
},
);
// Scroll to a specific container on button press
void scrollToContainer() {
_scrollController.animateTo(
yourContainerIndex * yourContainerHeight,
duration: Duration(milliseconds: 500),
curve: Curves.easeInOut,
);
}
In this solution, we create a ScrollController instance and assign it to the controller property of the ListView. Then, when the button is pressed, we use the animateTo method with the desired container's index and height to smoothly scroll to that widget. Remember to adjust the duration and curve based on your preference.
Solution 2: ListView.scrollTo or ListView.controller.scrollTo (Flutter 2.5+) 🔄
Starting from Flutter 2.5, the team introduced two new features to simplify scrolling to a specific widget in ListView.
ListView.scrollTo
: This method allows you to scroll to a specific item in the ListView directly.
myListView.scrollTo(
index: yourContainerIndex,
duration: Duration(milliseconds: 500),
curve: Curves.easeInOut,
);
ListView.controller.scrollTo
: If you prefer a more declarative approach, you can use the controller property of the ListView directly.
myListView.controller.scrollTo(
index: yourContainerIndex,
duration: Duration(milliseconds: 500),
curve: Curves.easeInOut,
);
These new methods provide a more concise and readable way to achieve the desired scroll behavior. Make sure you're using a version of Flutter that supports these features or upgrade your Flutter version accordingly.
Conclusion and Call to Action 🎉
Congratulations! 🥳 You've learned two easy solutions to scroll to a widget in a ListView. Whether you choose to use ScrollController or the new scrolling methods in Flutter 2.5+, now you can implement this functionality effortlessly in your projects. So don't be afraid to explore and experiment with these techniques.
If you found this blog post helpful, ❤️ share it with your fellow Flutter developers. Let's spread the knowledge and make scrolling in ListView a breeze! If you have any questions or suggestions, feel free to leave a comment below. Happy coding! 👨💻👩💻
PS: If you want to dive deeper into Flutter and learn more amazing tips and tricks, consider subscribing to our newsletter. Get ready for an exciting Flutter journey! 🌟
Keep Fluttering! ✨