Does the VBA "And" operator evaluate the second argument when the first is false?
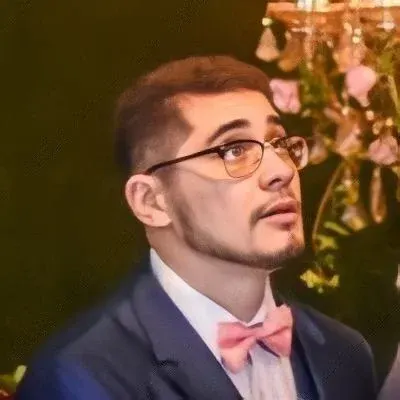

π VBA And Operator: Evaluating the Second Argument when the First is False?
Hey there, tech enthusiasts! π Let's dive into the mysterious world of VBA (Visual Basic for Applications) and explore whether the "And" operator evaluates the second argument even if the first one is false. π€
Recently, a fellow developer came across an intriguing situation with a VBA function. Let me share the context to give you a clearer picture. π§
Function Foo(thiscell As Range) As Boolean
Foo = thiscell.hasFormula And (InStr(1, UCase(Split(thiscell.formula, Chr(40))(0)), "bar") > 0)
End Function
This VBA function, aptly named "Foo," was designed to check if a particular substring (in this case, "bar") exists before the opening parentheses ((). Sounds simple, right? But here's where it gets interesting. π
The issue arises when an empty cell is passed into the function. In such cases, thiscell.hasFormula
evaluates to false. However, even with this first condition being false, the statement after the And
operator is still being evaluated. As a result, a pesky "subscript out of range" error occurs during runtime. π«
Now, let's get to the burning question: Does VBA really continue evaluating the second argument of the And operator, even when the first one is false? π€¨
The answer is YES! π± When using the And operator, VBA evaluates both the first and second arguments, regardless of the outcome of the first argument.
But fear not, my friends! π¦ΈββοΈ I'm here to provide easy solutions to mitigate this issue.
So what can you do to avoid encountering that nasty error?
Short-Circuit Evaluation: Instead of using the And operator, you can take advantage of short-circuit evaluation by using the
If...Then
statement. This way, the second argument will only be evaluated if the first one is true.
Function Foo(thiscell As Range) As Boolean
If thiscell.hasFormula Then
Foo = InStr(1, UCase(Split(thiscell.formula, Chr(40))(0)), "bar") > 0
Else
Foo = False
End If
End Function
Nested If Statement: Another approach is to use a nested If statement to check the conditions sequentially. This way, the second condition is only assessed if the first condition is true.
Function Foo(thiscell As Range) As Boolean
If thiscell.hasFormula Then
If InStr(1, UCase(Split(thiscell.formula, Chr(40))(0)), "bar") > 0 Then
Foo = True
Else
Foo = False
End If
Else
Foo = False
End If
End Function
These simple changes to the function allow you to avoid the dreaded subscript out of range error when dealing with empty cells. π
π Finally, I'd love to hear your thoughts on this topic! Share your experience or any alternative approaches you've used. Let's create a vibrant tech community! π¬π‘
Keep coding and stay curious! π
Take Your Tech Career to the Next Level
Our application tracking tool helps you manage your job search effectively. Stay organized, track your progress, and land your dream tech job faster.
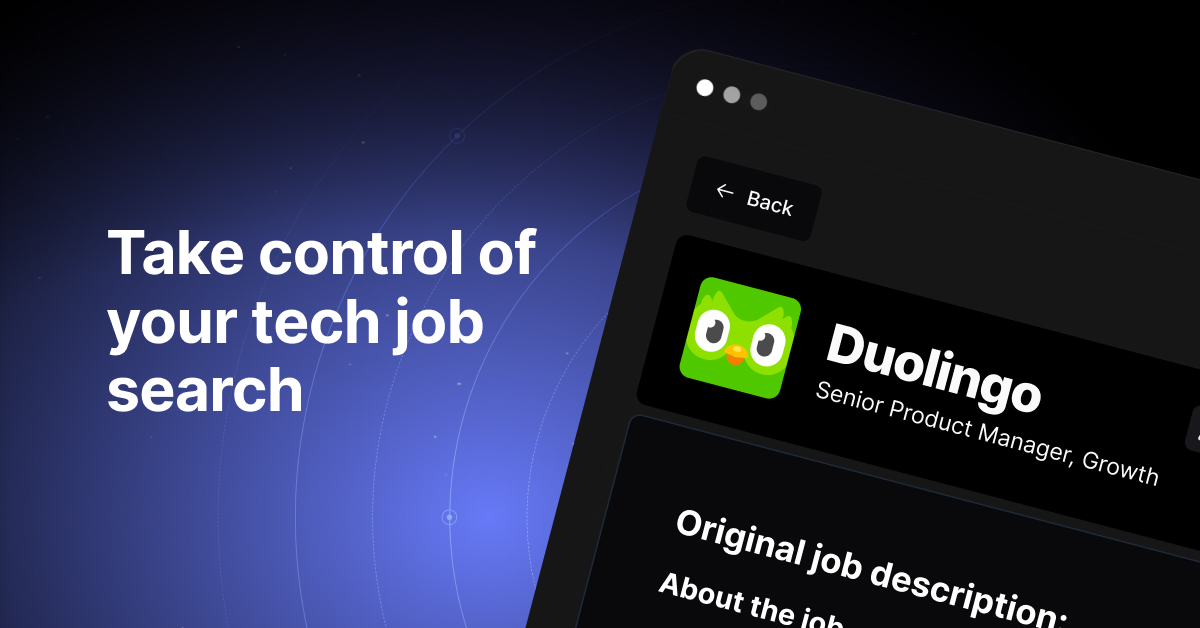