How to upload a file in Django?
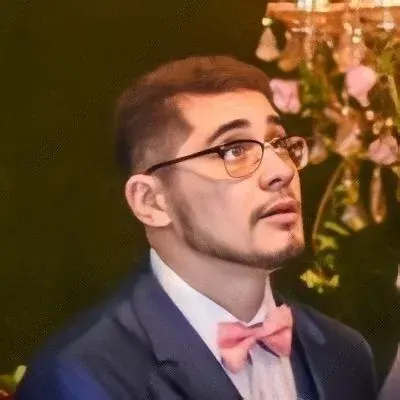
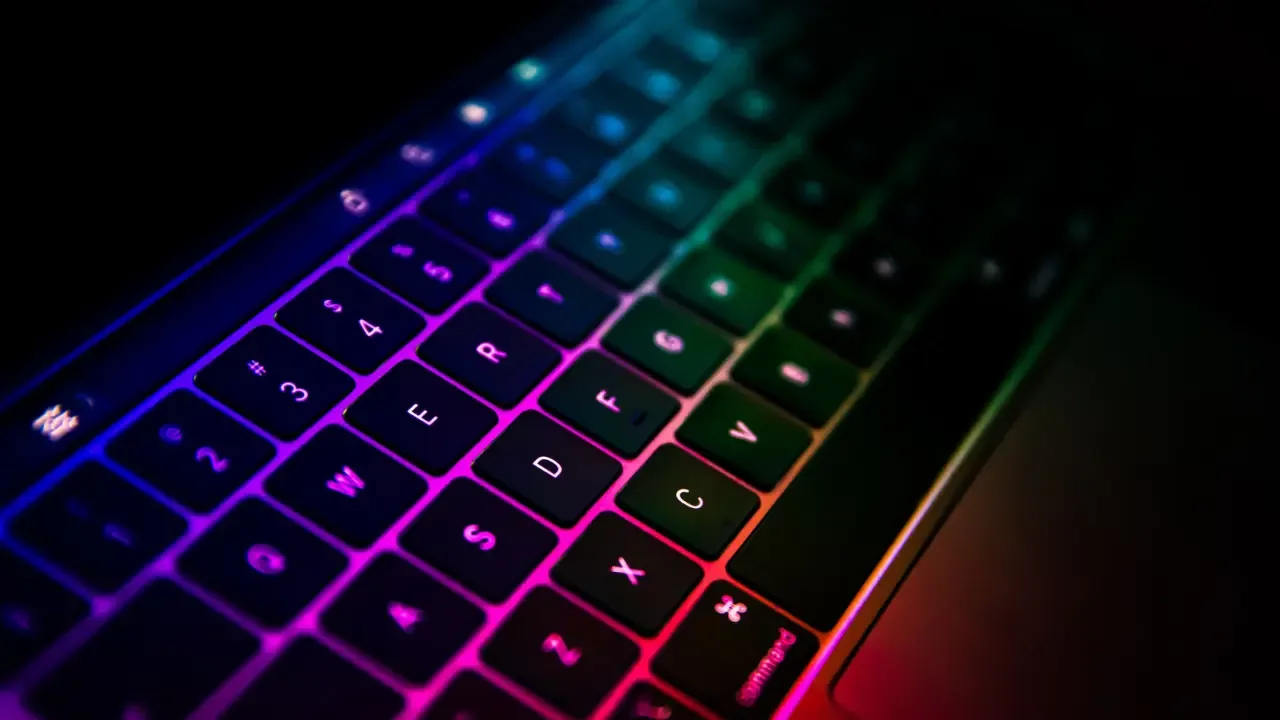
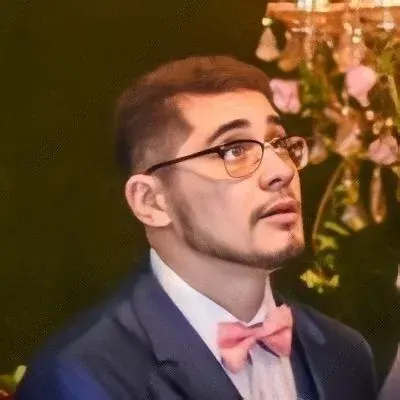
How to Upload a File in Django: A Beginner's Guide
Are you a Django developer trying to figure out how to enable file uploads in your web application? 🤔 Look no further! In this guide, we will walk you through the process step by step. By the end, you'll know exactly how to implement file uploading in your Django app. 💪
Why Uploading Files is Important
File uploading is a critical feature in many web applications. It allows users to share images, documents, videos, and more. Whether you're building a social media platform, a file management system, or an e-commerce website, enabling file uploads can greatly enhance user interaction and engagement. 😎
The Minimal Example Code
Let's start with the minimal example code you need to create a "hello world" app in Django that enables users to upload a file. 🔍
Here's what you should do:
First, make sure you have Django 1.3 or a later version installed.
Create a new Django project by running the following command in your terminal:
django-admin startproject myproject
Create a new Django app within your project by running the following command:
python manage.py startapp myapp
Open the
myapp/views.py
file and add the following code:from django.shortcuts import render def upload_file(request): if request.method == 'POST': file = request.FILES['file'] # Perform any necessary operations with the uploaded file # (e.g., save it to a database, process its contents, etc.) return render(request, 'upload_success.html') return render(request, 'upload.html')
Create two HTML templates
upload.html
andupload_success.html
. Inupload.html
, add a form that allows users to upload a file. Here's an example:<form method="post" enctype="multipart/form-data"> {% csrf_token %} <label for="file">Select a file:</label> <input type="file" name="file" id="file"> <button type="submit">Upload</button> </form>
Configure your app's routes by opening the
myproject/urls.py
file and adding the following code:from django.urls import path from myapp.views import upload_file urlpatterns = [ path('upload/', upload_file, name='upload_file'), ]
Run your Django server by executing the following command:
python manage.py runserver
And that's it! You now have a "hello world" Django app that enables users to upload a file. 🎉
Troubleshooting Common Issues
If you encounter any issues or have questions, here are some common problems and their solutions:
1. "CSRF verification failed" error:
Make sure you included {% csrf_token %}
in your form.
2. Uploaded files not being processed:
Check that you're handling the uploaded file correctly in your view. You can save it to your server, store it in a database, or perform any other necessary processing.
3. File size limitation:
By default, Django limits the maximum upload size to 2.5MB. If you need to allow larger file sizes, you can configure the FILE_UPLOAD_MAX_MEMORY_SIZE
and MAX_UPLOAD_SIZE
settings in your settings.py
file.
Remember, practice makes perfect! Don't be afraid to experiment and explore further customizations to fit your specific needs. Django offers a lot of flexibility, so feel free to dive deeper into its documentation if you want to learn more! 📚
Join the Django Community
Still have questions or need additional guidance? Join the vibrant Django community! 🌐 You can find helpful resources, tutorials, and engage in discussions on platforms like Stack Overflow and the official Django forum. And don't forget to share your success stories with us! We'd love to hear how you incorporate file uploads into your Django applications.
So what are you waiting for? Start building fantastic applications with Django's file uploading feature today! 🚀
Have any cool file uploading stories or tips to share? Drop them in the comments below. Let's learn from each other! 💬