How do you serialize a model instance in Django?
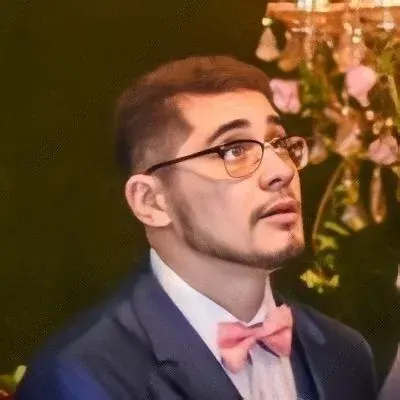
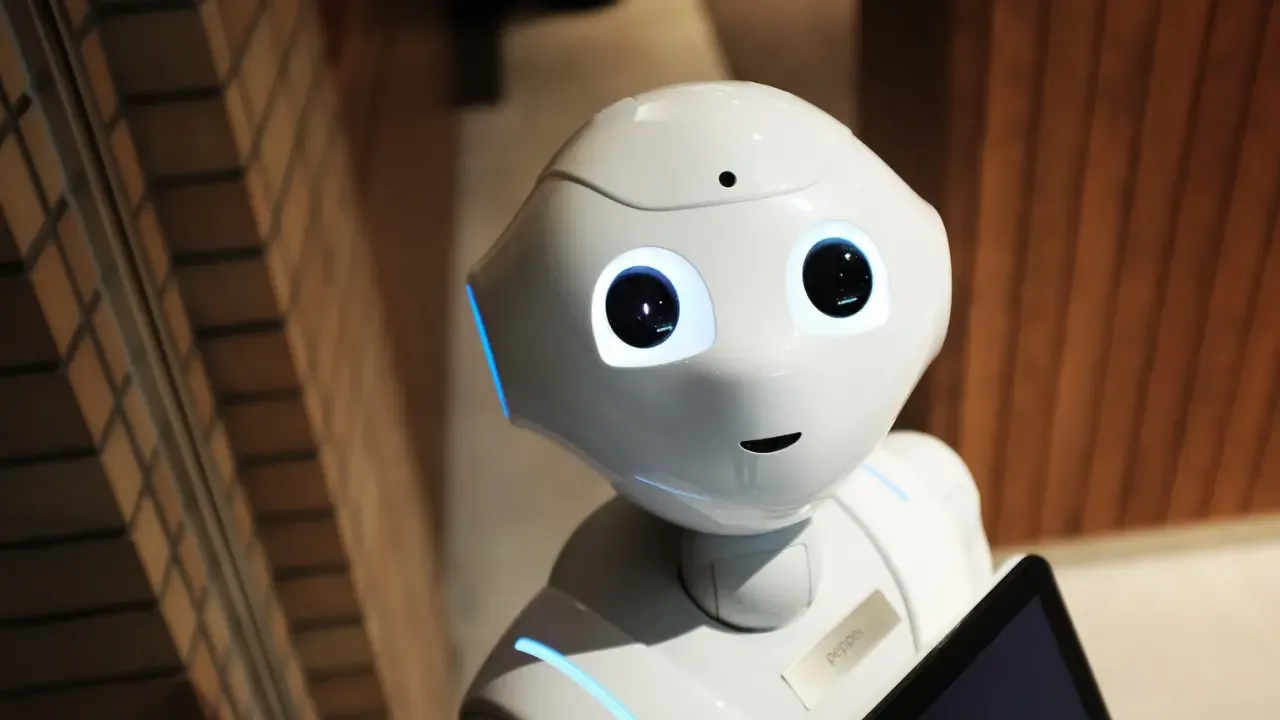
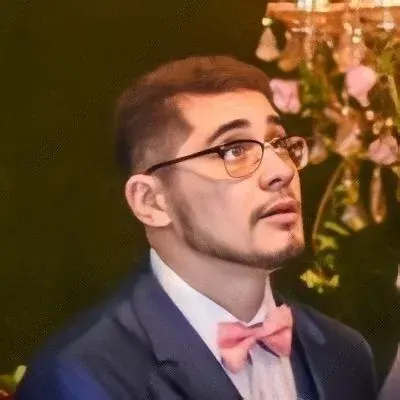
How to Serialize a Model Instance in Django: A Quick Guide 🚀
So, you've built your amazing Django application and now you need to serialize a single Model Instance to JSON. 🤔 Don't worry, we've got you covered! In this guide, we'll walk you through the process step by step. Let's dive right in!
The Challenge 🎯
By default, Django provides easy ways to serialize Model QuerySets. However, when it comes to serializing individual Model Instances, the documentation is a bit scarce. 😕 Hence, the question arises: How do we serialize only the fields of a Model Instance into JSON?
The Solution 💡
Fortunately, serializing a Model Instance in Django is simpler than you might think! Follow these three easy steps:
Step 1: Import the Necessary Libraries 📚
Start by importing the required libraries at the top of your Python file:
import json
from django.core.serializers import serialize
Step 2: Serialize the Model Instance 🔄
Next, let's serialize the Model Instance. Django's serialize
function provides a quick and convenient way to achieve this. Here's an example:
from myapp.models import MyModel
instance = MyModel.objects.get(id=1)
serialized_data = serialize('json', [instance], fields=('field1', 'field2', 'field3'))
In the above code snippet, we first retrieve the desired Model Instance using Django's ORM. Then, we pass the instance into the serialize
function, specifying the format ('json'
) and the desired fields to include in the serialization.
Step 3: Convert the Serialized Data to JSON 📜
Lastly, we need to convert the serialized data into a JSON string for easy consumption. We can achieve this using Python's built-in json
library:
json_data = json.loads(serialized_data)[0]['fields']
In the code snippet above, we utilize json.loads
to parse the serialized data into a Python dictionary. Finally, we extract the 'fields'
key from the dictionary, which contains the serialized Model Instance as JSON.
And there you have it! The fields of your Model Instance are now serialized as JSON. 🎉
Common Issues and Troubleshooting 🚧
Problem: Including Related Fields
What if your Model Instance has related fields? 🤔 By default, the serialize
function only serializes the fields of the Model Instance itself, excluding related fields.
To include related fields in the serialization, you can make use of Django's select_related
method:
instance = MyModel.objects.select_related('related_model').get(id=1)
By invoking select_related
with the desired related model(s) before retrieving the instance, Django will eagerly fetch the related fields, ensuring they are included in the serialization.
Problem: Customizing Serialization
Sometimes, you may want to customize the serialization process. For example, you might want to exclude certain fields or change their formatting.
To achieve this, you can define a custom Serializer class in your Django app and override its serialization methods as needed. This approach gives you fine-grained control over how your model instances are serialized.
For more information about custom serialization, refer to Django's documentation on Serializing Django Objects.
Go Ahead, Serialize with Confidence! 🙌
You're now armed with the knowledge to serialize a Model Instance in Django! 🚀 Whether you're building RESTful APIs or working with complex data structures, this skill will come in handy.
Remember, you can always refer back to this guide if you face any difficulties or need a quick reminder. Happy coding! 💻
Have any questions or encountered any hurdles while serializing your Model Instances? We'd love to hear from you! Leave a comment below and let's tackle these challenges together. 👇