In a django model custom save() method, how should you identify a new object?
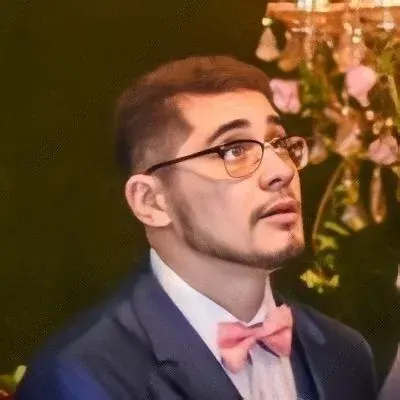
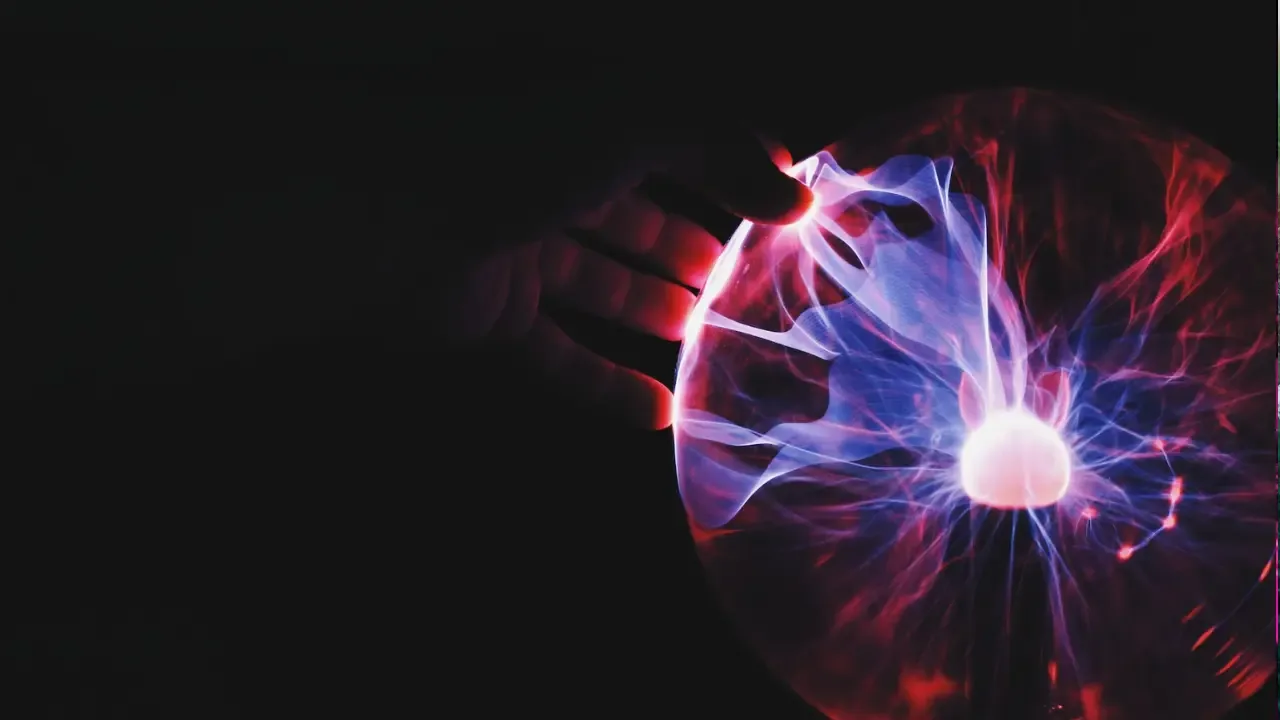
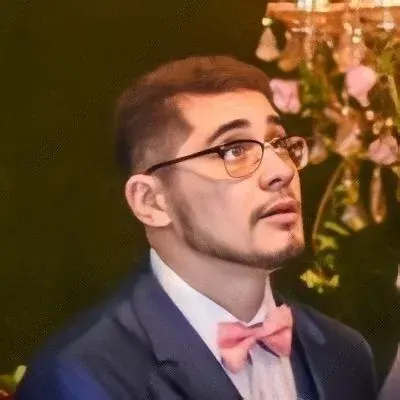
Identifying a New Object in Django Model's Custom save()
Method 🐍
So, you want to perform a special action when saving a new record in Django's save()
method? 🤔 No worries, mate! We got you covered! In this blog post, we'll explore how to identify a new object and trigger that desired action. Let's dive in! 💪
The Dilemma: Ensuring We're Dealing with a New Object 😓
When it comes to the save()
method, distinguishing between creating a new record and updating an existing one can be a bit tricky. The id
attribute of a Django Model
object can help us differentiate between the two. But is it enough? Let's find out! 💡
The Naive Approach: Comparing with None
🤷♀️
One might be tempted to use the comparison self.id != None
to check if the object is new. Though it seems plausible, this might not be the most reliable method. Here's why:
✨ Issue 1: Unsaved Objects ✨
Before an object is saved to the database, its id
is None
. So, technically, using self.id != None
would indicate a new object. However, it wouldn't detect changes made to an existing record that hasn't been saved yet. Crazy, right? 😵
✨ Issue 2: User Overrides ✨
Another problem arises when users or developers manually set the id
of an object before saving it. In such cases, using self.id != None
would mistakenly identify the record as an update, when in fact, it's a brand new object. Oops! 😬
A Better Way: Leveraging Django's _state
Attribute 🌟
To overcome the limitations of the naive approach, Django provides us with the _state
attribute that helps identify whether an object is new or not. Here's how it works:
def save(self, *args, **kwargs):
if self._state.adding:
# Perform special actions for new objects
else:
# Perform actions for updating existing objects
super().save(*args, **kwargs)
By checking self._state.adding
, we can reliably determine if the object is being created for the first time. 🎉
Handling Special Cases: What Could Go Wrong? 🤔
While using self._state.adding
is quite dependable, there are always exceptions to the rule. Here are a few special cases to be aware of:
🚧 Case 1: Object Deletion 🚧
When an object is deleted and then recreated with the same primary key, self._state.adding
would incorrectly indicate it as an update. If you're not expecting this scenario, take precautionary measures to avoid unwanted surprises. 🛠️
🚧 Case 2: Deferred Object Creation 🚧
Django supports deferred object creation, which allows you to create objects without saving them immediately. In such cases, self._state.adding
would return False
until you explicitly save the object. Keep this in mind when working with deferred creation. 💭
Your Call to Action: Engage and Share! 📣
And that's a wrap! You now possess the knowledge to confidently identify new objects in Django's save()
method while avoiding common pitfalls. 👏
Share this blog post with your fellow Django developers, and let's spread the word about this handy technique! 💻🌐
If you have any questions, scenarios, or personal experiences with object identification, drop a comment below. Let's start a discussion and help each other out! 🗣️💬
Stay tuned for more helpful Django tips and tricks. Until then, happy coding! 😄🐍
Disclaimer: The information in this blog post is based on Django version 3.2. Some implementation details may vary in earlier or later versions.