What is reverse()?
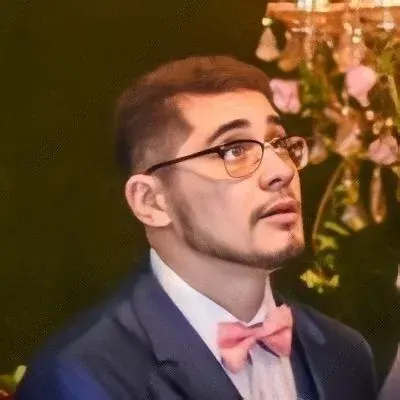
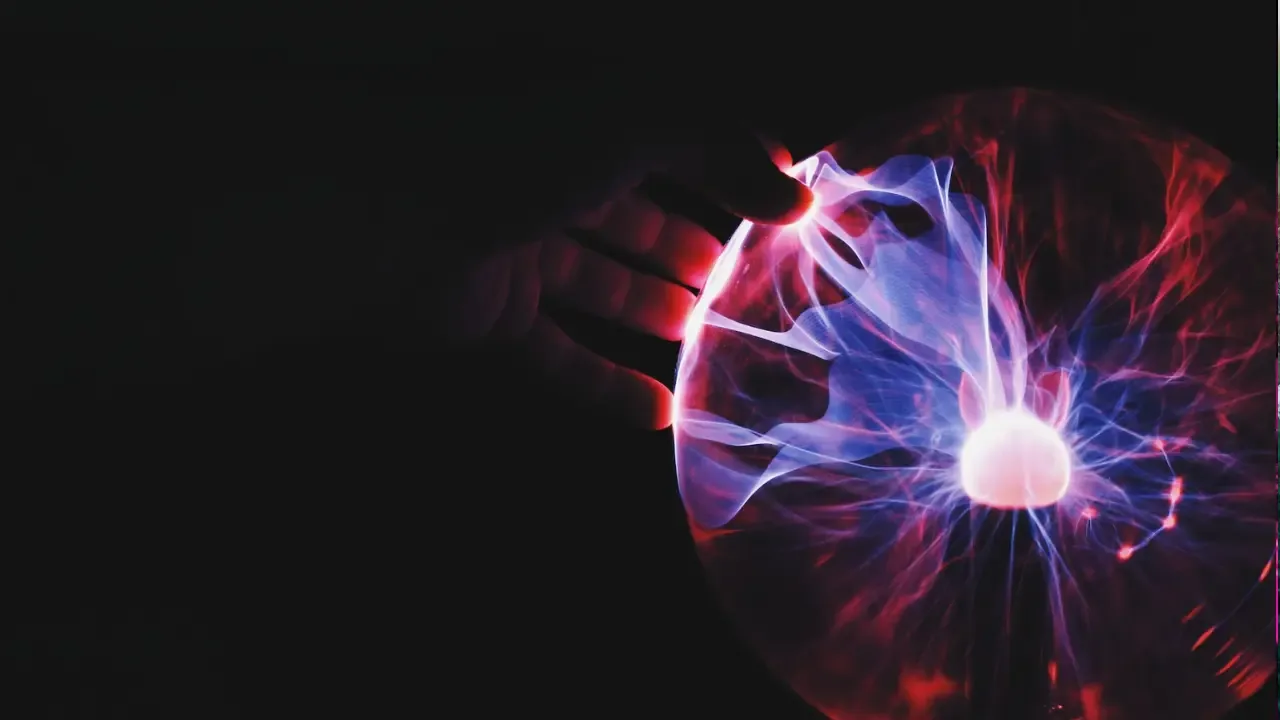
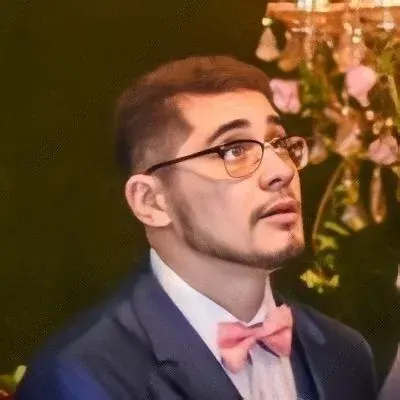
What is reverse() in Django?
If you've ever come across the mysterious reverse()
function while reading Django code, you're not alone. It can be a bit confusing at first, but fear not! In this blog post, we'll unravel the secrets of reverse()
and show you how and when to use it effectively.
Understanding the purpose of reverse()
reverse()
is a powerful function provided by Django that allows you to dynamically build URLs for your web application. It takes the name of a view as its argument and returns a URL pattern that corresponds to that view. This is particularly useful when you want to redirect users to a specific page after a certain action has been taken.
Common issues and how to solve them
Issue 1: Using reverse() without importing it
One common mistake that developers make when working with reverse()
is forgetting to import it. If you try to use reverse()
without importing it first, you'll likely encounter a NameError
telling you that reverse
is not defined.
Solution: To solve this issue, simply import reverse
at the top of your file. You can do this by adding the following line:
from django.urls import reverse
Issue 2: Providing the incorrect view name
Another issue that can arise is passing an incorrect view name to reverse()
. This can happen if you misspell the view name or if the view doesn't exist in your application.
Solution: To fix this issue, double-check the spelling of the view name and ensure that it exists in your project's URL configuration. If you're unsure about the correct view name, you can always refer to the URLs file in your Django project.
Issue 3: Forgetting to include app namespacing
If you're working with a Django application that uses app namespacing, you need to include the app name in the view name when using reverse()
. Failure to do so will result in an NoReverseMatch
error.
Solution: To resolve this issue, prepend the app name followed by a colon to the view name. For example, if your app name is myapp
and your view name is home
, you would use reverse('myapp:home')
.
A practical example
Let's say you have a web application where users can submit forms. After successfully submitting a form, you want to redirect them to a "success" page. Here's how you can achieve this using reverse()
:
from django.http import HttpResponseRedirect
from django.urls import reverse
def form_submission(request):
# ... form handling logic ...
# Redirect to the success page
return HttpResponseRedirect(reverse('success'))
In this example, the reverse()
function is used to dynamically build the URL for the success
view. The HttpResponseRedirect
class is then used to perform the actual redirect.
Take action and level up your Django skills!
Now that you have a better understanding of reverse()
in Django, it's time to put your newfound knowledge into practice. Start using reverse()
in your own projects and see how it can simplify your URL management.
Don't forget to share this blog post with your fellow Django developers so that they too can level up their skills and avoid common pitfalls with reverse()
.
Got any questions or other tips about reverse()
? Let us know in the comments below! 🎉