How do I raise a Response Forbidden in django
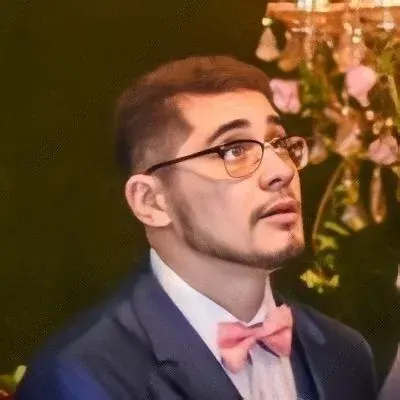
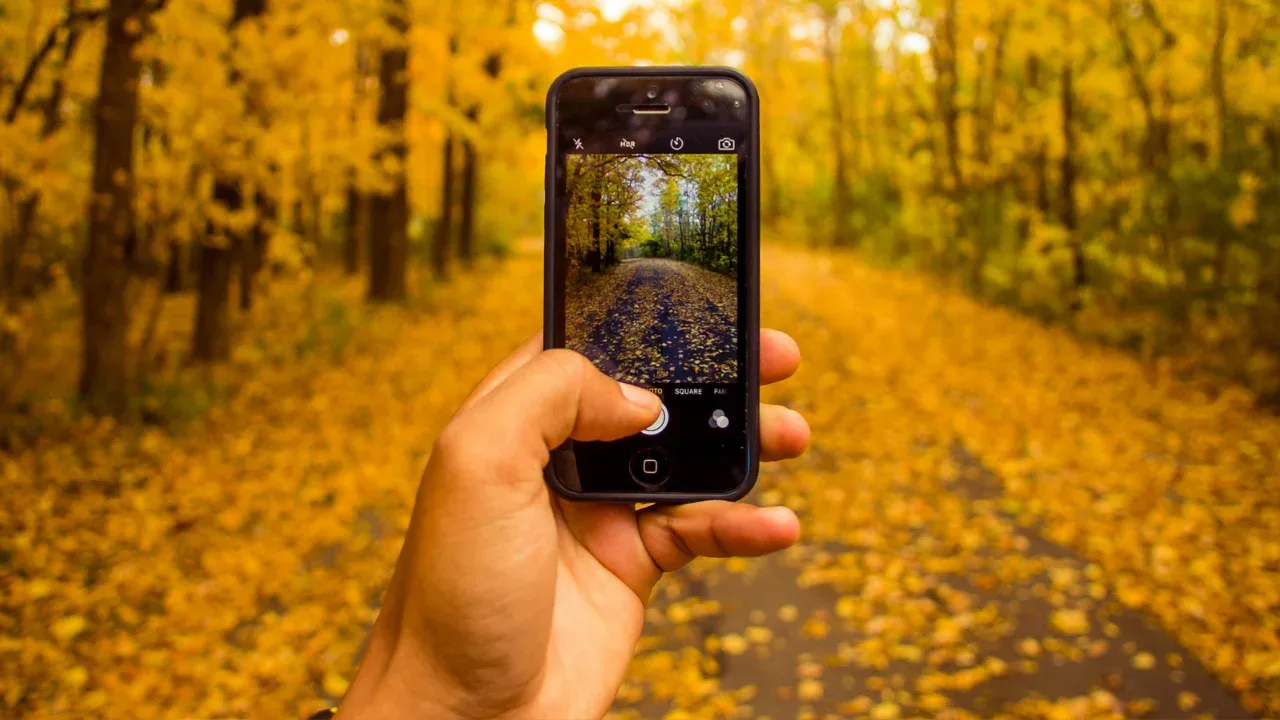
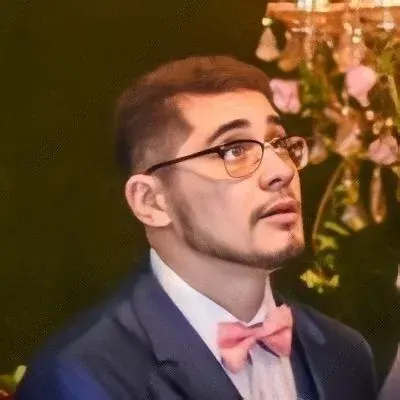
š Title: How to Raise a "Response Forbidden" in Django: Easy Solutions to a Common Error!
Introduction
š Hey there, Django developers! š©āš»šØāš» Are you struggling with raising a "Response Forbidden" error in Django? It can be quite frustrating when you encounter error messages like "exceptions must be old-style classes or derived from BaseException, not HttpResponseForbidden". But don't worry, I've got you covered! In this blog post, I'll guide you through common issues when raising a "Response Forbidden" error and provide you with easy solutions to resolve them. Let's dive in! š
Understanding the Problem
So, you want to raise a "Response Forbidden" error in Django by using the HttpResponseForbidden()
function. However, you're encountering the following error:
exceptions must be old-style classes or derived from BaseException, not HttpResponseForbidden
This error occurs because HttpResponseForbidden()
is a class-based exception, and in modern Python, exceptions must be derived from the BaseException class. Luckily, there's a simple solution to overcome this issue! šŖ
Easy Solution: Raising a "Response Forbidden" Error
To raise a "Response Forbidden" error in Django, you can follow these steps:
Import the
HttpResponseForbidden
class from Django'shttp
module:
from django.http import HttpResponseForbidden
Raise the exception using the class directly:
raise HttpResponseForbidden()
That's it! With these two steps, you can raise a "Response Forbidden" error without any issues. š
Deeper Dive: Understanding Old-Style Classes
Now, you might wonder, what are "old-style classes" mentioned in the error message? In Python 2.x, classes that didn't inherit from object
were considered old-style classes. However, in Python 3.x, everything is an object, so the concept of old-style classes has been removed.
The error message you encountered is a Python 3.x error, suggesting that you need to use a class derived from BaseException
instead of a class-based exception. By importing HttpResponseForbidden
from Django's http
module, you're using the appropriate class derived from BaseException
. šÆ
Conclusion and Call-to-Action
In conclusion, raising a "Response Forbidden" error in Django is straightforward when you follow the correct approach. Remember to use the HttpResponseForbidden
class from Django's http
module, and you'll be able to raise the error without any hiccups. š
If you found this blog post helpful, why not share it with your fellow Django developers? Spread the knowledge! š
Feel free to leave a comment below if you have any questions or need further assistance. I'm here to help! Happy coding, folks! š©āš»šØāš»
š Like this article? Share it with your Django friends! š