Read/Parse text file line by line in VBA
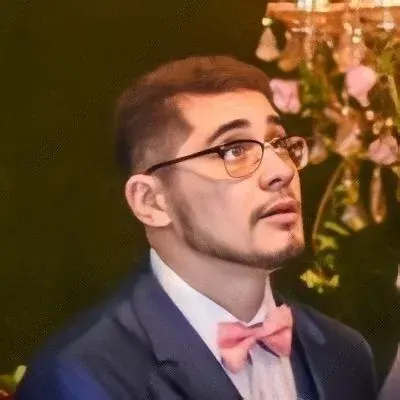
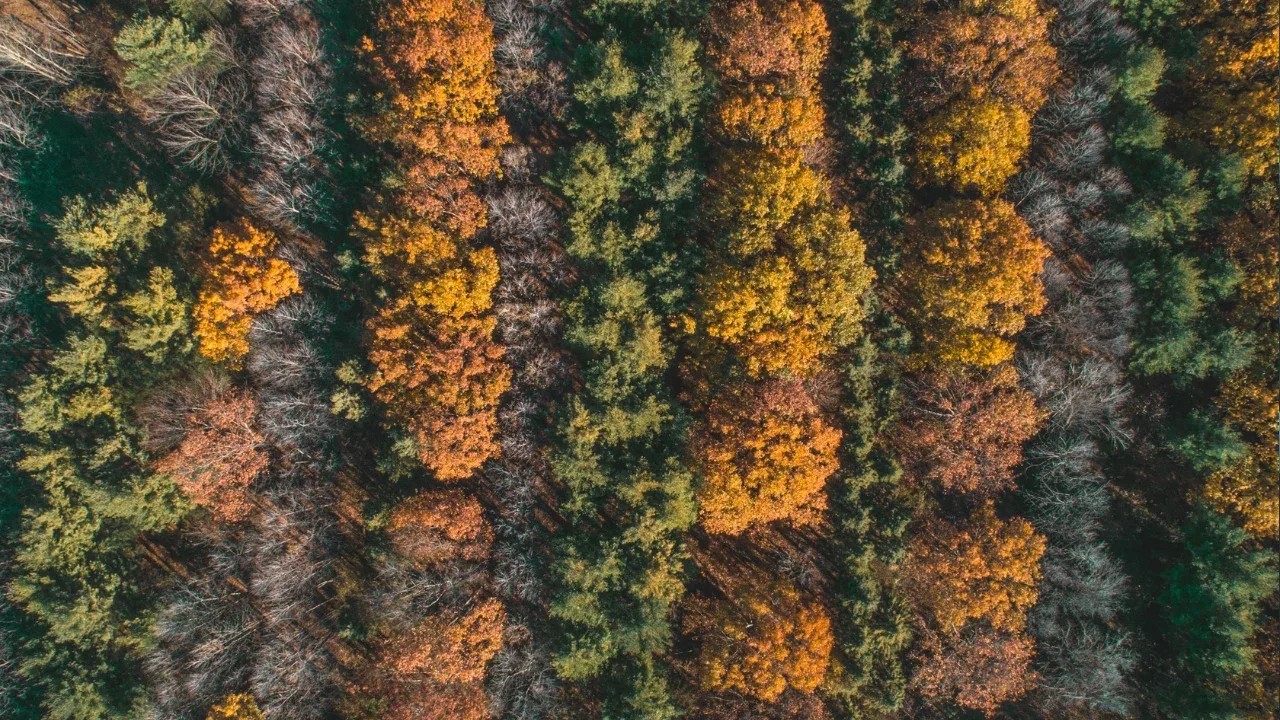
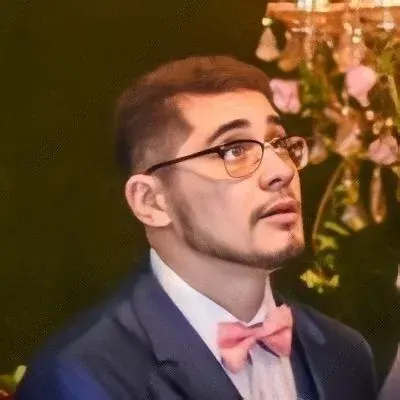
📝 Blog Post: Read/Parse Text File Line by Line in VBA
Are you struggling with parsing text documents using VBA? 🤔 Don't worry, we've got you covered! In this blog post, we'll walk you through the best and simplest way to read through a text file line by line using VBA and solve the specific problem of extracting file paths from the text file. Let's dive in! 💪
The Challenge: Parsing the Text File
Imagine you have a text file that contains instructions and file paths. You want to extract those file paths and write them to specific cells in an Excel spreadsheet.
*Blah blah instructions
*Blah blah instructions on line 2
G:\\Folder\...\data.xls
D:\\AnotherFolder\...\moredata.xls
Solution 1: Reading the Text File Using VBA
To read a text file using VBA, you can make use of the FileSystemObject
from the Microsoft Scripting Runtime library. Here's how you can do it:
Ensure that the Microsoft Scripting Runtime reference is enabled in your VBA project. You can do this by going to Tools > References and checking the box next to "Microsoft Scripting Runtime."
Declare a variable of type
Scripting.FileSystemObject
and create a new instance of it.
Dim fso As Scripting.FileSystemObject
Set fso = New Scripting.FileSystemObject
Use the
OpenTextFile
method of theFileSystemObject
to open the text file.
Dim file As Scripting.TextStream
Set file = fso.OpenTextFile("C:\path\to\your\text\file.txt")
Now, you can start reading the file line by line using the
ReadLine
method of theTextStream
object. For each line, you can perform your desired operations.
Do Until file.AtEndOfStream
Dim line As String
line = file.ReadLine
' Perform your operations with the line of text
' For example, check if it is a file path and write it to a cell
If Not line Like "*" Then
' Write the path to a cell, e.g., A2
Range("A2").Value = line
End If
' Move to the next line
Loop
Finally, remember to close the text file using the
Close
method.
file.Close
Solution 2: Parsing Line by Line
Now that you know how to read a text file, let's address how to parse it line by line. In the given context, we want to skip lines starting with a *
and extract the file paths. Here's a modified version of the code that does just that:
Do Until file.AtEndOfStream
Dim line As String
line = file.ReadLine
If Not line Like "*" Then
' Check if it is a file path and write it to the next available cell in row 2
For Each cell In Range("2:2")
If cell.Value = "" Then
cell.Value = line
Exit For
End If
Next cell
End If
' Move to the next line
Loop
Let's Recap and Take Action! ✅
In this blog post, we explored the best and simplest way to read through a text file line by line using VBA. We also solved a specific problem of extracting file paths and writing them to specific cells in an Excel spreadsheet.
Now it's your turn! Try implementing these solutions in your own VBA project and let us know how it goes. Do you have any other VBA challenges or questions? Share them with us in the comments below! We're here to help you. 💪💬
Happy coding! 🚀