How should I make my VBA code compatible with 64-bit Windows?
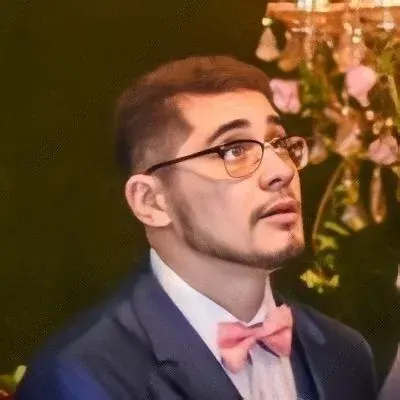
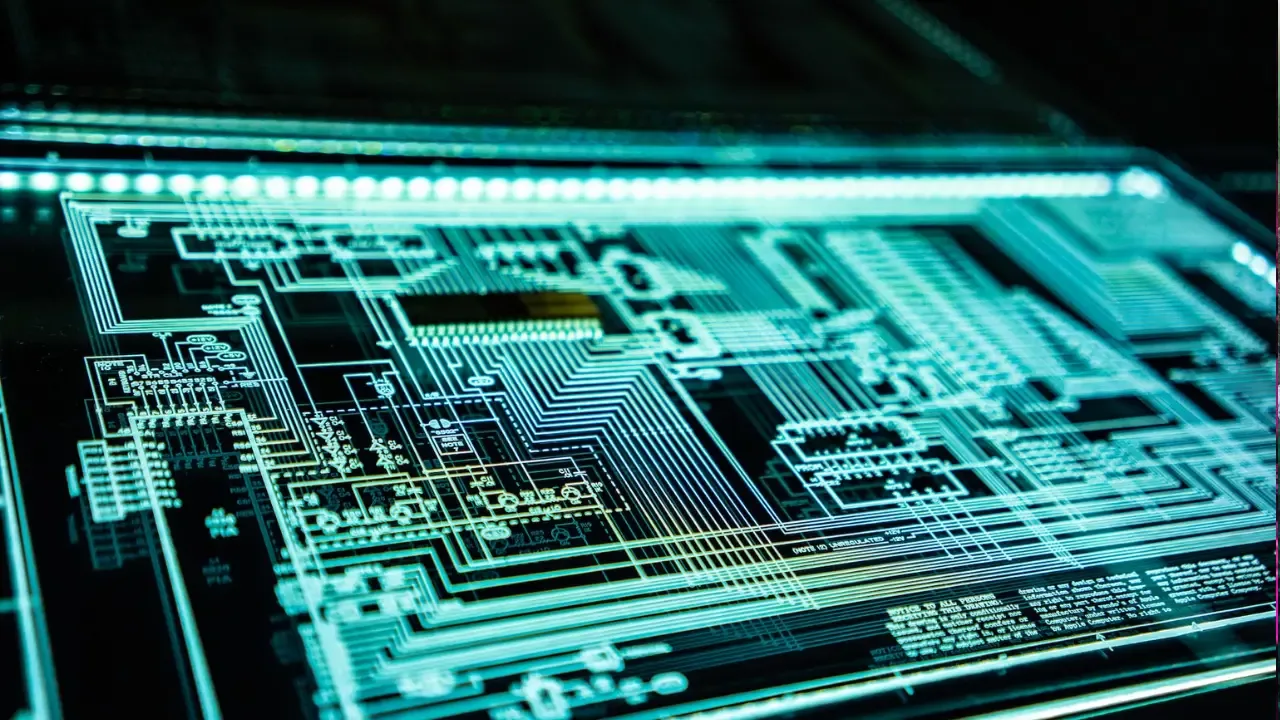
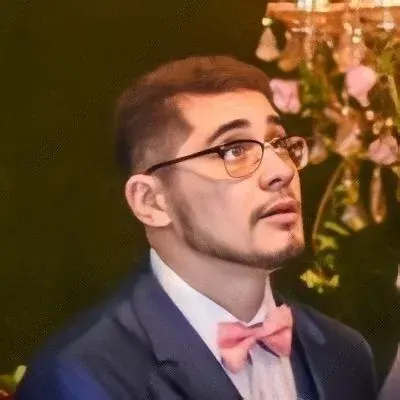
๐ Title: Making Your VBA Code Compatible with 64-bit Windows: Easy Solutions for Seamless Functionality!
๐ก Introduction Are you struggling to make your VBA code compatible with 64-bit Windows? You're not alone! Many developers face this challenge when working with VBA applications. But worry not, we've got you covered with easy solutions and alternative options that will ensure your code works seamlessly on any Windows platform. Let's dive in, shall we? ๐ช
๐ Understanding the Problem
The root of the problem lies in the compatibility issues between 32-bit and 64-bit systems, especially when working with VBA. In your case, you have an application developed in Excel 2007, and you're using the ShellExecute
function from Shell32.dll
with a Declare
statement. Your aim is to ensure this code works flawlessly on both 32-bit and 64-bit Windows systems.
๐ง Dispelling the Myth Before we get into the solutions, it's important to clarify a misconception. Your assumption that the code won't compile on a 64-bit version of Windows with 32-bit Office 2007 is not accurate! ๐ซ๐งข Thanks to David's answer, we now know that the code will work just fine as long as you're not using Office 2010 64-bit on Windows 64-bit. So, your Office 2007 environment is not holding you back. Let's explore the options!
๐ ๏ธ Solution 1: Conditional Compilation If you have a VBA application that needs to work on both 32-bit and 64-bit Windows systems, the best approach is to use conditional compilation. This technique allows you to have a single codebase while ensuring compatibility across different platforms. Here's how you do it:
Use the
#If
directive to check the VBA version and declare the function accordingly.#If VBA7 Then Private Declare PtrSafe Function ShellExecute Lib "shell32.dll" Alias "ShellExecuteA" (ByVal hwnd As LongPtr, ByVal lpOperation As String, ByVal lpFile As String, ByVal lpParameters As String, ByVal lpDirectory As String, ByVal nShowCmd As Long) As LongPtr #Else Private Declare Function ShellExecute Lib "shell32.dll" Alias "ShellExecuteA" (ByVal hwnd As Long, ByVal lpOperation As String, ByVal lpFile As String, ByVal lpParameters As String, ByVal lpDirectory As String, ByVal nShowCmd As Long) As Long #End If
In the example above, we use the
VBA7
constant to conditionally declare theShellExecute
function based on the VBA version being used. This ensures compatibility with both 32-bit and 64-bit Windows systems.Compile the code and distribute the application to your users. The conditional compilation will handle the appropriate declaration based on the user's system.
๐ ๏ธ Solution 2: Late Binding
If you want to avoid conditional compilation or have an older VBA version that doesn't support it, another option is to use late binding. This technique allows your code to interact with external libraries without relying on explicit Declare
statements. Here's how it works:
Remove the
Declare
statement for theShellExecute
function from your code.Use late binding to access the function dynamically at runtime.
Dim ShellExecute As Object Set ShellExecute = CreateObject("Shell.Application").ShellExecute
With late binding, you first create an object of the
Shell.Application
class, which provides access to various shell-related operations. Once you have the object, you can use theShellExecute
method to achieve the same functionality as before.Modify your code to use the late-bound
ShellExecute
object.Dim hwnd As Long Dim lpOperation As String Dim lpFile As String Dim lpParameters As String Dim lpDirectory As String Dim nShowCmd As Long hwnd = 0 ' Set the window handle as needed lpOperation = "open" lpFile = "C:\Path\To\File.docx" lpParameters = "" lpDirectory = "" nShowCmd = 1 ' Show the window normally ShellExecute.ShellExecute hwnd, lpOperation, lpFile, lpParameters, lpDirectory, nShowCmd
In this code snippet, we invoke the
ShellExecute
method on the late-boundShellExecute
object, passing the required parameters to perform the desired action.
๐ฃ A Call-to-Action for You! Congratulations! ๐ You're now equipped with two fantastic solutions to make your VBA code compatible with 64-bit Windows. Whether you choose conditional compilation or late binding, you can ensure your application works seamlessly across different platforms.
๐ก So, are you ready to level up your VBA skills and tackle compatibility challenges like a pro? Don't wait! Share your thoughts in the comments below and let us know which solution resonates with you. Have you encountered any other compatibility issues? We'd love to hear about them too. Happy coding! ๐๐ฉโ๐ป๐จโ๐ป
โก Disclaimer: The code snippets provided are for educational purposes only. Always test and validate code changes in your specific environment to ensure compatibility and stability.