How to express a One-To-Many relationship in Django?
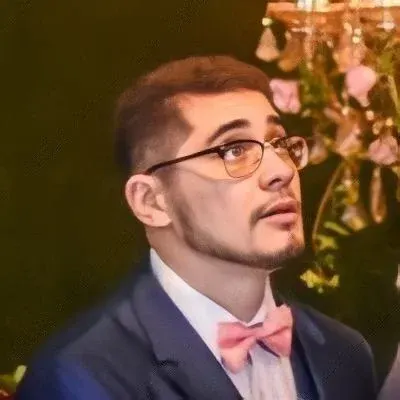

How to Express a One-To-Many Relationship in Django?
So you're building your Django models, and you encounter a problem: there's no OneToManyField
in the model field types. 🤔 Don't worry, there's a way to handle a one-to-many relationship in Django, and I'm here to guide you through it! 🚀
Understanding the Problem
Let's take a look at the example you provided:
class Dude(models.Model):
numbers = models.OneToManyField('PhoneNumber')
class PhoneNumber(models.Model):
number = models.CharField()
In this case, each Dude
can have multiple PhoneNumber
s. However, the relationship should be unidirectional, meaning you don't need to know which Dude
owns a specific PhoneNumber
.
The Solution: ForeignKey
To represent a one-to-many relationship in Django, you can use the ForeignKey
field. 🎉
Step 1: Update the Dude
model
Modify the Dude
model to include a ForeignKey
field pointing to the PhoneNumber
model:
class Dude(models.Model):
# 1 dude can have 0+ phone numbers
# Use 'PhoneNumber' string to reference the PhoneNumber model
number = models.ForeignKey('PhoneNumber', on_delete=models.CASCADE)
Here, the on_delete=models.CASCADE
parameter ensures that when a PhoneNumber
is deleted, the associated Dude
will also be deleted.
Step 2: Clarify Ownership in PhoneNumber
Since you mentioned that the PhoneNumber
model can be owned by various objects like Dude
and Business
, let's add a field to indicate the object's ownership. You can achieve this by introducing a GenericForeignKey
field.
from django.contrib.contenttypes.fields import GenericForeignKey
from django.contrib.contenttypes.models import ContentType
class PhoneNumber(models.Model):
number = models.CharField()
content_type = models.ForeignKey(
ContentType, on_delete=models.CASCADE
)
object_id = models.PositiveIntegerField()
content_object = GenericForeignKey('content_type', 'object_id')
By adding these fields, you can now associate a Dude
or a Business
with a specific PhoneNumber
.
📣 Call-to-Action: Engage in the Django Community
Congratulations! You now know how to express a one-to-many relationship in Django using the ForeignKey
field. 🎉
But don't stop here - the Django community is vast and filled with knowledge. Join the conversation and share your experiences, challenges, and solutions by:
Participating in Django forums, such as the official Django Users Group.
Posting your questions on Stack Overflow.
Contributing to the Django project itself by checking out their contributing guidelines.
Remember, the more you engage, the more you'll learn and grow alongside other Django enthusiasts. Happy coding! 💻🐍
Take Your Tech Career to the Next Level
Our application tracking tool helps you manage your job search effectively. Stay organized, track your progress, and land your dream tech job faster.
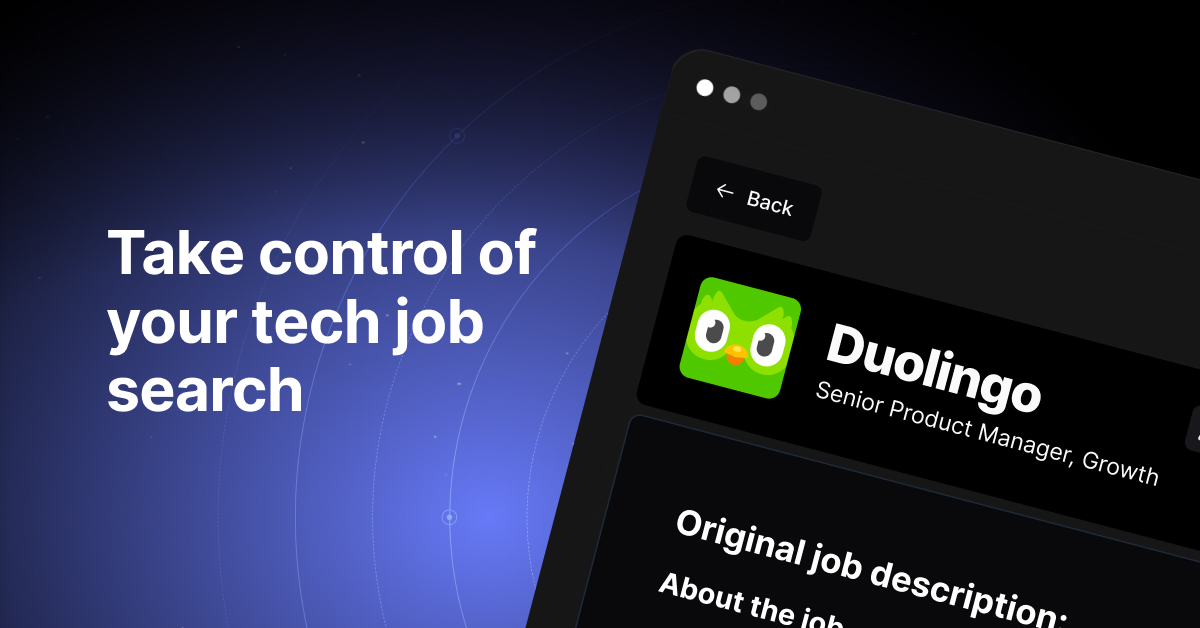