Check whether there is an Internet connection available on Flutter app
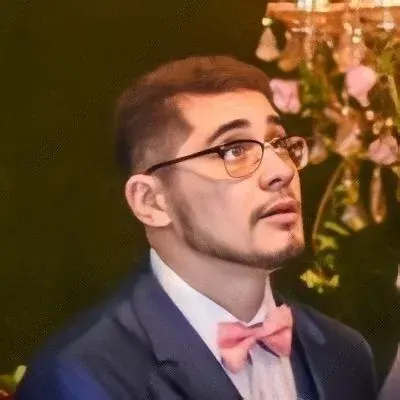
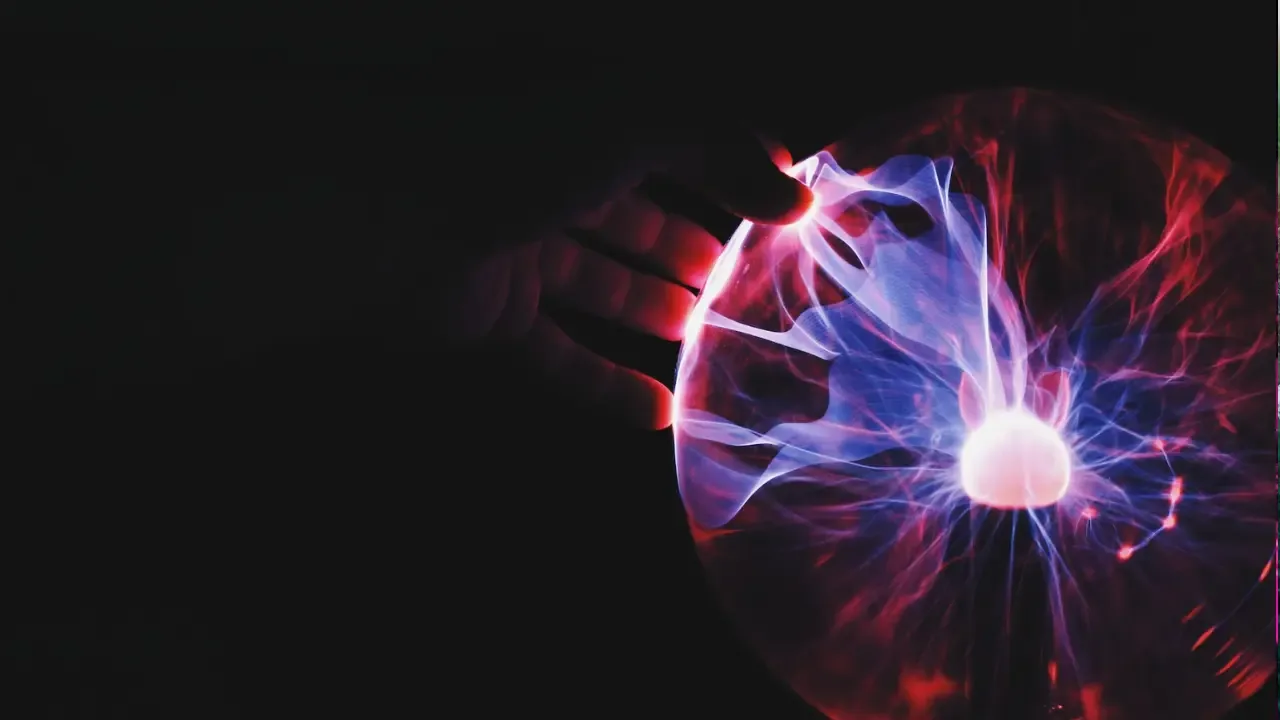
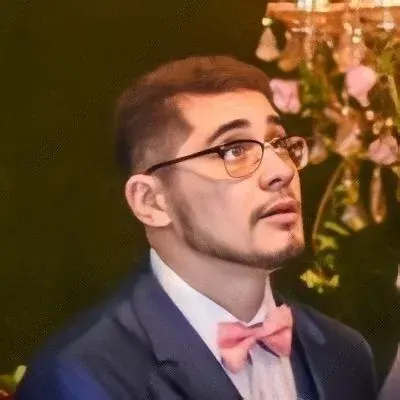
🌐 Is Your Flutter App Connected to the Internet? 📶
So, you're trying to execute a network call in your Flutter app, but before you do that, you want to confirm whether the device has an active internet connection. Makes sense! 😄
You've already attempted a solution, but unfortunately, it's not working as expected. Don't worry, we've got your back! In this blog post, we're going to address some common issues and provide easy solutions for checking internet connectivity in your Flutter app.
The Common Problem(s)
Before we dive into the solution, let's understand the problem you're facing. In your code snippet, you're using the checkConnectivity()
method from a user-defined class. However, it seems that this method isn't giving you the desired result.
So, what could be the issue here? Well, one possibility is that the checkConnectivity()
method might not be implemented correctly. Another possibility is that some permissions or dependencies might be missing. Let's explore some solutions to these problems!
Solution 1: Implementing the connectivity
Package
To check internet connectivity in your Flutter app, you can utilize the connectivity
package. This package provides a simple way to access information about the user's internet connection status. Here's how you can implement it:
Add the
connectivity
package to yourpubspec.yaml
file:
dependencies:
connectivity: ^3.0.6 # replace with the latest version of the package
Run the following command to fetch and install the package:
flutter pub get
Import the
connectivity
package in your Dart file:
import 'package:connectivity/connectivity.dart';
Check the internet connectivity using the
connectivity
package:
var connectivityResult = await (Connectivity().checkConnectivity());
if (connectivityResult == ConnectivityResult.mobile || connectivityResult == ConnectivityResult.wifi) {
// Yay! You have an internet connection. Proceed with your network call.
this.getData();
} else {
// Oops! No internet connection. Handle this scenario as per your app's requirements.
neverSatisfied();
}
By following these steps, you should be able to properly check for internet connectivity in your Flutter app using the connectivity
package.
Solution 2: Handling Missing Permissions or Dependencies
If the previous solution didn't work, another potential issue could be missing permissions or dependencies. To make sure your app can access the internet, you can add the necessary permissions and dependencies.
Permissions:
Android: Open the
AndroidManifest.xml
file located in theandroid/app/src/main
directory of your Flutter project. Add the following permission if it's not already present:<uses-permission android:name="android.permission.ACCESS_NETWORK_STATE" />
iOS: Open the
Info.plist
file located in theios/Runner
directory of your Flutter project. Add the following key-value pair if it's not already present:<key>NSAllowsArbitraryLoads</key> <true/>
Dependencies:
Android: Open the
build.gradle
file located in theandroid/app
directory of your project. Add the following dependency in thedependencies
block:implementation 'androidx.core:core:1.7.0'
iOS: No additional dependencies are required for iOS.
After adding the necessary permissions and dependencies, try running your app again and check if the internet connectivity issue is resolved.
Your Turn! 🚀
Now that you've learned some handy solutions for checking internet connectivity in your Flutter app, it's time to put them into action! Try implementing either Solution 1 or Solution 2, based on your specific situation.
Don't forget to share your thoughts and experiences in the comments section below! Have you encountered any other challenges related to internet connectivity in your Flutter app? We'd love to hear about it. Let's grow together as a Flutter community! 😊💙
Happy Fluttering!