Flutter align two items on extremes - one on the left and one on the right
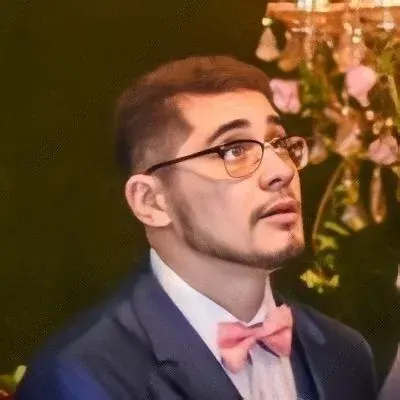
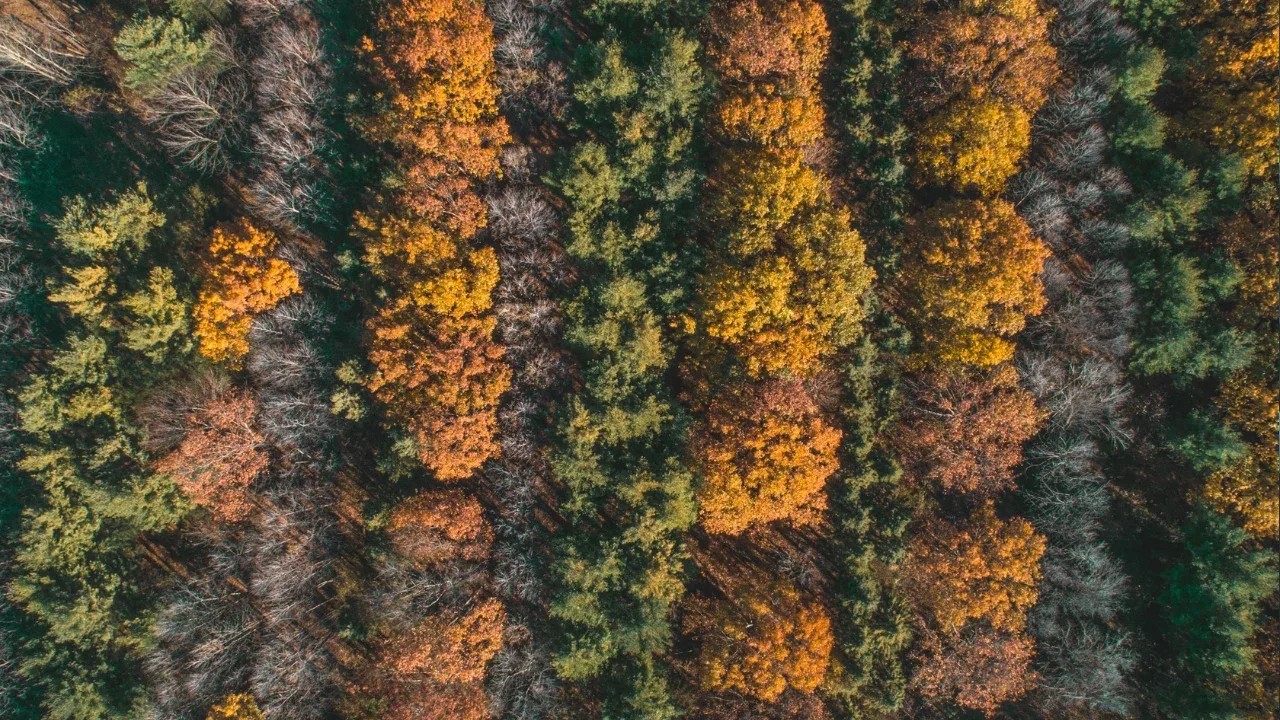
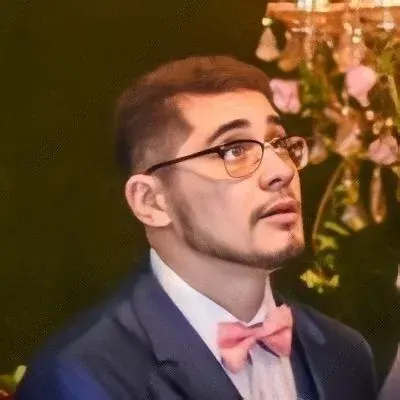
🎉 How to Align Two Items on Extremes in Flutter 🎉
Are you trying to align two items on opposite ends of a row in your Flutter app? 🤔 Don't worry, we've got you covered! In this blog post, we'll address the common issue of aligning items on the left and right extremes, provide easy solutions, and help you understand why your current code might not be working as expected.
🔍 Understanding the Problem
Let's start by taking a closer look at the code example you provided. It seems like you have a nameRow
consisting of two widgets: "Left" and SettingsRow
. The SettingsRow
widget is meant to be aligned to the right using MainAxisAlignment.end
, but it doesn't seem to work the way you intended. Here's why:
The alignment property of a child widget is influenced by its parent widget. In this case, the SettingsRow
is a child of nameRow
which has its mainAxisAlignment
set to MainAxisAlignment.start
. As a result, the child row inherits the alignment of its parent, overriding its own MainAxisAlignment.end
property.
💡 Simple Solutions
There are a couple of ways you can achieve the desired result. Let's explore two simple solutions:
Solution 1: Expanded Widget
Wrap the SettingsRow
widget with an Expanded
widget. This way, the SettingsRow
will take up the remaining space in the row, pushing "Left" to the left extreme and "Right" to the right extreme.
var nameRow = Row(
mainAxisAlignment: MainAxisAlignment.start,
crossAxisAlignment: CrossAxisAlignment.center,
mainAxisSize: MainAxisSize.max,
children: <Widget>[
Text("Left"),
Expanded(
child: SettingsRow,
),
],
);
Solution 2: Spacer Widget
Alternatively, you can use a Spacer
widget to create empty space between "Left" and "Right". The spacer will automatically fill the available space, pushing the items to their respective extremes.
var nameRow = Row(
mainAxisAlignment: MainAxisAlignment.start,
crossAxisAlignment: CrossAxisAlignment.center,
mainAxisSize: MainAxisSize.max,
children: <Widget>[
Text("Left"),
Spacer(),
Text("Right"),
],
);
🔑 Key Takeaways
When aligning items on opposite ends in Flutter, make sure to consider the relationship between parent and child widgets. If the parent widget's alignment conflicts with the desired alignment of a child widget, you may need to use an Expanded
or Spacer
widget to achieve the desired result.
📣 Your Turn to Engage!
We hope this guide helped you align those items just the way you wanted! 🎉 Now it's your turn to step up and share your experience. Have you encountered any alignment challenges in Flutter? What solutions have worked for you? Let us know in the comments below! 👇
Tags: #flutter #alignment #widgets #coding