How to get Request.User in Django-Rest-Framework serializer?
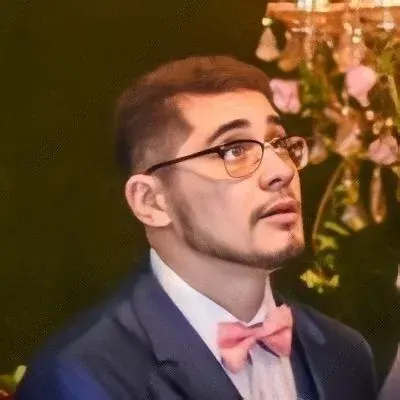
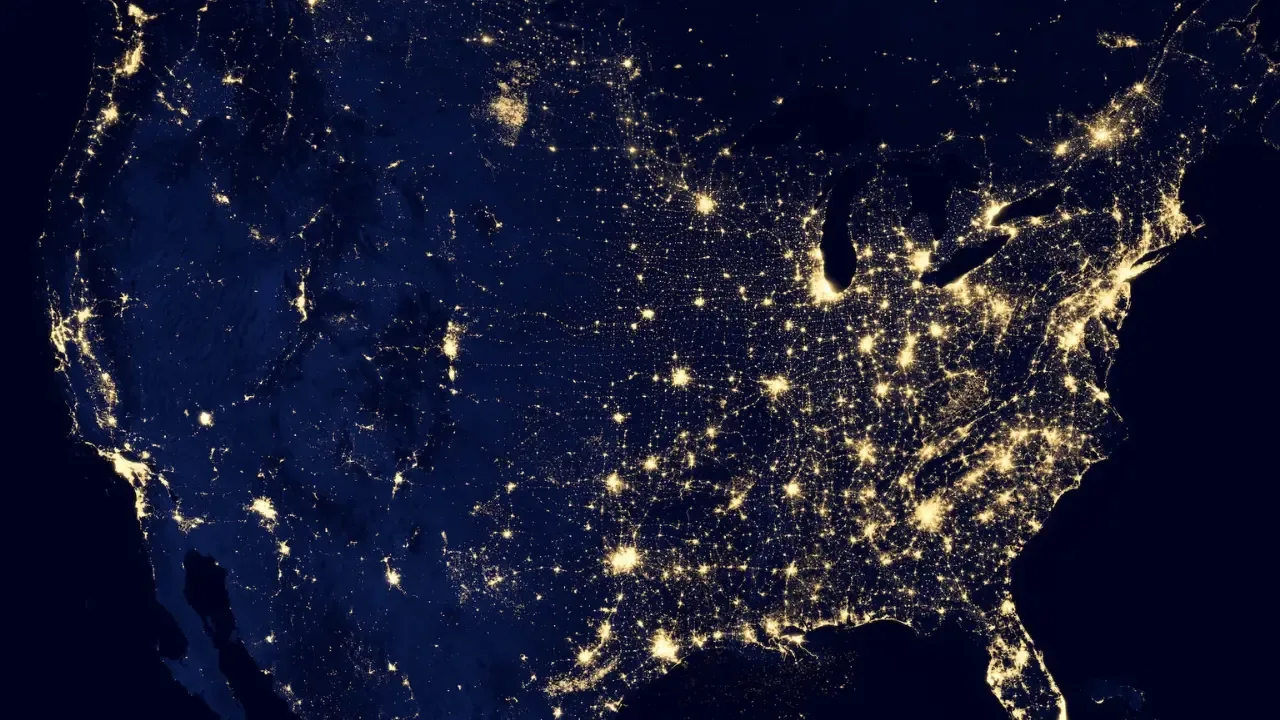
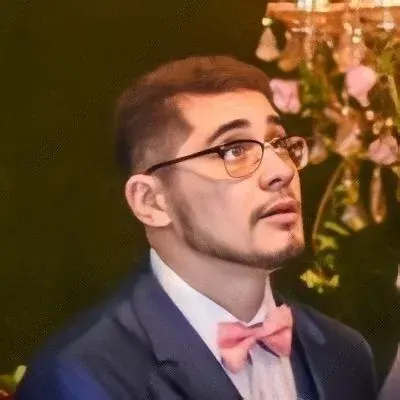
π Title: "Unlocking the Power of Request.User in Django-Rest-Framework Serializer π"
Introduction: Hey there, tech enthusiasts π! If you're diving into the Django Rest Framework and finding yourself scratching your head over how to access the Request.User object in your serializer class, you've come to the right place! π― In this post, we'll tackle this common challenge head-on and equip you with easy solutions. Let's dive right in! πͺ
Understanding the Problem: The challenge begins when we try accessing the Request.User object within the serializer class. As shown in the example code π₯, attempting to access it directly will lead to disappointment. But don't worryβwe've got your back! π€
Solution 1: Using the request context The first solution involves using the request context π. By passing it to the serializer during initialization, we can easily access the Request.User object.
# Modifying the serializer code
class PostSerializer(serializers.ModelSerializer):
class Meta:
model = Post
def save(self):
user = self.context['request'].user
title = self.validated_data['title']
article = self.validated_data['article']
Here, we accessed the request object from the context by using self.context['request']
. Then, by calling .user
on the request object, we successfully obtained the user information π.
Solution 2: Extending the serializer
In some cases, you might want to access the Request.User object across different serializer methods, not only in the save()
method. In such scenarios, extending the serializer class might be a more appropriate solution.
# Create a new serializer class as a parent
class CustomBaseSerializer(serializers.ModelSerializer):
def __init__(self, *args, **kwargs):
super().__init__(*args, **kwargs)
self.user = self.context['request'].user
# Extend the new serializer class
class PostSerializer(CustomBaseSerializer):
class Meta:
model = Post
def save(self):
user = self.user
title = self.validated_data['title']
article = self.validated_data['article']
In this solution, we created a parent serializer class called CustomBaseSerializer
. It extracts the Request.User object from the context during initialization. By extending this parent class in our PostSerializer
, we gain access to the user information throughout the serializer's methods π.
Call-to-Action: Congratulations, you've leveled up your Django Rest Framework knowledge! π Now you can effortlessly access the Request.User object within your serializer classes like a pro. Go ahead and give these solutions a try on your own projects π . If you have any questions or other Django-related topics you'd like us to cover, let us know in the comments below. Happy coding! π»π
So, techies, did you find this guide helpful? Don't forget to share it with your fellow developers and give us your thoughts in the comments. Let's spread the knowledge! ππ‘
Keep coding and stay curious! πβ¨