What does on_delete do on Django models?
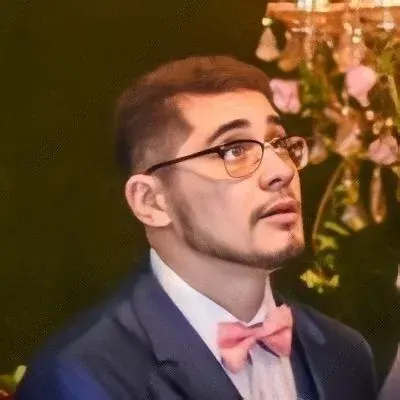
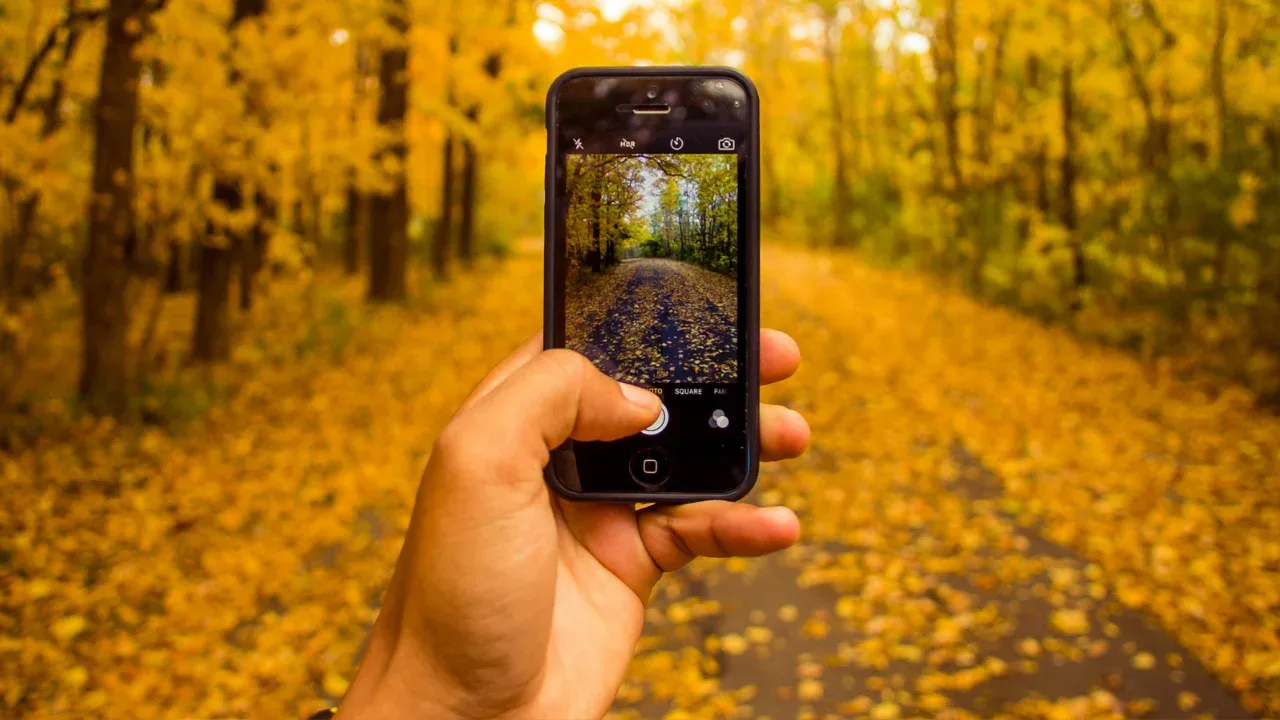
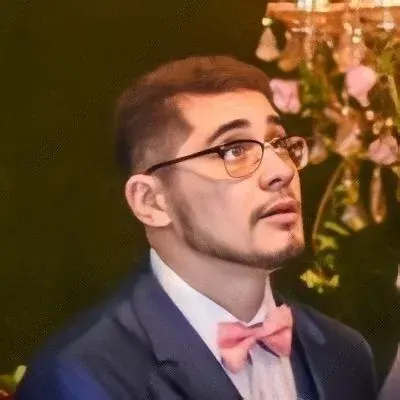
What does on_delete
do on Django models? 🤔
If you've been working with Django, you may have come across the on_delete
option in models. It's a bit mysterious, as the official documentation doesn't provide much detail. But worry not! We're here to break it down for you.
Understanding the on_delete
option ⚙️
The on_delete
option is used in Django models to specify the behavior when a related object (in this case, a foreign key) is deleted. It allows you to define what should happen to the objects that are linked to the deleted object.
🎯 Common issues and a simple solution
🌊 CASCADE: The most common option
The default value for on_delete
is models.CASCADE
. What does this mean? When you delete an object that has a foreign key relationship, all the objects linked to it will be deleted as well. Let's take a look at an example:
from django.db import models
class Car(models.Model):
manufacturer = models.ForeignKey(
'Manufacturer',
on_delete=models.CASCADE,
)
# ...
In this case, if you delete a Manufacturer
instance, all the associated Car
instances will also be deleted. This is the most common and straightforward behavior.
🌴 PROTECT: Prevent deletion
Another option you can use for on_delete
is models.PROTECT
. This option prevents you from deleting an object that has related objects. Django will raise an error and prevent the deletion. Here's an example:
class Manufacturer(models.Model):
# ...
class Car(models.Model):
manufacturer = models.ForeignKey(
Manufacturer,
on_delete=models.PROTECT,
)
# ...
Now, if you try to delete a Manufacturer
instance that has associated Car
instances, Django will raise an error to protect the integrity of your data. This can be useful when you want to enforce referential integrity.
🚪 SET_NULL: Set foreign key to null
The models.SET_NULL
option can be handy when you want to retain the related objects but remove the association. When you set on_delete=models.SET_NULL
, Django will set the foreign key to NULL
for all the related objects. Here's an example:
class Manufacturer(models.Model):
# ...
class Car(models.Model):
manufacturer = models.ForeignKey(
Manufacturer,
on_delete=models.SET_NULL,
null=True,
)
# ...
With this configuration, if you delete a Manufacturer
instance, the manufacturer
attribute in all the associated Car
instances will be set to NULL
.
📚 Other options and where to find documentation
Apart from the options we mentioned, Django provides other options for the on_delete
parameter, such as models.SET_DEFAULT
and models.SET()
.
To find detailed documentation on the on_delete
behavior and all the available options, check out the official Django documentation on ForeignKey. Although the information may not be as extensive as you'd like, it covers all the essential details.
Let's wrap it up!
Now you have a better understanding of what on_delete
does in Django models. Remember, it allows you to define the behavior when a related object is deleted. You can choose to cascade the deletion, protect it, set it to NULL
, or use other available options.
Next time you encounter the on_delete
parameter, you won't be left guessing its purpose. Feel free to explore the documentation for more information, and don't hesitate to reach out if you have any further questions or want to share your thoughts. 😊👍