How to add multiple objects to ManyToMany relationship at once in Django ?
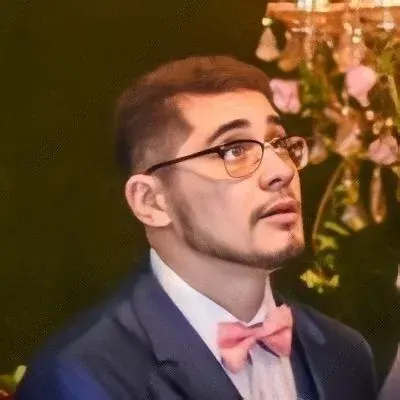
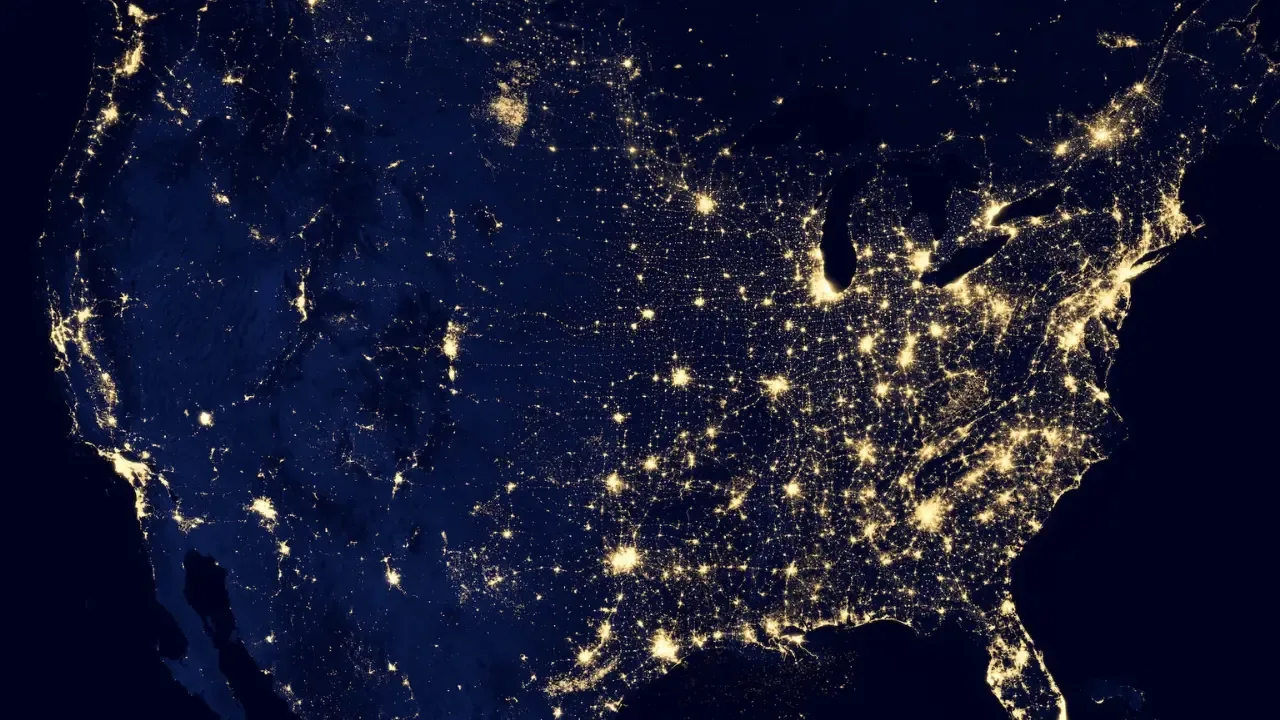
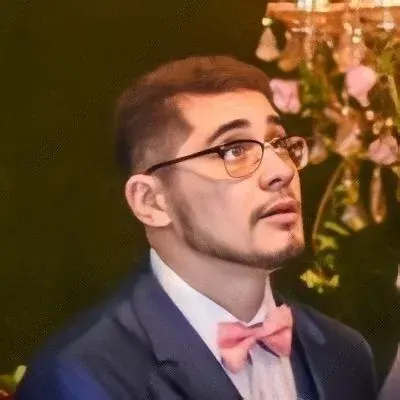
📝 Adding Multiple Objects to ManyToMany Relationship at Once in Django
Are you facing the frustrating TypeError: unhashable type: 'list'
when you try to add multiple objects to a ManyToMany relationship in Django? Don't worry, you're not alone! In this blog post, we'll explore this common issue and provide you with easy solutions to overcome it. Let's dive in!
Understanding the Problem
Django's documentation mentions that you can add multiple objects at once to a ManyToMany relationship. However, when you try to do so by passing a list (or even a Django QuerySet), you encounter the dreaded TypeError
.
The error occurs because the ManyToMany's add
method internally uses a set to store the object IDs for efficient lookup. But lists are mutable and cannot be hashed, resulting in this error.
Easy Solutions
Solution 1: Using a for Loop
One straightforward way to add multiple objects is by using a for loop to iterate over the objects and add them individually. While this solution works, it might not be the most efficient approach, especially if you have a large number of objects to add.
Here's an example code snippet using a for loop:
objects_to_add = [object1, object2, object3]
for obj in objects_to_add:
my_model.many_to_many_field.add(obj)
Solution 2: Using the unpacking operator
A more elegant solution is to use the unpacking operator (*
) to expand the objects and pass them individually to the add
method. This way, you can avoid the error and simplify your code.
Here's an example of how to use the unpacking operator:
objects_to_add = [object1, object2, object3]
my_model.many_to_many_field.add(*objects_to_add)
By passing *objects_to_add
, each object is unpacked and separately added to the ManyToMany relationship. This method is not only concise but also more efficient than using a for loop for larger lists of objects.
Solution 3: Using the set() Function
Another way to resolve the TypeError issue is by converting the list to a set using the set()
function. Since sets are hashable, they can be used with ManyToMany fields without any errors.
Here's an example:
objects_to_add = [object1, object2, object3]
my_model.many_to_many_field.add(*set(objects_to_add))
By wrapping objects_to_add
in set()
, we eliminate any duplicate objects and ensure that it is hashable for adding to the ManyToMany relationship.
Your Turn!
Now that you have three easy solutions to add multiple objects to a ManyToMany relationship in Django, it's time to put them into practice! Experiment with each solution and see which one works best for your specific scenario.
If you have any other Django-related questions or want to learn more about simplifying complex tasks, feel free to reach out in the comments section below. Happy coding!
🔗 Read More: For additional Django tips and tricks, check out our comprehensive guide on Django Best Practices.
📣 Join the Community: Share your experiences and insights with fellow Django developers on our vibrant Django Devs Forum. Don't miss out on the opportunity to learn from others and grow together!
💌 Stay Updated: Subscribe to our newsletter for the latest tech news, tutorials, and exclusive offers! Signup Now
Disclaimer: The code examples provided in this article are for illustration purposes and may not directly reflect your implementation or specific code structure. Always ensure to adapt the code to suit your project's requirements.