Create excel ranges using column numbers in vba?
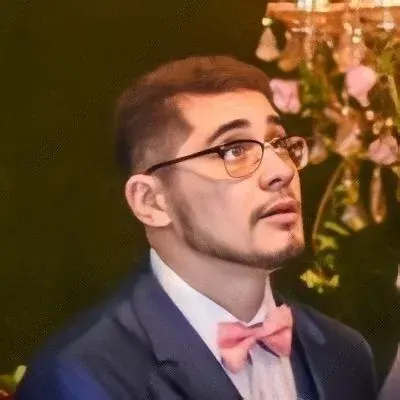
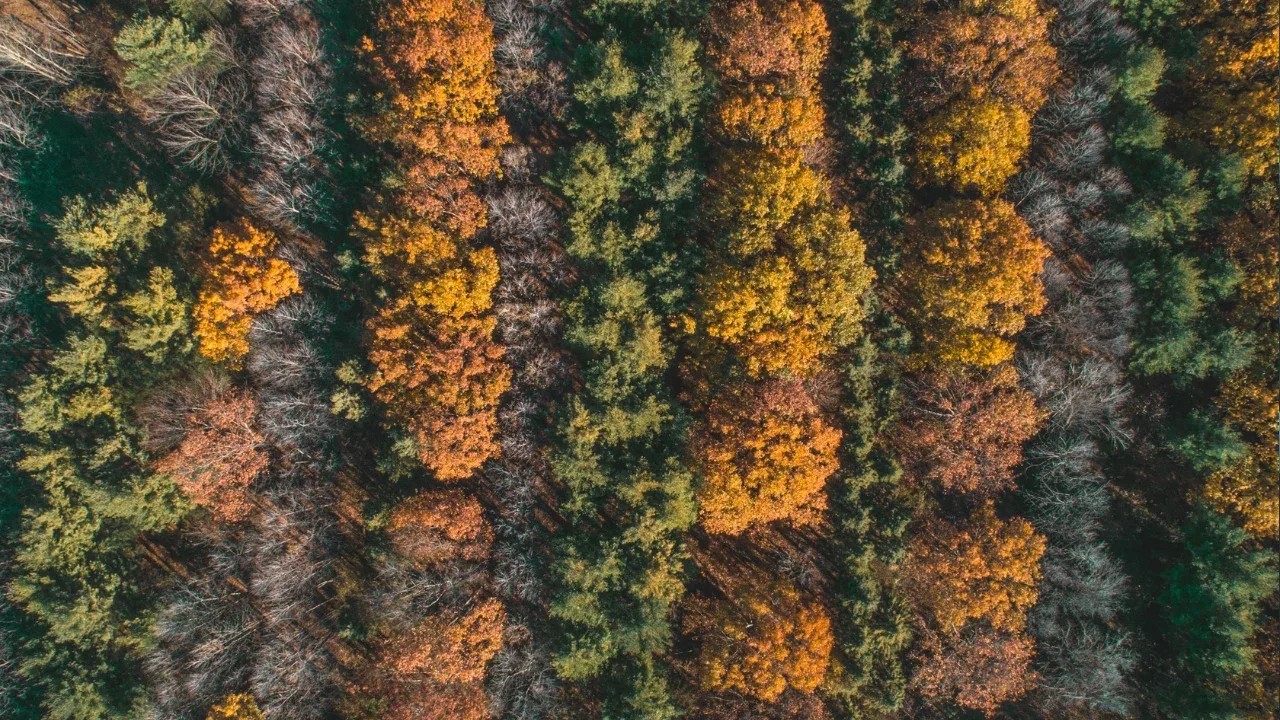
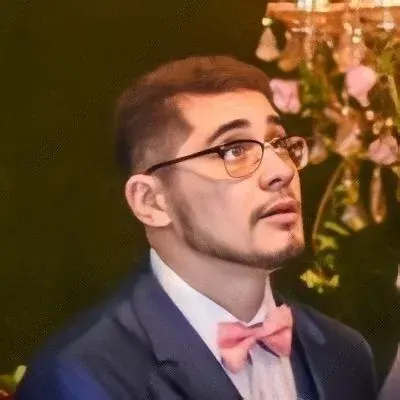
📊 Creating Excel Ranges Using Column Numbers in VBA
Have you ever found yourself struggling to create Excel ranges using column numbers instead of letters in VBA? It can be quite tricky, especially if you're used to working with column letters. But fear not! In this guide, we'll explore the common issues and provide easy solutions to help you conquer this challenge like a pro.
🔎 The Problem: Creating Ranges with Column Numbers
By default, Excel uses column letters (e.g., A, B, C) to identify columns. However, when working with VBA, it might be more convenient or necessary to use column numbers.
For instance, let's say you want to select all the cells in column 5 (which corresponds to column E). Without the right knowledge, you might find yourself scratching your head on how to achieve this.
💡 The Solution: Converting Column Numbers to Letters
The key to creating Excel ranges using column numbers is to convert these numbers to their corresponding letter representation. Fortunately, VBA provides a simple method to achieve this using the Chr
function.
Here's an example of how you can use this technique to select the range in column 5:
Dim columnNumber As Integer
Dim columnLetter As String
Dim targetRange As Range
columnNumber = 5
columnLetter = Chr(64 + columnNumber) ' Convert column number to letter
Set targetRange = Range(columnLetter & ":" & columnLetter) ' Create the range
' Now you can perform operations on the targetRange as needed
In this example, columnNumber
is set to 5, and Chr
is used to convert this number to the ASCII character code. By adding 64 to the column number, we get the corresponding ASCII code for the column letter. Finally, we create the range using the Range
method and the converted column letter.
🚀 Take It to the Next Level: Building Dynamic Range Functions
Now that you know how to create Excel ranges using column numbers, why not take it a step further and build your own dynamic range functions? These functions can be incredibly powerful and make your VBA code more modular.
For example, you can create a function that accepts the column number and the range's starting and ending row numbers as parameters. The function would then convert the column number to a letter and create the range accordingly.
Function CreateDynamicRange(columnNumber As Integer, startRow As Long, endRow As Long) As Range
Dim columnLetter As String
columnLetter = Chr(64 + columnNumber) ' Convert column number to letter
Set CreateDynamicRange = Range(columnLetter & startRow & ":" & columnLetter & endRow) ' Create the range
End Function
With this function, you can easily create dynamic ranges in your VBA code. Just pass in the desired column number and the starting and ending row numbers, and voila!
✨ Engage and Share Your Insights!
We hope this guide has helped you overcome the challenges of creating Excel ranges using column numbers in VBA. Now it's your turn to share your insights!
Have you encountered any other difficulties when working with Excel ranges in VBA?
Do you have any other tips or tricks to share on this topic?
Let us know in the comments section below! 📝
Remember, sharing is caring! If you found this guide helpful, don't forget to share it with your fellow VBA enthusiasts. Happy coding! 😉