How do I reference a Django settings variable in my models.py?
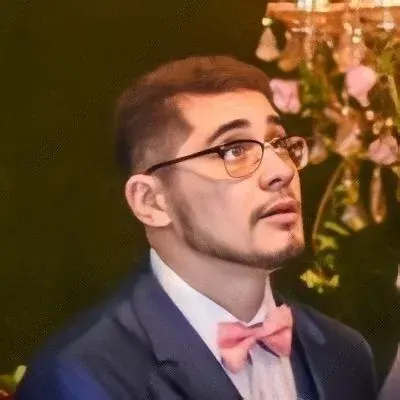
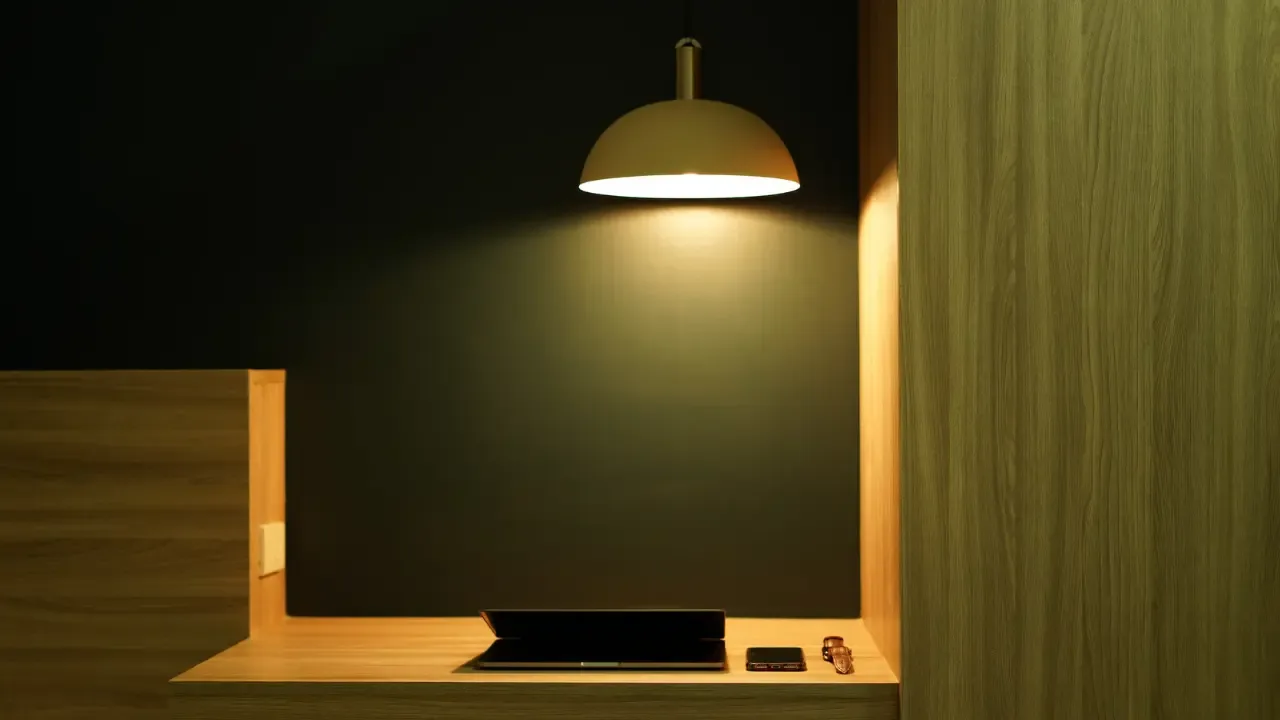
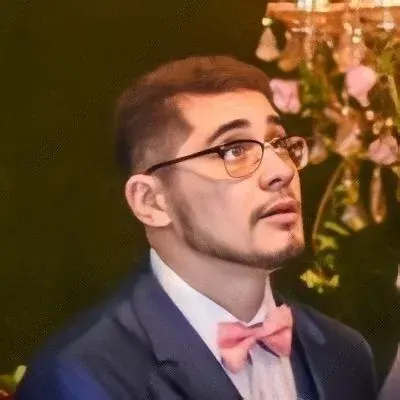
How to Reference a Django Settings Variable in your models.py
So, you're building a Django project and you want to reference a variable from your settings.py file in your models.py file. You may have tried a few things already, like settings.PRIVATE_DIR
, but ended up with a frustrating NameError: name 'PRIVATE_DIR' is not defined
. Don't worry, we've got you covered! 🤩
The Problem
Let's first understand why you're facing this issue. The error message indicates that the PRIVATE_DIR
variable is not defined, which means it's not accessible in your models.py file. But fear not, there's a simple solution to this problem! 😎
The Solution
To reference a Django settings variable in your models.py, you should import the settings
module and access the variable using settings.VARIABLE_NAME
. In your case, it would be from django.conf import settings
and settings.PRIVATE_DIR
. Let's update your code snippet accordingly:
from django.conf import settings
from django.core.files.storage import FileSystemStorage
fs = FileSystemStorage(location=settings.PRIVATE_DIR)
class Customer(models.Model):
lastName = models.CharField(max_length=20)
firstName = models.CharField(max_length=20)
image = models.ImageField(storage=fs, upload_to='photos', blank=True, null=True)
With this modification, you should no longer encounter the NameError
. Your models.py file will now be able to access the PRIVATE_DIR
variable from the settings.py file successfully. 🎉
Handy Tip
Remember to ensure that the PRIVATE_DIR
variable is properly defined and assigned in your settings.py file. It should be located at the root level of the file, outside of any function or class definition, similar to the provided code snippet:
PRIVATE_DIR = '/home/me/django_projects/myproject/storage_dir'
Share your Experience
We hope this guide helped you resolve the issue of referencing a Django settings variable in your models.py file. Let us know in the comments below if you found this solution helpful or if you have any other Django-related questions. Keep calm and code on! 💻💪
👉 What other Django problems have you faced? Share your experience in the comments! 👈