How to disable admin-style browsable interface of django-rest-framework?
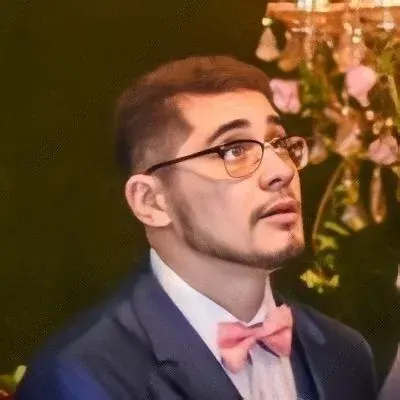
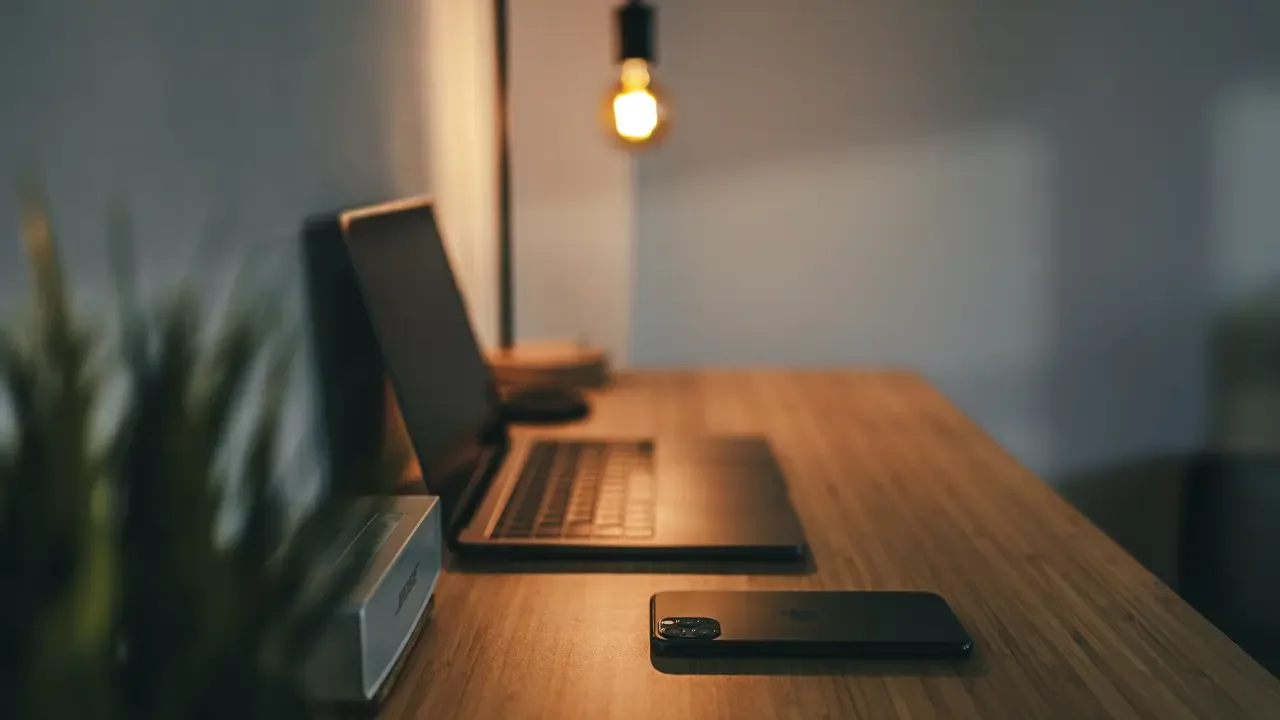
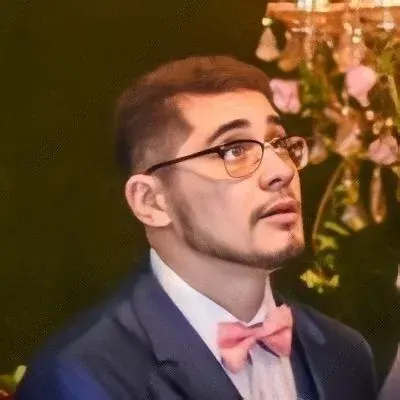
How to Disable the Admin-Style Browsable Interface of Django Rest Framework
So, you're using the amazing Django Rest Framework (DRF), and it's providing you with a beautiful admin style browsable self-documenting API. But uh-oh! Anyone can visit those pages and start adding data through the interface using POST requests. 😱
Don't worry, disabling this feature is as easy as pie! 🍰 In this blog post, we'll walk you through how to disable the admin-style browsable interface of DRF, ensuring that only authorized users can access and modify your API.
The Problem
The default setup of DRF allows open access to the browsable interface, which means anyone with the URL can interact with your API. While this can be handy during development, it poses a significant security risk in production environments.
The Solution
Here's what you need to do to disable the admin-style browsable interface in DRF:
Step 1: Update your Django settings
Open your project's settings.py
file and look for the REST_FRAMEWORK
configuration. If it doesn't exist, you can add it to the bottom of your file. Ensure the DEFAULT_RENDERER_CLASSES
setting is overridden as follows:
REST_FRAMEWORK = {
'DEFAULT_RENDERER_CLASSES': (
'rest_framework.renderers.JSONRenderer',
),
}
By specifying only the JSONRenderer
, we're essentially telling DRF to only render responses as JSON, removing the browsable interface.
Step 2: Revise URL Configuration
To ensure users can no longer access the browsable interface, we need to update the URL configuration. In your project's urls.py
file, look for the URL patterns related to the DRF browsable interface. If you're using the default configuration, it should resemble something like this:
from django.urls import path, include
from rest_framework import routers
router = routers.DefaultRouter()
router.register(r'your-endpoint', YourViewSet)
urlpatterns = [
# other URL patterns
path('api/', include(router.urls)),
path('api-auth/', include('rest_framework.urls', namespace='rest_framework')),
]
To disable the browsable interface, you need to remove the line that includes the 'rest_framework.urls'
module, resulting in the following code:
from django.urls import path, include
from rest_framework import routers
router = routers.DefaultRouter()
router.register(r'your-endpoint', YourViewSet)
urlpatterns = [
# other URL patterns
path('api/', include(router.urls)),
]
Take It to the Next Level
Congratulations, you have successfully disabled the admin-style browsable interface of DRF! 🎉 However, don't stop here – let's take it to the next level.
To secure your API and restrict access only to authorized users, make sure to implement appropriate authentication and permission mechanisms. DRF offers a wide range of authentication and permission classes that can be easily integrated into your project.
Conclusion
In this blog post, we learned how to disable the admin-style browsable interface in Django Rest Framework. Protecting your API from unauthorized access is crucial, and by following the steps outlined above, you've taken a significant leap towards securing your application.
Now it's your turn to take action! Update your project's settings and URL configuration to prevent any Tom, Dick, or Harry from adding data through the interface. 💪 And don't forget to implement proper authentication and permission mechanisms for even greater security.
If you found this blog post helpful, make sure to share it with your fellow developers. Let's secure our APIs together! 🚀🔒