How do I express "if value is not empty" in the VBA language?
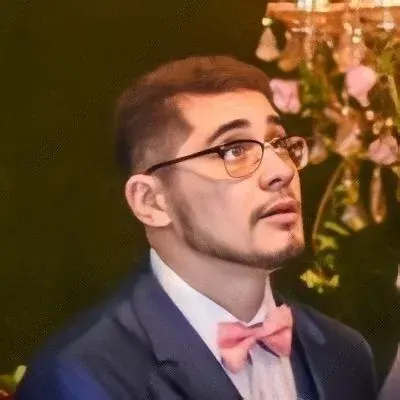
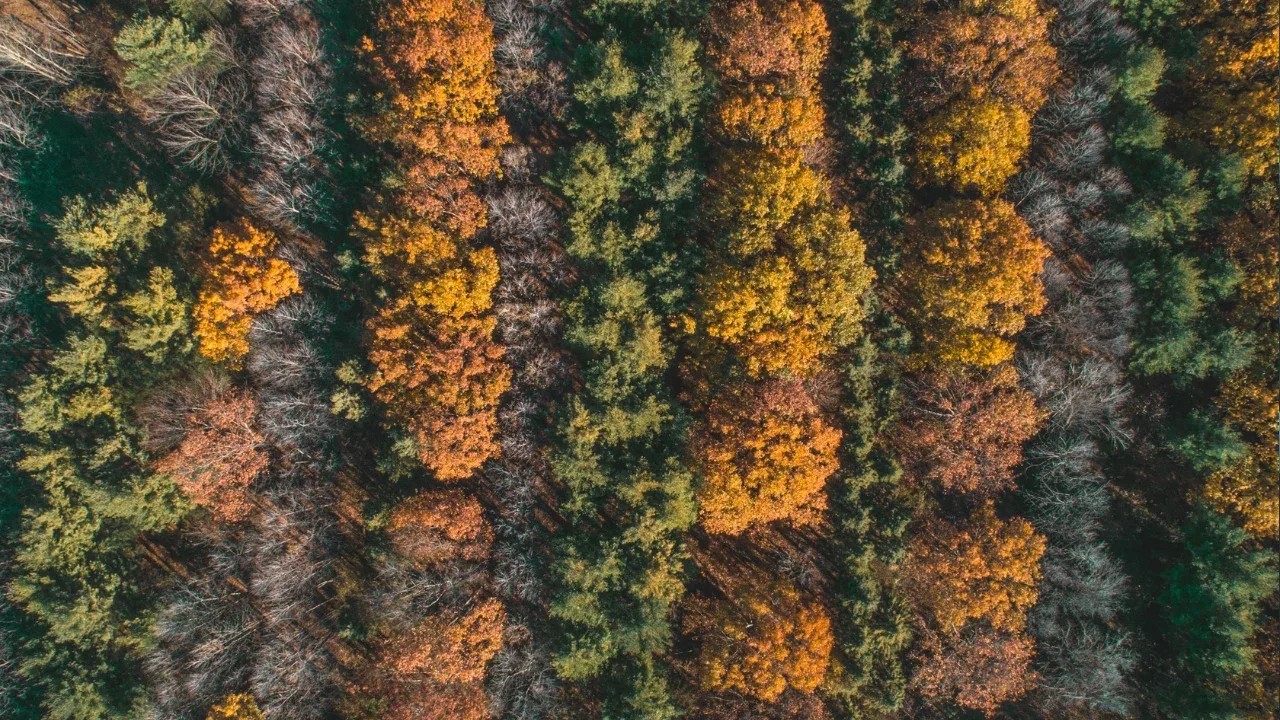
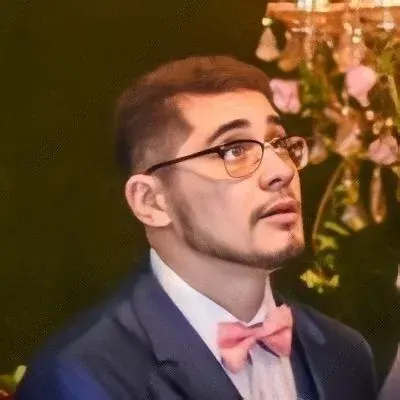
How to Check if a Value is Empty in VBA
Do you find yourself asking how to express the condition "if value is not empty" in the VBA language? You're in the right place! 🙌 In this blog post, we will guide you through the common issues and provide easy solutions to help you tackle this problem with confidence.
The Challenge of Checking for Empty Values
When programming in VBA, it's essential to handle empty values properly, as they can lead to unexpected behaviors or errors in your code. The question asks if the syntax if value is not empty then...
is correct. However, VBA uses a slightly different approach to check for empty values.
Using the IsEmpty Function
In VBA, you can use the IsEmpty
function to check if a value is empty. This function returns a Boolean value, True
if the value is empty and False
if it's not.
Here's an example:
Dim myValue As Variant
myValue = ""
If IsEmpty(myValue) Then
' Value is empty
' Your code here
Else
' Value is not empty
' Your code here
End If
In the example above, we declare a variable myValue
and assign an empty string ""
to it. We then use the IsEmpty
function to check if the value is empty. If it is, we execute the code inside the If
block. Otherwise, we execute the code inside the Else
block.
Be Aware of Variant Data Type
It's important to note that the IsEmpty
function works best with the Variant
data type. If you're dealing with other data types like String
, Integer
, or Double
, you might need to consider additional checks specific to those data types.
For example, if you want to check if a string value is empty, you should use the Len
function along with the Trim
function to account for whitespace:
Dim myString As String
myString = " "
If Len(Trim(myString)) = 0 Then
' String value is empty or contains only whitespace
' Your code here
Else
' String value is not empty
' Your code here
End If
Take Control of Your Code
Now that you have a clear understanding of how to check if a value is empty in VBA, you can confidently handle this common challenge in your code. Remember to choose the appropriate function (IsEmpty
, Len
, etc.) based on the data type you're working with.
If you found this guide helpful, why not share it with your fellow VBA enthusiasts? Sharing is caring! 😊
Do you have any other VBA-related questions or challenges? We'd love to hear from you! Leave us a comment below and let's continue the conversation.