How to get the excel file name / path in VBA
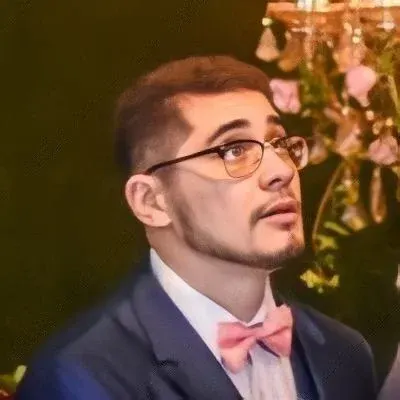

📊 How to Get the Excel File Name / Path in VBA
So, you're working on some fancy VBA magic inside your Excel file 📑 and you find yourself in need of the full path to that very file? Don't fret, my friend! We have a neat solution for you. 😉
The Scenario
Imagine you're working on a VBA project within your Excel file called 📙 sample.xls. You need to retrieve the complete path of the sample.xls file while running your VBA code. How do you make it happen? 🤔
The Solution
Fortunately, VBA provides a simple and effective solution to this problem. You can use a built-in property called ThisWorkbook.Path
to get the full path of your Excel file. 🙌
Here's an example of how you can retrieve the file path using VBA:
Sub GetExcelFilePath()
Dim filePath As String
' Get the full path of the Excel file
filePath = ThisWorkbook.Path
' Display the file path
MsgBox "The file path is: " & filePath
End Sub
In this example, we declare a variable called filePath
as a string. Then, we use the ThisWorkbook.Path
property to assign the full path of the Excel file to the filePath
variable. Finally, we display the file path using a message box.
That's it! You can now access the full file path of your Excel file with ease. 😎
Common Issues
Issue 1: Referencing the Wrong Workbook
In some cases, you might find yourself referencing the wrong workbook when trying to retrieve the file path. This can happen if you have multiple Excel files open or if your VBA code is stored in a different file.
To ensure you're referencing the correct workbook, use the ThisWorkbook
keyword, which refers to the workbook containing the VBA code.
Issue 2: Saving the Excel File Before Retrieving the Path
If you haven't saved your Excel file before running the VBA code, the ThisWorkbook.Path
property might return an empty string. Make sure to save your file before attempting to retrieve the file path.
Conclusion
Getting the full path of your Excel file in VBA is a breeze with the ThisWorkbook.Path
property! 🌈 Remember to be cautious when dealing with multiple workbooks and to save your file before running the code.
Now it's your turn! 🚀 Feel free to experiment with the provided solution and add your own creative touch to your VBA projects.
If you found this guide helpful, share it with your friends or fellow VBA enthusiasts. And don't hesitate to comment below if you have any questions or additional tips to share! 💬
Take Your Tech Career to the Next Level
Our application tracking tool helps you manage your job search effectively. Stay organized, track your progress, and land your dream tech job faster.
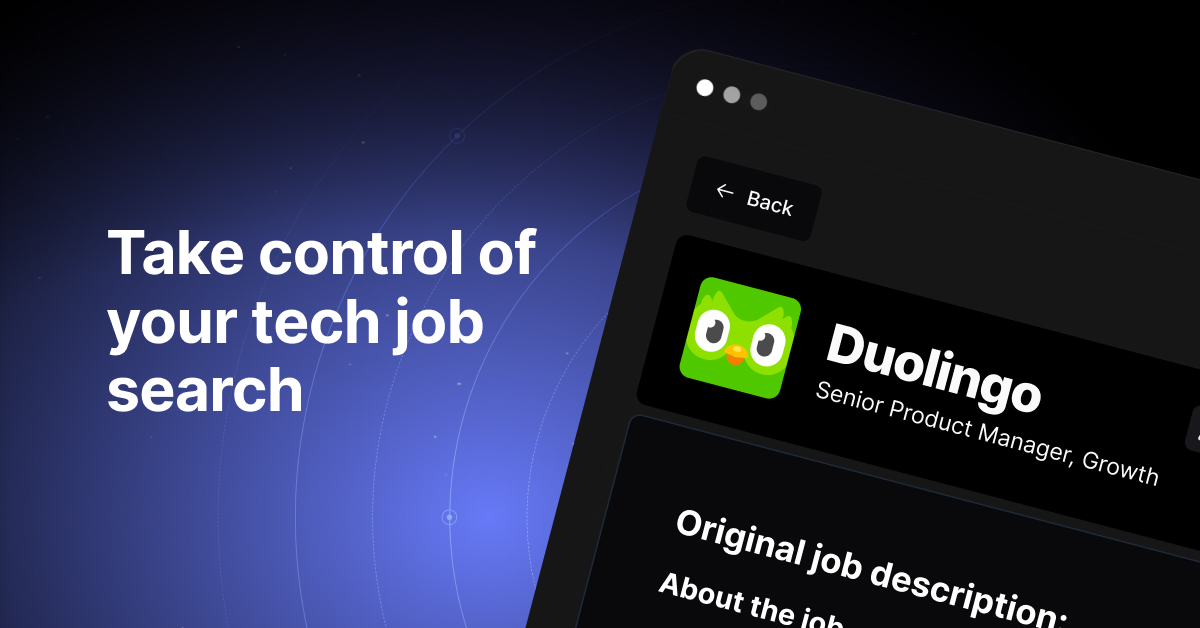