In a Django form, how do I make a field readonly (or disabled) so that it cannot be edited?
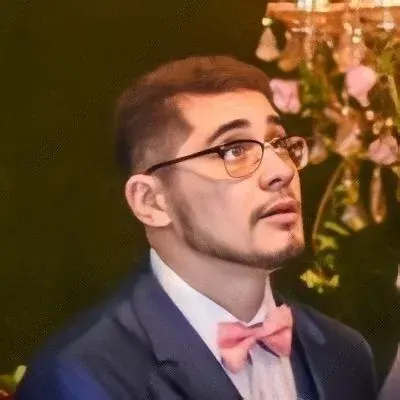
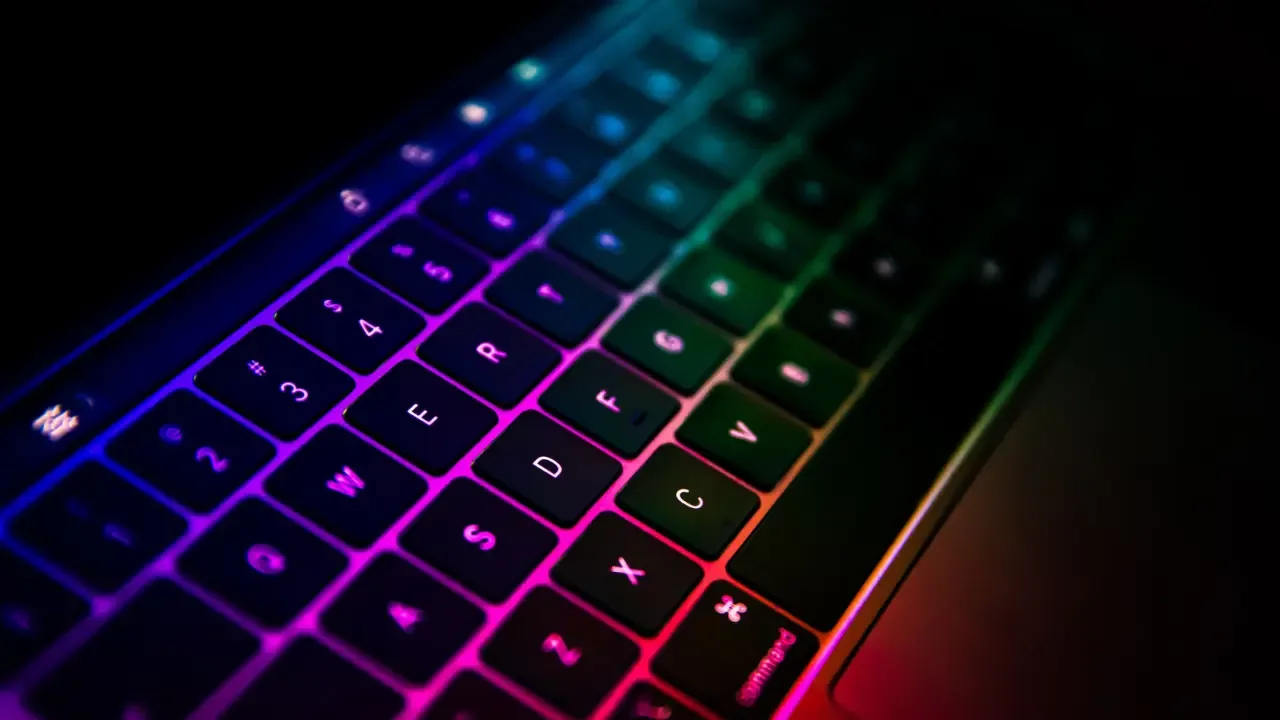
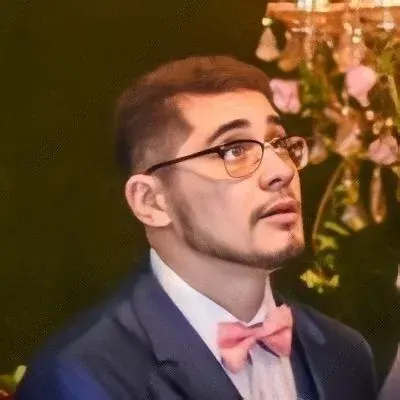
How to Make a Field Readonly in Django Form 📝🔒
Do you need to make a field in your Django form read-only or disabled so that it cannot be edited? 🤔 Don't worry, we've got you covered! In this blog post, we'll guide you through the process of making a field readonly in a Django form, whether you are creating a new entry or updating an existing record. Let's dive right in! 💪
The Scenario 🌄
Imagine you have a Django form for creating or updating items. When the form is used for creating a new entry, all fields should be editable. However, when the record is in update mode, certain fields should be read-only or disabled. For example, let's consider the sku
field in the Item
model. While updating the record, you want the sku
field to be visible but not editable.
Reusing the Form Class 🔄
Before we address the read-only field issue, let's talk about reusing the form class. Can the ItemForm
class be reused for both creating and updating items? 🔄 Absolutely! Here's what you need to do:
In the
ItemForm
class'sMeta
class, add theexclude
attribute to exclude theadded_by
field.class ItemForm(ModelForm): class Meta: model = Item exclude = ('added_by',)
In the
new_item_view
, use theItemForm
class for both creating and updating items.def new_item_view(request): if request.method == 'POST': form = ItemForm(request.POST) # Validate and save the form else: form = ItemForm() # Render the view
By excluding the added_by
field in the form class and using the same form for both creating and updating, you can easily reuse the ItemForm
class. 🔄
Making a Field Readonly or Disabled 🔒
Now, let's focus on making the sku
field readonly or disabled while updating the record. Here's what you need to do:
Create a new form class,
ItemUpdateForm
, that inherits fromItemForm
.class ItemUpdateForm(ItemForm): class Meta: model = Item fields = '__all__'
Override the widget for the
sku
field in theItemUpdateForm
class and set theattrs
dictionary to make the field readonly or disabled.class ItemUpdateForm(ItemForm): class Meta: model = Item fields = '__all__' def __init__(self, *args, **kwargs): super().__init__(*args, **kwargs) self.fields['sku'].widget.attrs['readonly'] = True # or 'disabled' instead of 'readonly'
In the
update_item_view
, use theItemUpdateForm
class for updating the item.def update_item_view(request): if request.method == 'POST': form = ItemUpdateForm(request.POST) # Validate and save the form else: form = ItemUpdateForm()
By customizing the widget attributes in the ItemUpdateForm
class, you can make the sku
field readonly or disabled when updating the item. 🔒
Conclusion and Call-to-Action ✅📢
Congratulations! You have learned how to make a field readonly or disabled in a Django form. Now you can control the editability of fields based on your specific requirements. Remember, you can easily reuse form classes for different operations by customizing the form's metadata. 🔄💡
If you found this guide helpful, why not share it with your fellow Django developers? Let them know the best practices for making fields readonly or disabled in Django forms. 🤗🚀