How to access array elements in a Django template?
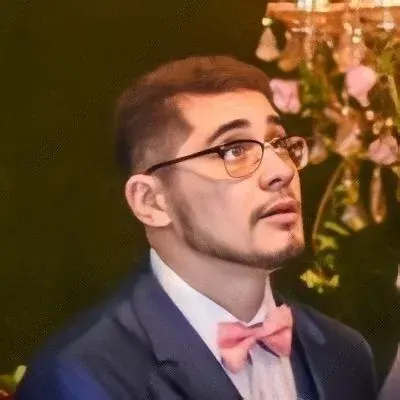
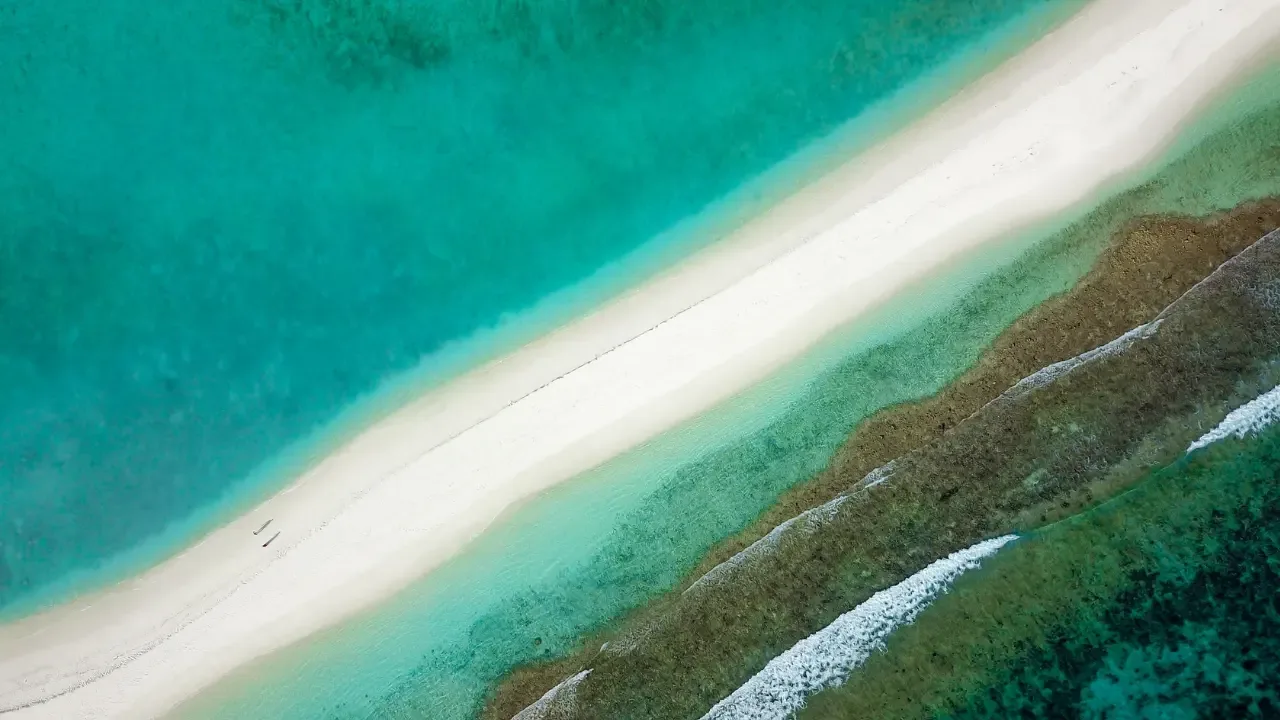
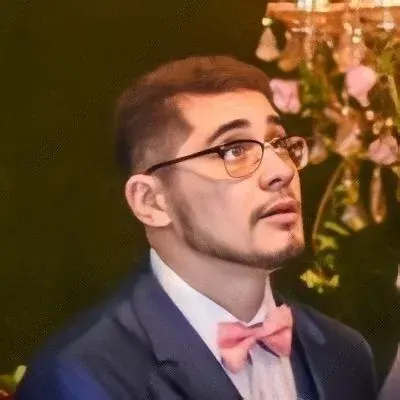
How to Access Array Elements in a Django Template? ππ
So, you have an array (arr
) that is passed to your Django template, and you want to access individual elements of the array without having to loop through the entire array. You're in luck! Django provides an easy solution for this. Let's dive right into it! πͺπΌπ₯
The Problem: Accessing Individual Array Elements in a Django Template
By default, Django templates are designed to work with HTML templates and provide a way to easily render dynamic data. However, when it comes to accessing individual elements of an array, Django templates don't offer a built-in syntax like arr[0]
or arr[1]
to directly access array elements.
The Solution: Using the forloop.counter
Variable
Fear not! Django provides an important variable called forloop.counter
that can be used to solve this problem. π‘
By leveraging the forloop.counter
variable, we can mimic array element access in a Django template. Here's how:
<!-- Accessing Individual Array Elements in Django Template -->
{% for item in arr %}
{% if forloop.counter0 == 0 %}
<!-- Accessing first element of the array: -->
{{ item }}
{% elif forloop.counter0 == 1 %}
<!-- Accessing second element of the array: -->
{{ item }}
{% endif %}
{% endfor %}
In the above code snippet, we are using forloop.counter0
instead of forloop.counter
to access array elements, as the forloop.counter
variable starts from 1. However, arrays are zero-indexed, so we need to subtract 1 from forloop.counter
to match the correct index.
π Note: Replace {{ item }}
with your desired code or rendering logic to make use of the individual array element.
Example: Accessing First and Second Elements of an Array in a Django Template
To illustrate this solution, let's assume we want to display the first and second elements of an array, arr
, in our Django template.
# views.py
from django.shortcuts import render
def my_view(request):
arr = ['element1', 'element2', 'element3']
return render(request, 'my_template.html', {'arr': arr})
<!-- my_template.html -->
<h1>Accessing First and Second Elements of an Array:</h1>
{% for item in arr %}
{% if forloop.counter0 == 0 %}
<p>First Element: {{ item }}</p>
{% elif forloop.counter0 == 1 %}
<p>Second Element: {{ item }}</p>
{% endif %}
{% endfor %}
In the above code snippet, we are accessing the first element of the arr
array using if forloop.counter0 == 0
, and the second element using if forloop.counter0 == 1
.
π‘ Feel free to modify the example to fit your requirements and extend it to access more array elements as needed.
Your Turn: Start Accessing Array Elements in Django Templates! π
Now that you know how to access individual array elements in a Django template, go ahead and implement it in your project. Experiment with different array elements and extend the solution to access more elements.
If you have any questions or run into any issues, feel free to leave a comment below. Happy coding! πβ¨
π [Action Time]: Share your Django template array element access experience in the comments section and let us know how this solution worked for you. We'd love to hear your success stories and any tips/tricks you discovered along the way. Let's grow and learn together! ππ