How to "bulk update" with Django?
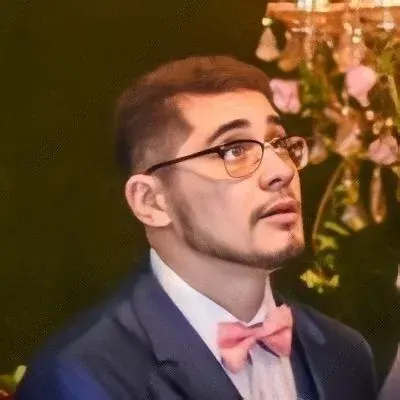
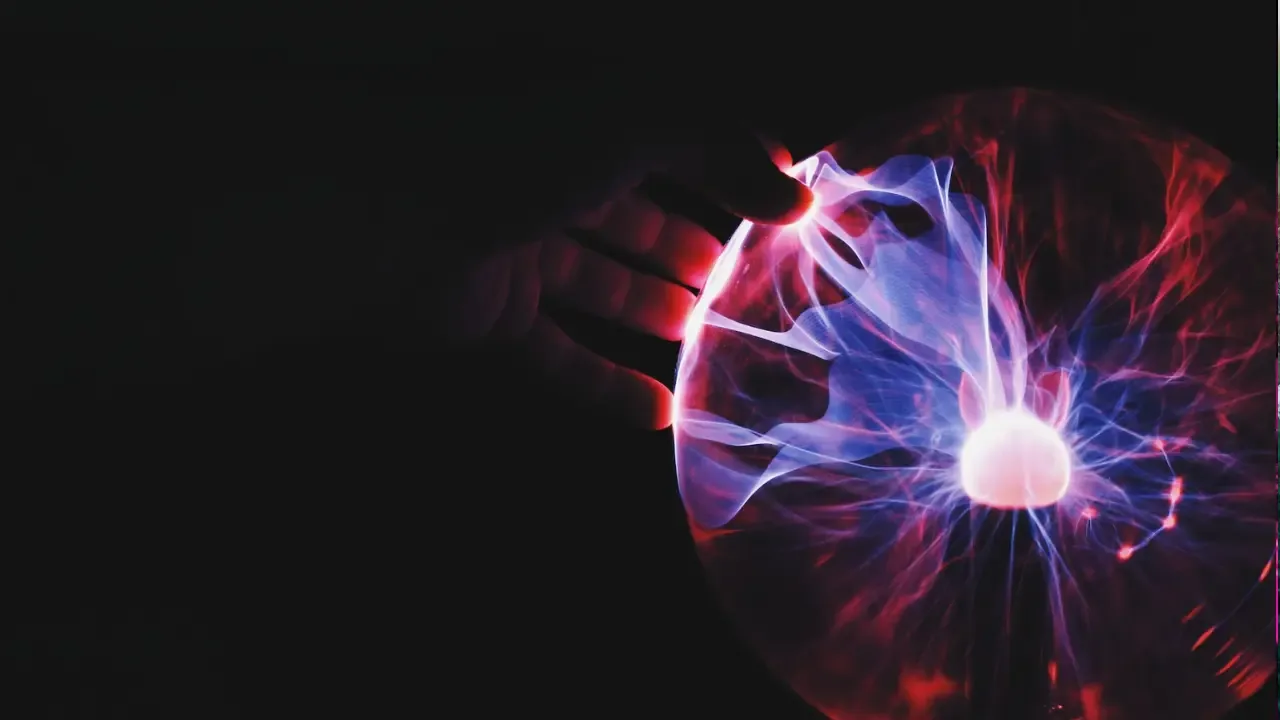
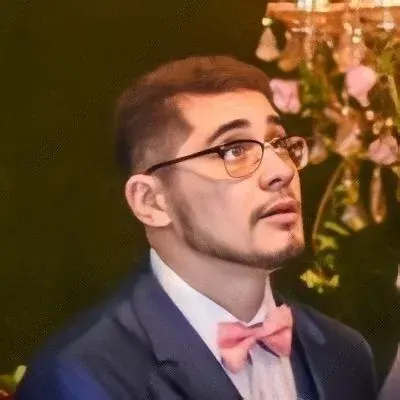
How to 'Bulk Update' with Django? 💪
Are you tired of writing long and tedious code to update your Django models one by one? We feel you! Updating data in bulk shouldn't be a pain. In this blog post, we'll explore a more elegant solution to perform bulk updates in Django. Say goodbye to repetitive code and hello to efficiency!
The Nasty Approach 😖
Let's start by addressing the "nasty" approach you mentioned in your question. Although functional, iterating over a queryset and saving each object individually can be inefficient and time-consuming. Here's a reminder of the code you showed us:
list = ModelClass.objects.filter(name='bar')
for obj in list:
obj.name = 'foo'
obj.save()
While this code gets the job done, it hits the database multiple times, resulting in unnecessary overhead. Fear not, for there's a better way to achieve the same result!
The Elegant Solution 💡
Django provides a convenient method called update()
that allows you to perform bulk updates efficiently. Let's demonstrate how it works for your case:
ModelClass.objects.filter(name='bar').update(name='foo')
Voila! With a single line of code, you can update all instances of ModelClass
where the name is 'bar' to 'foo'. Isn't that much cleaner and easier to read? 😊
The update()
method executes a single SQL statement in the database, resulting in improved performance over the previous approach. It's perfect for bulk updates, saving you precious time and effort.
Remember the Caveats ⚠️
Before you go update-crazy, it's important to note a few caveats when using the update()
method:
Hooks and signals: The
update()
method bypasses model save methods and signals. If you have specific functionalities triggered upon saving an object, make sure to handle them accordingly.Field validation and default values: The
update()
method doesn't invoke any field validation or utilize default values. If you rely on these mechanisms, ensure they are applied manually in your code.Limited functionality: While the
update()
method shines in bulk updates, it doesn't support complex operations involving multiple fields. For advanced scenarios, you may need to resort to other techniques or introduce additional logic.
Engage with the Community! ✨
We hope this guide helped you understand how to perform 'bulk updates' with Django in a more elegant way. Now it's your turn to try it out and share your experience!
Have you encountered other hurdles when updating models in bulk? Any cool tricks you'd like to share? Let us know in the comments below! Let's create a vibrant community of Django enthusiasts and help each other become more productive developers. 💪🌟
So what are you waiting for? Grab that keyboard, unleash your coding skills, and start optimizing those bulk updates with Django today! Happy coding! 🎉