How do I make many-to-many field optional in Django?
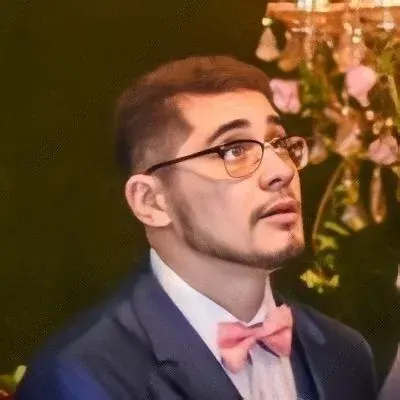
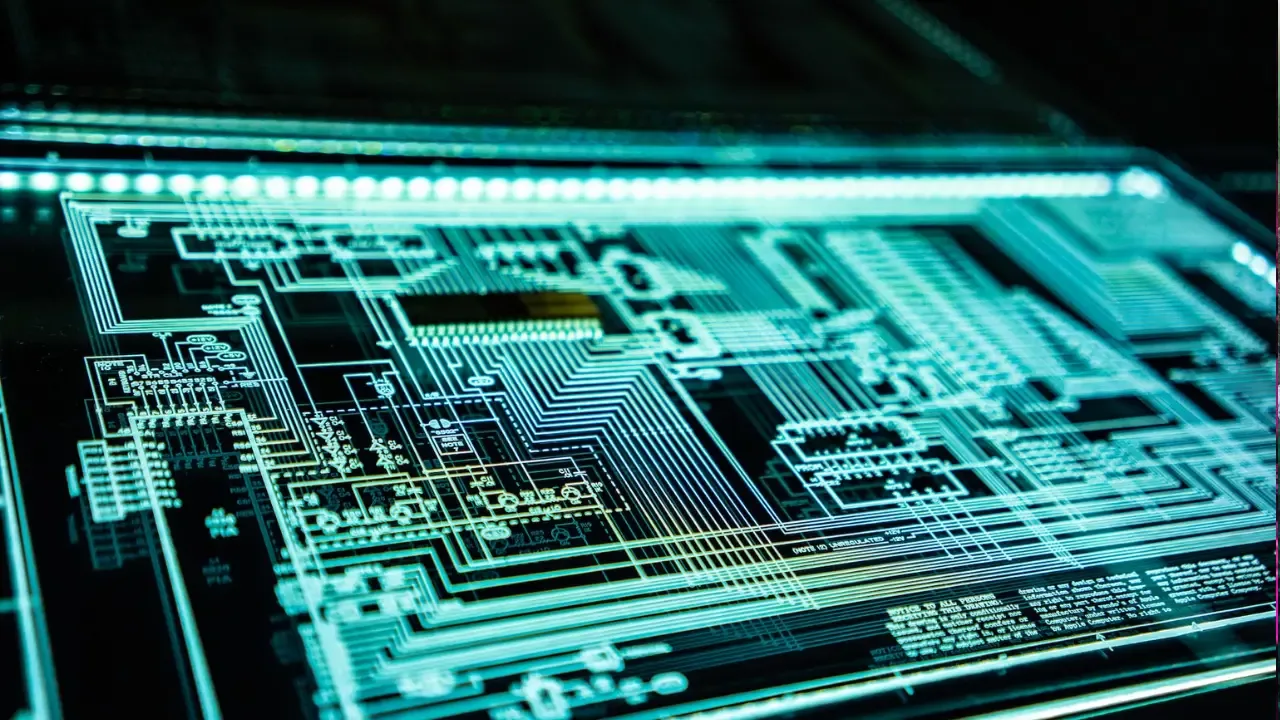
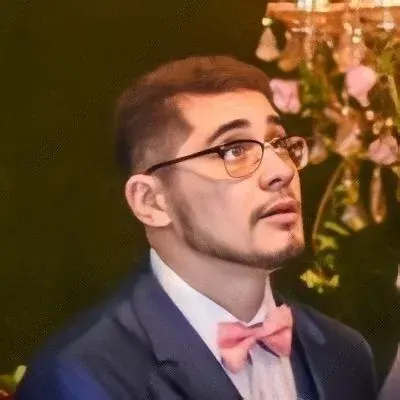
How to Make a Many-to-Many Field Optional in Django 😊
So you're working on a Django app and you've encountered a common issue with many-to-many relationships. When using the admin interface, you're required to enter a value for the many-to-many field, even though it doesn't have to exist for you to create the first entry. 🤔
In this blog post, we'll explore this issue in detail and provide easy solutions to make the many-to-many field optional. Let's dive right in! 💪
The Challenge: Creating an Event Organizer App 🎉
To provide some context, let's imagine we have two models: Event
and Group
. These models are connected through a many-to-many relationship. In the Django admin interface, you're supposed to add a Group
while creating an Event
. However, it would be great if we didn't have to make this relationship mandatory. 🙌
The Power of related_name
in Django 👥
In Django, the related_name
parameter allows you to specify the name of the reverse relation from the target model back to the current model. By default, Django creates an extra table with indices of the two related models. But here's the catch – we don't really need to populate this extra table just to create an Event
. 🙅♂️
Solution: Making the Many-to-Many Field Optional ✔️
To make the many-to-many field optional in Django, we can follow these steps:
Open the file containing your
Event
model (e.g.,models.py
) in your Django project.Locate the many-to-many field representing the relationship with the
Group
model.Add the
blank=True
parameter to the field definition. This allows the field to be left empty when creating anEvent
.Save the file and run the migrations using the
python manage.py makemigrations
andpython manage.py migrate
commands.
from django.db import models
class Event(models.Model):
# Your other fields
groups = models.ManyToManyField('Group', blank=True)
That's it! With just a simple addition of blank=True
, you've made the many-to-many field optional. Now, you can create an Event
without being forced to add a Group
. 🎉
Embracing Flexibility with the Django Admin Interface 🖥️
But wait, there's more! How can we make the Django admin interface realize that the many-to-many field is now optional? Good news – Django provides a way to customize the admin interface easily. Let's do it! ✨
Open the file containing your
Event
admin configuration (e.g.,admin.py
) in your Django project.Import the necessary modules:
from django.contrib import admin
from .models import Event
Create a class to represent your
Event
admin configuration:class EventAdmin(admin.ModelAdmin):
Inside the class, define the
filter_horizontal
attribute and set it to the name of the many-to-many field:filter_horizontal = ('groups',)
Register your
Event
model with the custom admin configuration:admin.site.register(Event, EventAdmin)
Here's an example of how your Event
admin configuration might look:
from django.contrib import admin
from .models import Event
class EventAdmin(admin.ModelAdmin):
filter_horizontal = ('groups',)
admin.site.register(Event, EventAdmin)
By using the filter_horizontal
attribute, you can achieve a more user-friendly and intuitive interface for selecting Group
values when creating an Event
. 🎉
Time to Take Control of Your Many-to-Many Fields! ✊
Congratulations, you've conquered the challenge of making many-to-many fields optional in Django! Now you have the power to create Event
entries without adding a Group
, thanks to the blank=True
parameter. 🚀
Don't forget to apply the custom admin configuration to enhance the user experience with the filter_horizontal
attribute. Your users will surely appreciate the flexibility you've provided. 😊
If you have any questions or want to share your experience, feel free to leave a comment below. Happy coding, and remember – there's no limit to what you can achieve with Django! 💪⚡️