How to define two fields "unique" as couple
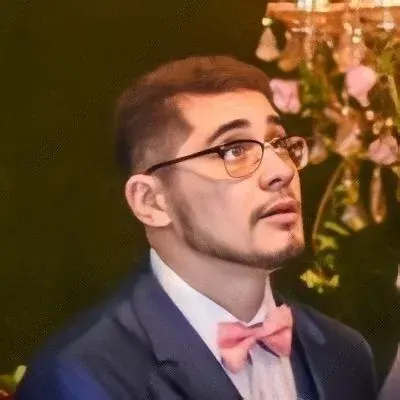
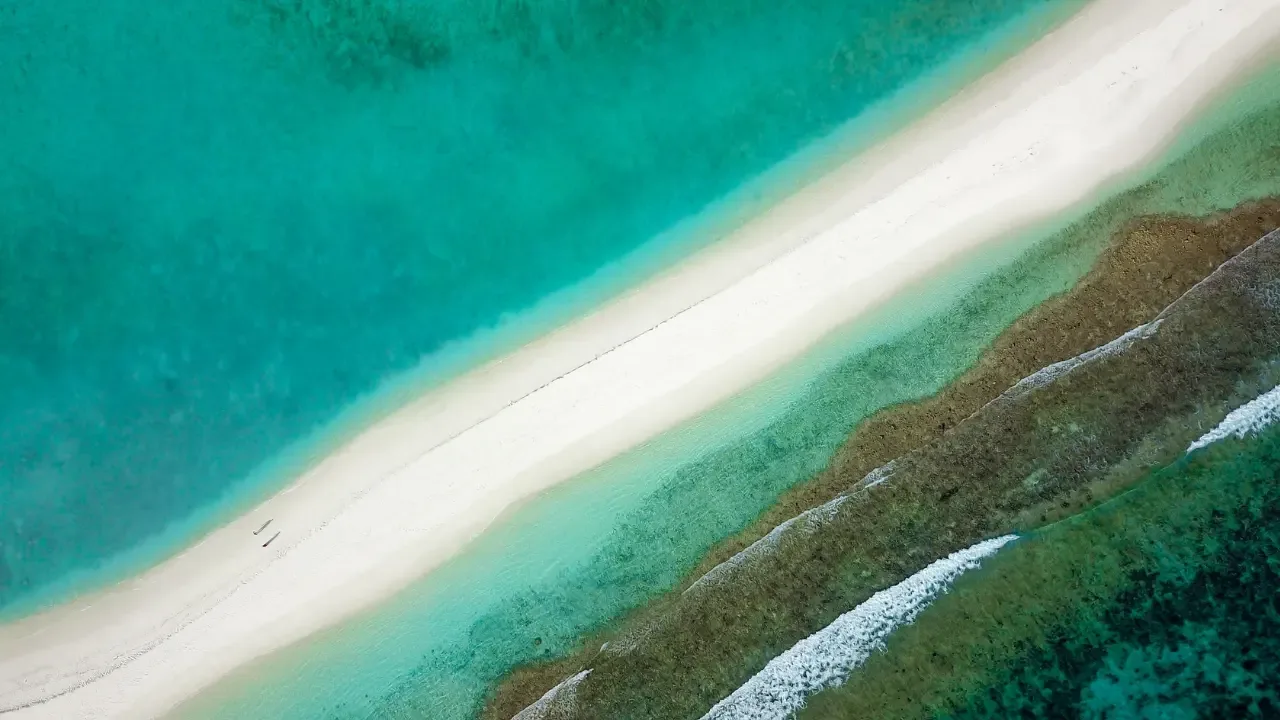
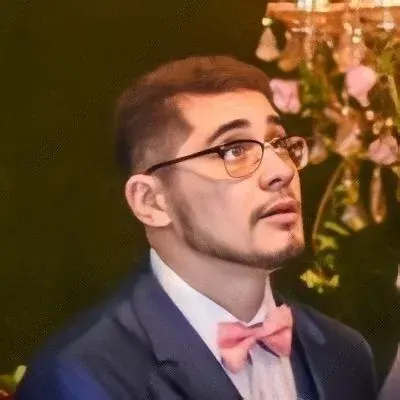
How to Define Two Fields as "Unique" as a Couple in Django
Are you faced with the challenge of defining a couple of fields as unique in Django? 🤔 Specifically, are you struggling to ensure that no more than one volume number is associated with the same journal in your table of volumes? 📚 Well, fear not! We have got you covered with some easy solutions to make your life easier. 😎
Let's dive right in!
The Problem
In the provided context, the Volume
model has two fields: journal_id
and volume_number
. The objective is to prevent the occurrence of multiple volume numbers for the same journal.
The Attempted Solution
One might initially think that setting the unique
attribute to True
for the fields journal_id
and volume_number
would do the trick. However, as mentioned, this approach did not yield the desired results. 😫
The Solution
To achieve the desired unique combination validation, you can leverage Django's powerful UniqueConstraint
class. This class allows you to specify a set of fields that must be unique together. 😄
Here's how you can implement it:
from django.db import models
from django.db.models import UniqueConstraint
class Volume(models.Model):
id = models.AutoField(primary_key=True)
journal_id = models.ForeignKey(Journals, db_column='jid', null=True, verbose_name="Journal")
volume_number = models.CharField('Volume Number', max_length=100)
comments = models.TextField('Comments', max_length=4000, blank=True)
class Meta:
constraints = [
UniqueConstraint(fields=['journal_id', 'volume_number'], name='unique_volume'),
]
Using the UniqueConstraint
class, the fields journal_id
and volume_number
are specified within the fields
attribute. The name
attribute allows you to assign a name for the constraint (in this case, we have used "unique_volume"
).
Now, Django will enforce the uniqueness of the combination of these two fields, ensuring that no duplicate volume numbers are associated with the same journal. 🙌
Conclusion
By utilizing Django's UniqueConstraint
class, you can easily define two fields as "unique" as a couple, resolving the issue of multiple volume numbers for the same journal. 🎉
So, why wait? Implement this solution today and save yourself from any future data integrity headaches. 💪
If you found this guide helpful, don't forget to share it with your fellow developers! And if you have any questions or alternative solutions, let us know in the comments section below. We would love to hear from you! 😊