RuntimeWarning: DateTimeField received a naive datetime
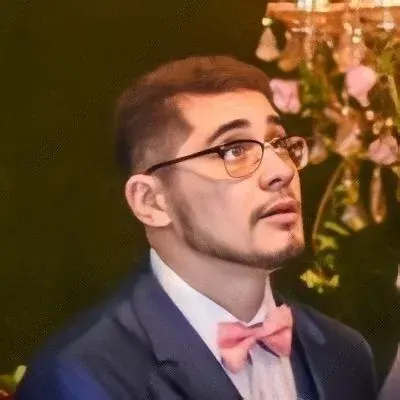
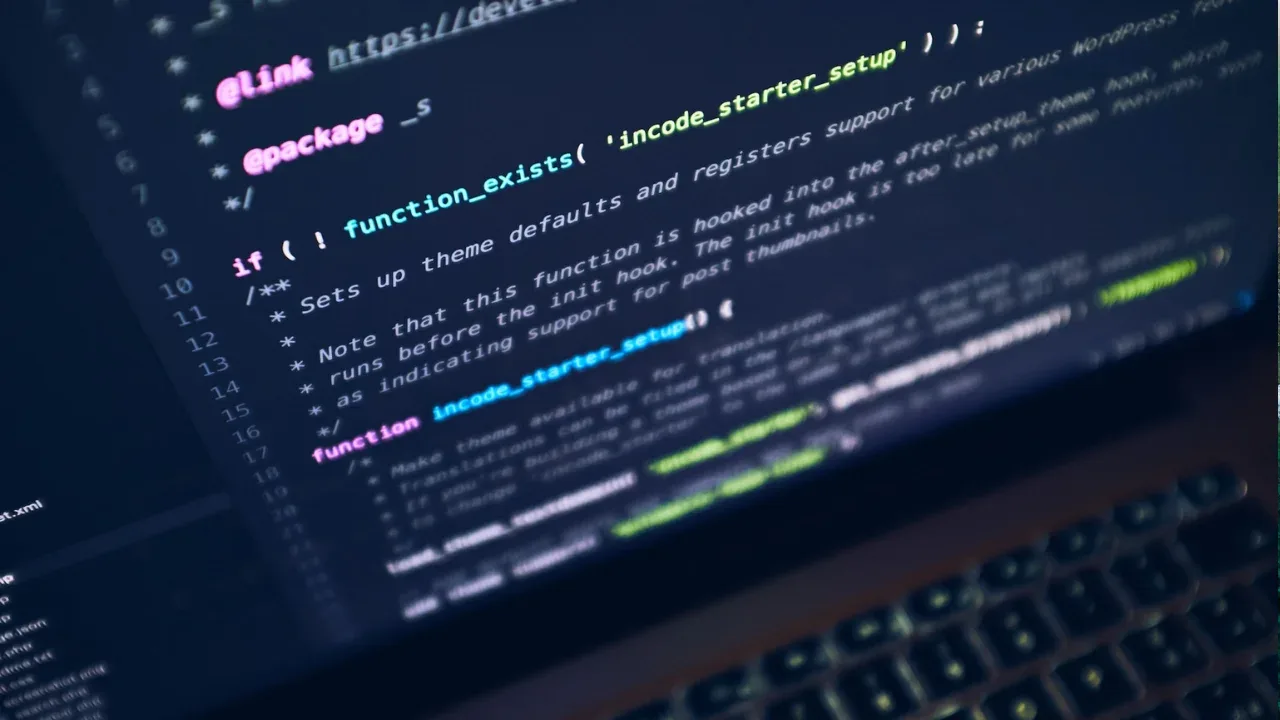
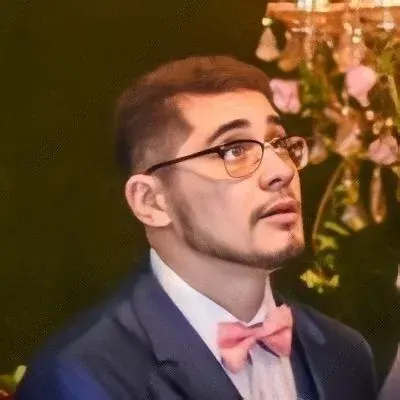
📝✉️ Fixing the "RuntimeWarning: DateTimeField received a naive datetime" Error in Django
If you're encountering the "RuntimeWarning: DateTimeField received a naive datetime" error while working with Django, don't worry, there's a simple solution for you! 😎🔧
🔍 The Problem: Naive Datetime 🔍
This error occurs when you're trying to use a DateTimeField in Django with a naive datetime object. A naive datetime object doesn't have any knowledge of the timezone, while Django requires timezone-aware datetime objects for DateTimeFields. 📅⏰
🛠️ The Solution: Activating Timezone Support 🛠️
To fix this issue, follow these simple steps:
Open your Django project's
settings.py
file.Add the line
USE_TZ = True
to enable timezone support. ✅If you haven't already installed it, install the
pytz
library using the commandpip install pytz
. This library provides timezone-aware datetime support for Python and Django. 🌍🕒
That's it! With these changes, you'll now be able to use DateTimeFields without encountering the "RuntimeWarning: DateTimeField received a naive datetime" error. 🚀
🔍 Understanding the Error: An Example 🔍
Let's consider an example to understand this error better. Suppose you have a Django project where you want to save the creation time of a blog post. You define a model like this:
from django.db import models
class BlogPost(models.Model):
title = models.CharField(max_length=100)
content = models.TextField()
created_at = models.DateTimeField()
In this case, if you try to create a new blog post without providing a timezone-aware datetime object for the created_at
field, you'll encounter the "RuntimeWarning: DateTimeField received a naive datetime" error. 😮
🔧 Implementing the Solution: A Practical Example 🔧
To fix this issue for our example, follow these steps:
Open your Django project's
settings.py
file.Add the line
USE_TZ = True
to enable timezone support.Install the
pytz
library using the commandpip install pytz
.In your code where you create a new blog post, use
timezone.now()
fromdjango.utils.timezone
to get a timezone-aware datetime object:
from django.utils import timezone
new_post = BlogPost(
title="Example Post",
content="This is an example blog post.",
created_at=timezone.now()
)
new_post.save()
Now, when you save the blog post, the created_at
field will contain a timezone-aware datetime object, and you won't encounter the "RuntimeWarning: DateTimeField received a naive datetime" error anymore. 🎉
💡 Take Action and Elevate Your Django Skills! 💡
The "RuntimeWarning: DateTimeField received a naive datetime" error might seem tricky at first, but with the solutions provided above, you'll be able to overcome it easily. 🎯✅
So go ahead, implement the solution, and start building amazing Django applications without worrying about timezone-related errors! If you'd like to learn more about Django or have any questions, feel free to leave a comment below. Let's build together! 🌟🚀