How do I do an OR filter in a Django query?
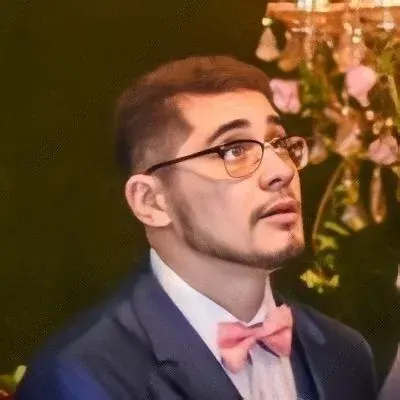
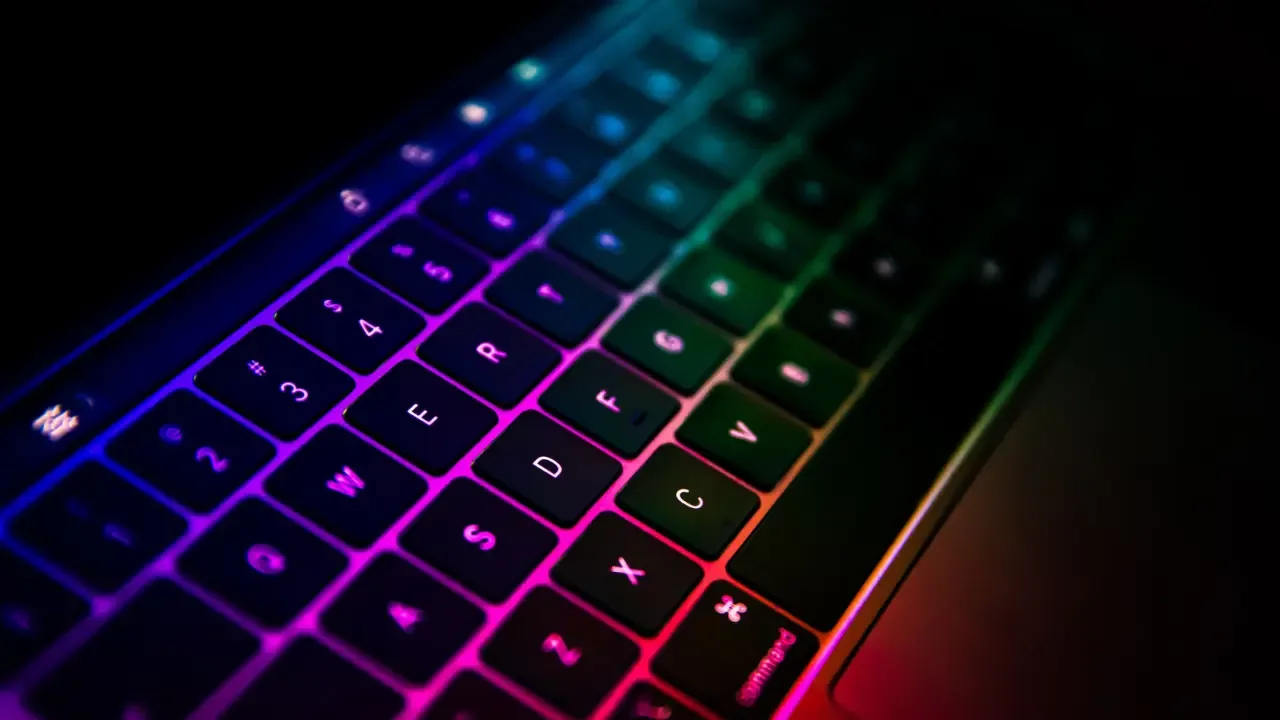
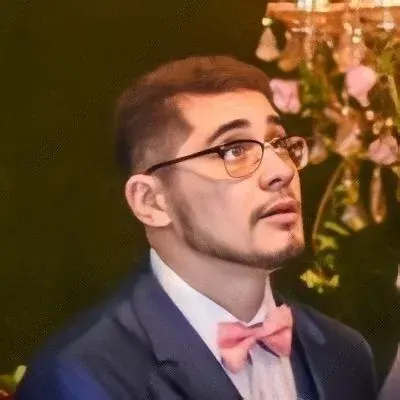
š Tech Blog Post: How to Use OR Filtering in Django Queries
š Welcome, Django Developers! š
Are you facing the challenge of filtering your Django query to retrieve items that have either been added by a specific user or have not been moderated yet? Fear not, for we have the perfect solution for you! š
š Understanding the Problem The goal is to select items that satisfy the following condition: either the item was created by the owner or the item has not been moderated. Let's break this down:
Item creator = ownerOR Item moderation status = False
š” The Django QuerySet API to the Rescue!
Django provides a powerful QuerySet API that allows us to filter and query the database in a convenient and efficient way. To achieve our desired filtering, we can use the Q
object in combination with the |
operator, which represents the OR condition in a Django query.
⨠The Solution: OR Filtering in Django Queries
Here's how you can write your Django query using the Q
object and the |
operator:
from django.db.models import Q
items = YourModel.objects.filter(Q(creator=owner) | Q(moderated=False))
š„ Explanation:
Q(creator=owner)
represents the condition "item creator is the owner". This filters the items that the owner has added.Q(moderated=False)
represents the condition "item moderation status is False". This filters the items that have not been moderated yet.By using the
|
operator between the two conditions, we combine them with the OR condition, ensuring that either condition is met for an item to be included in the result set.
š Supercharge Your Django Query!
But wait, there's more! Django's QuerySet API offers a wide range of lookups and query expressions to further refine your filtering. You can add additional conditions or combine them with other logical operators, such as AND (&
), to create complex queries.
š” Pro Tip: QuerySet Laziness
Remember that Django QuerySets are lazy, meaning that the actual database query is not executed until the result is evaluated or iterated over. Therefore, you can further chain methods like order_by()
, values()
, or count()
to enhance your query.
š Your Turn! Now that you've learned how to use OR filtering in Django queries, it's time to put your newfound knowledge into practice! Modify your Django queryset, and see those desired items being fetched effortlessly.
š¬ We'd love to hear about your experience! Share your thoughts, challenges, or success stories in the comments below. Let's tackle this Django challenge together!
š¢ Spread the Word! If you found this blog post helpful, consider sharing it with your fellow Django developers. After all, sharing is caring! š
Happy Django coding! šāØ