How to Remove Line Break in String
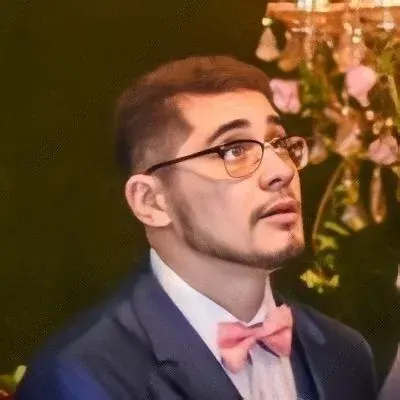
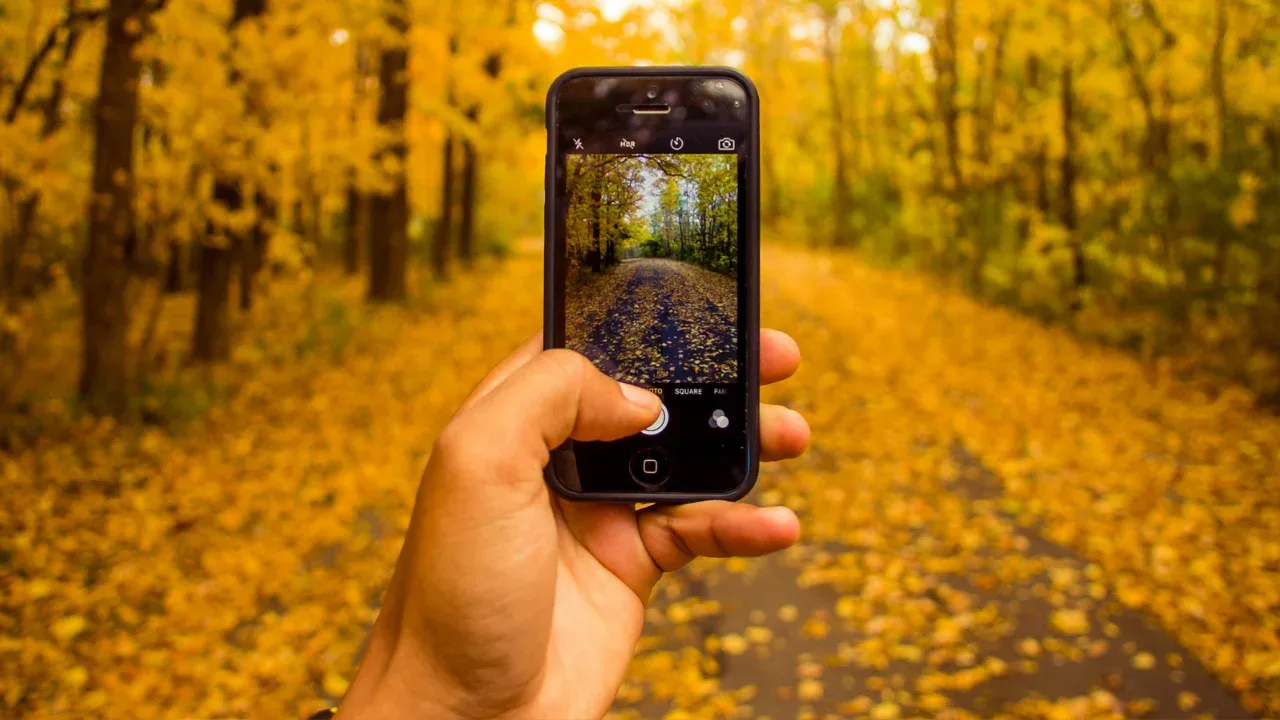
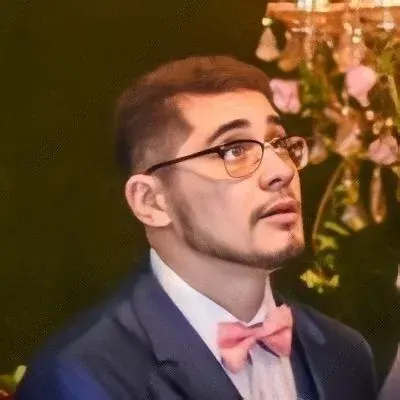
How to Remove Line Break in String
Have you ever encountered a frustrating situation where you want to remove a line break from a string, especially when the string ends with a line break? 🤔 We've got you covered with an easy solution! In this guide, we'll address this common issue and provide you with a simple approach to remove line breaks in a string. Let's dive in! 💪
The Problem: Removing Line Break From String
Imagine you have a string, and at the end of it, you have an annoying line break that you want to remove. This could cause issues when processing or displaying the string. It's a common scenario, and it can be quite cumbersome to handle. But fret not! We'll walk you through a straightforward solution that anyone can implement.
The Solution: Using VBA to Remove Line Break
If you're working with VBA (Visual Basic for Applications), here's a code snippet that demonstrates how to remove a line break if your string ends with one:
Sub linebreak(myString)
If Len(myString) <> 0 Then
If Right$(myString, 1) = vbCrLf Or Right$(myString, 1) = vbNewLine Then myString = Left$(myString, Len(myString) - 1)
End If
End Sub
Let's break down this code snippet to understand what's happening:
The
Len(myString) <> 0
condition ensures that the string is not empty, preventing any unnecessary processing.The
Right$(myString, 1) = vbCrLf Or Right$(myString, 1) = vbNewLine
condition checks if the last character of the string is either a carriage return followed by a line feed (vbCrLf
) or a single line feed (vbNewLine
).If the condition is true, the
Left$(myString, Len(myString) - 1)
expression removes the last character from the string using theLeft$
function.
With this code snippet, you can easily remove line breaks from a string. Feel free to integrate it into your VBA project or adapt it to your specific needs.
Taking It Further: Exploring Other Programming Languages
If you're not working with VBA and find yourself in a similar situation in another programming language, don't worry! The concept of removing line breaks from a string is applicable across various languages. You can achieve the same result with some modifications.
For example, in JavaScript, you can use the replace
method with a regular expression to remove line breaks:
const myString = 'Hello, World!\n';
const updatedString = myString.replace(/[\r\n]+$/g, '');
In this JavaScript example, the regular expression /[\r\n]+$/g
matches one or more (+
) carriage returns or line feed characters at the end of the string ($
). The replace
method replaces this pattern with an empty string, effectively removing the line breaks.
Explore the documentation or search for code examples specific to your programming language of choice, and you'll likely find simple and effective ways to solve this problem!
Share Your Experience and Get Involved
We hope this guide helped you effectively remove line breaks from a string. Give it a try in your code, and let us know how it worked for you! Do you have any other tips or tricks for handling line breaks in strings? Share them in the comments below. We'd love to hear from you!
Remember, programming is all about learning and sharing knowledge. If you found this guide helpful, consider sharing it with your friends and colleagues. Together, we can empower more developers to solve these common challenges easily.
Stay tuned for more helpful guides on tech-related topics. Happy coding! 🚀